Arrays in Java
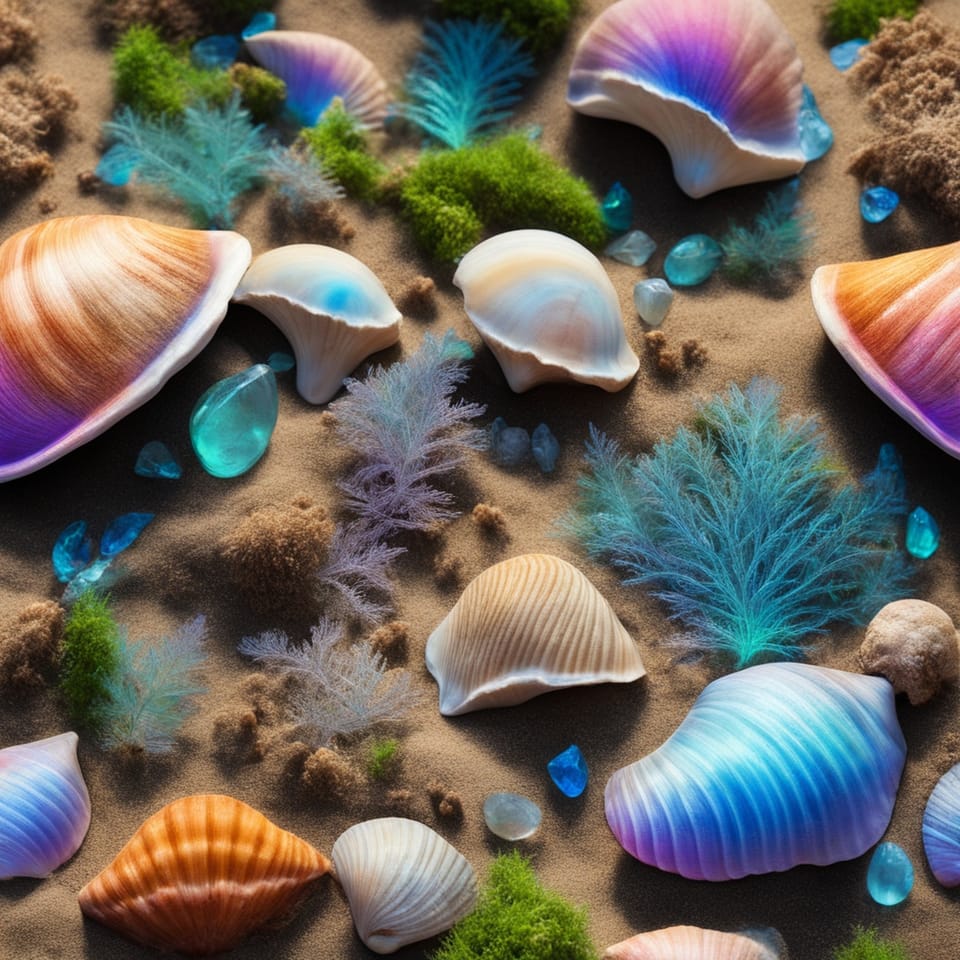
What Are Arrays?
Arrays are a special type of container object. They contain a fixed number of objects that are the same type. Each value in an array is called an element. Arrays extend Object, so they have the same methods any object does. Arrays are commonly used for things like handling binary data or building collections.
Declaring and Initializing Arrays
Arrays are declared starting with a type and ending with open and close brackets.
final int[] numbers;
final boolean[] flags;
final Object[] objects;
To initialize an array, you can do it with the following:
final int[] numbers = new int[10];
This declares and initializes an array with 10 elements. When an array is created, each element is assigned a default value. The default value of each element will depend on the type that the array is. These default values are the same default values assigned to non-array variables when they are declared and not assigned a value.
Type | Default Value |
---|---|
boolean | false |
char | '\u0000' which represents the null character |
byte | 0 |
short | 0 |
int | 0 |
long | 0 |
float | 0.0 |
double | 0.0 |
Any Object | null |
When declaring an array, you can also assign all the values to it at the same time with the following:
final var numbers = {1, 2, 3};
This declared an array with 3 elements, and each element assigned a value.
Accessing Elements
The elements in an array are accessed using an index. An array's index is zero based, meaning you start counting at 0 instead of 1. You can access elements in an array with the following:
final int[] numbers = {2, 4, 6};
System.out.println(numbers[0]);
System.out.println(numbers[1]);
System.out.println(numbers[2]);
This will print the following to the console:
2
4
6
If you try to access an element that doesn't exist, an java.lang.ArrayIndexOutOfBoundsException will be thrown. In the above example, if you were to try to access the element at index 3, this exception would be thrown since 2 is the last index.
Modifying Arrays
The elements of an array can be modified using the index and then assigning a new value to it.
final int[] numbers = {1, 2, 3};
numbers[0] = 2;
numbers[1] = 4;
numbers[2] = 6;
After this code executes, the new elements in the array would be [2, 4, 6]. Even though the array is marked as final, this doesn't mean that the elements cannot be reassigned a value. This only means that the numbers variable cannot be reassigned.
Array Length
Arrays have a length property. This returns how many elements the array has and will always be the length that the array was defined as.
final int[] numbers = new int[5];
System.out.println(numbers.length);
This will print 5 to the console. An array's length is not zero based since it isn't an index and is instead the length of the array.
Using the length property, you can get the last element in an array with the following:
final int[] numbers = new int[5];
final int lastElement = numbers[numbers.length - 1];
Remember, indexes are zero-based, so in order to get the last element, you have to subtract 1 from the length.
Iterating Over an Array
You can iterate over an array using loops in one of two ways. The legacy way is to use a for loop and access each element by the index.
final int[] number = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println(number[i]);
}
The modern way of doing it is to use a foreach loop.
final int[] numbers = {1, 2, 3, 4, 5};
for (final int element : numbers) {
System.out.println(element);
}
Both of these would print each element of the array.
Multidimensional Arrays
Arrays can be defined as multidimensional arrays. These are arrays of arrays. Each array can be its own size. The syntax for defining a multidimensional array is the following:
final int[][] numbers = {
{1, 2, 3, 4, 5},
{2, 4, 6, 8},
{3, 6, 9}
};
Each element in a multidimensional array is another array.
final int[] even = numbers[1];
This would return the array with the elements of [2, 4, 6, 8]. You can access elements in a multidimensional array with the following:
final int number = numbers[0][4];
This first accesses the array at the 0 index and then accesses the element of that array at index 4. This would return the number 5.
Transforming Arrays Into a List
It is common to need to transform an array into a List. You can do this manually, but it is so common that there is actually a method you can use in the java.util.Arrays class. The Arrays class has several helper methods when working with arrays. The asList() method will convert an array to a List.
final int[] array = {1, 2, 3};
final List<Integer> list = Arrays.asList(array);
You can also convert an array to a stream using the following:
final int[] array = {1, 2, 3};
final Stream<Integer> stream = Arrays.stream(array);
If you are interested in what other helper methods the Arrays class offers, you can view the Javadoc.
Conclusion
Arrays are a special object that is a container for a fixed number of values. Elements are accessed using a zero-based index. When working with arrays, the Arrays class has lots of helper methods that are good to get familiar with.