Being Positive When Writing if Statements
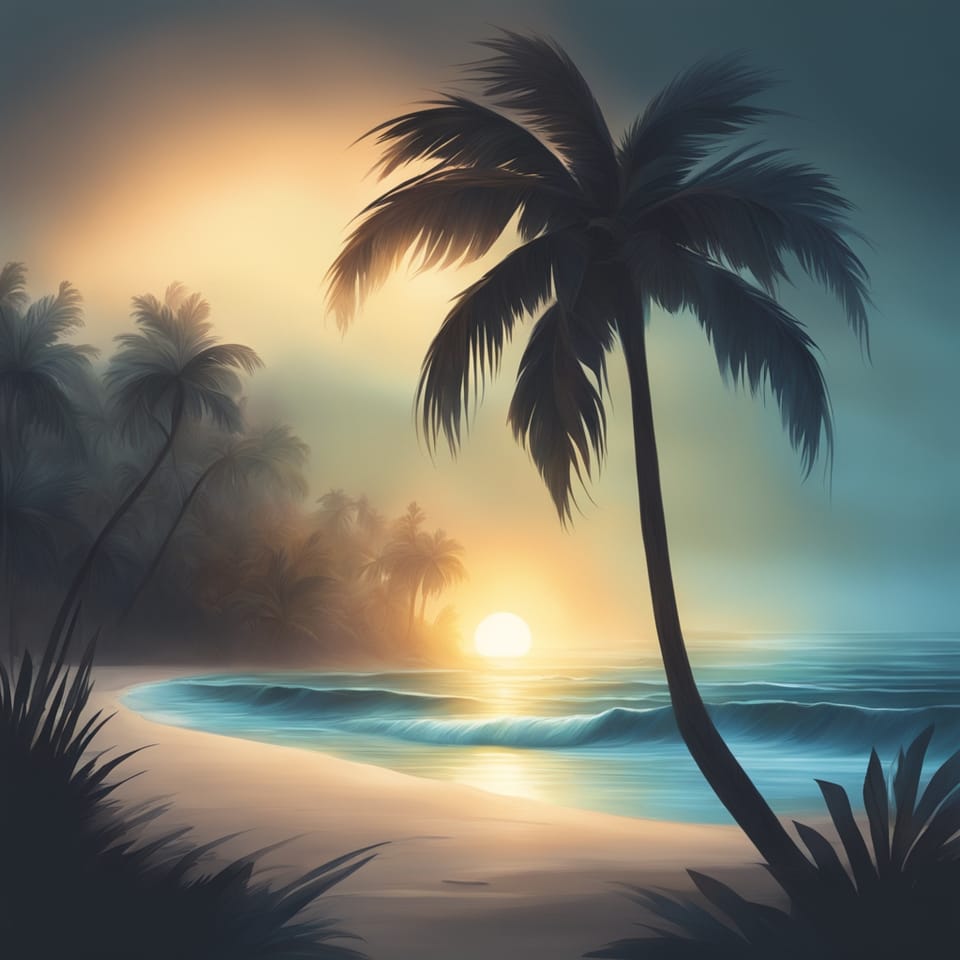
Being Negative
For whatever reason, when writing logic, the negative comes to mind first, so this is what is written first. An example of writing the negative first is the following:
if (a != b) {
return "Opened";
} else {
return "Closed";
}
Although this may be what you want to write, when it comes to writing it, it takes longer to understand.
Being Positive
When writing if statements, write the positive condition first. It tells the developer what the intent is instead of what the intent isn't. The previous example should be written like the following:
if (a == b) {
return "Closed";
} else {
return "Opened";
}
Positive conditions are more natural and easier to understand.
Positive Concepts
When dealing with boolean values, not just if statements, write them positive, not negative. In the first example, developers will generally want to write the first example. This is because it hasn't been translated into a concept yet. When translated into a concept, the code will be written how most developers normally want to write it. Using the first example in this article, which used the negative first, you can see how it becomes positive once the concept is created. Also, the poor variable names have been renamed.
final var isStoreOpened =
dayOfTheWeek != sunday;
if (isStoreOpened) {
return "Opened";
} else {
return "Closed";
}
This both satisfies how developers want to write it as well as making the code easier to read.
Positive Functions and Methods
This same concept applies when writing functions or methods that return a boolean. If you were writing your own collection class that needs an isEmpty() method, do you also need an isNotEmpty() method? Never write both the positive and negative for a method. You only want to create the positive.
// Possible use case 1
final var greetNewCustomer = orders.isEmpty();
// Possible use case 2
final var greetExistingCustomer = !orders.isEmpty();
You'll reduce the amount of work and keep it simple. Additionally, what happens if someone decides to write code like the following:
if (!items.isNotEmpty()) {
// ..
}
If you are always using the positive first, you'll eliminate most, if not all use cases for an isNotEmpty() method.
Conclusion
Writing negative logic does take longer to understand. Using the positive instead of the negative simplifies the logic and is quicker to understand.