Avoiding Redundant Method and Variable Names
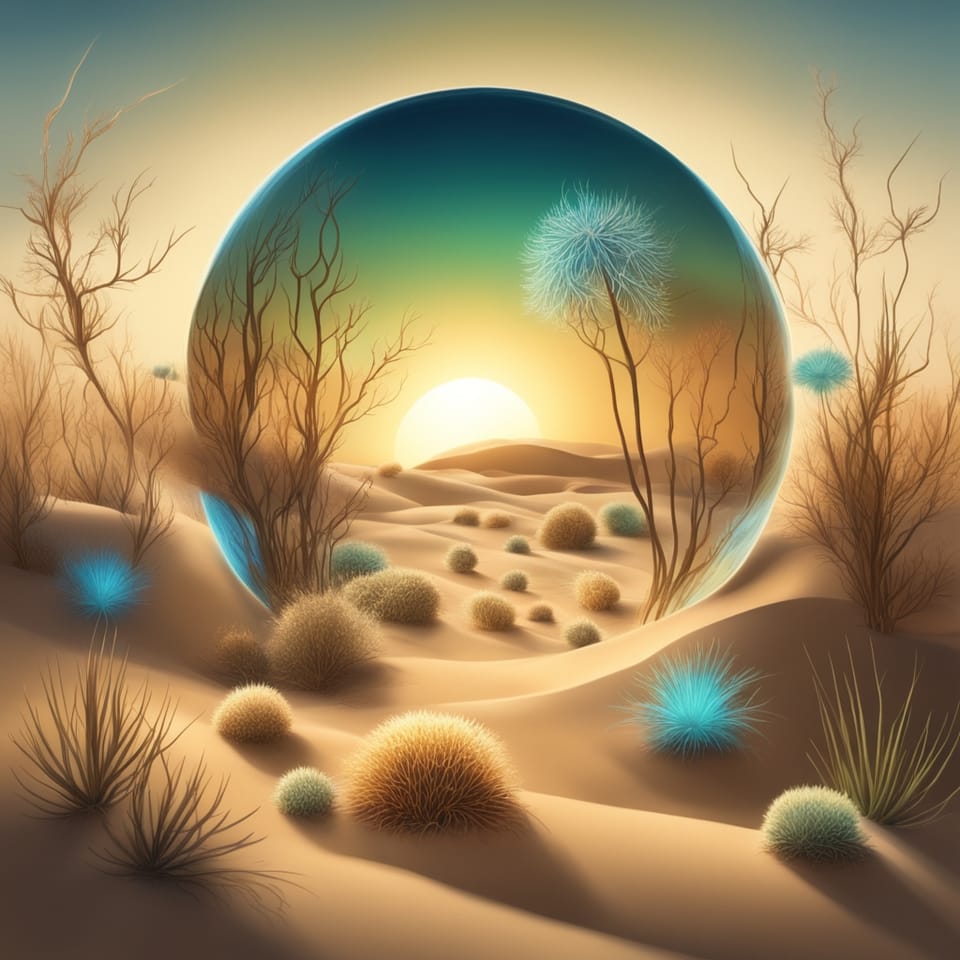
Context and Redundant Naming
Redundant names are names that have a detail that is already provided in the code. Redundant names can be avoided by understanding the context of where the name occurs in code. Context changes how a variable is named. What would be a good name in one place may be redundant in another.
Redundant Variable Names
Having the type in a variable name is usually unnecessary, regardless of context. Here are some examples:
// Java code
final var nameString = "name";
final var accountToAuthenticate = new Account();
final var timeoutInt = 10;
final var ordersList = new ArrayList<Order>();
Each of these variable names contains the type and is redundant. These names should have the type removed from them.
// Java code
final var name = "name";
final var toAuthenticate = new Account();
final var timeout = 10;
final var orders = new ArrayList<Order>();
Redundant Method Names
Redundant method names occur depending on the context of the class name, the method parameters, or the method return type.
Context of Classes
The following method name is redundant because it is already in a class named User.
// Java code
public class User {
public void preSaveUser() {
// ...
}
}
This method should have User removed from it.
// Java code
public class User {
public void preSave() {
// ...
}
}
Context of Method Parameters
Redundant method names are more common in methods that take an object.
// Java code
public boolean authenticateUser(final User user) {
// ..
}
This method takes a User as a parameter. Adding User to the method name is redundant and should be removed.
// Java code
public class User {
public boolean authenticate(final User user) {
// ..
}
}
Context of Method Return Type
A method that has a return type already states what it returns.
// Java code
public Order createOrder() {
return new Order();
}
Order is redundant and should be removed from the name.
// Java code
public Order create() {
return new Order();
}
Other Words That Are Redundant
When dealing with methods that return a value, don't use the word "get" or similar words in the method name.
// Java code
public Address getAddress() {
return address;
}
Using the word get is redundant because it is returning a value. This can be simplified to the following:
// Java code
public Address address() {
return address;
}
What Are the Exceptions?
Adding the type to a method name that converts from one type to another can add clarity to the code.
// Java code
public class User {
@Override
public LiteUser toLiteUser() {
// ...
}
}
Adding the type to a method name might be required if the name clashes with another name. In the Java programming language, the following is not allowed.
// Java code
public class MyClass {
public String value() {
// ...
}
public int value() {
// ...
}
}
The two value() method names clash and won't compile in Java. In this case, you would have to have the type in the name.
Conclusion
Eliminating redundant naming mostly comes from not using the type in the name. Consider the context of the code to determine if the name is redundant or not.