Booleans and Boolean Operators in Swift
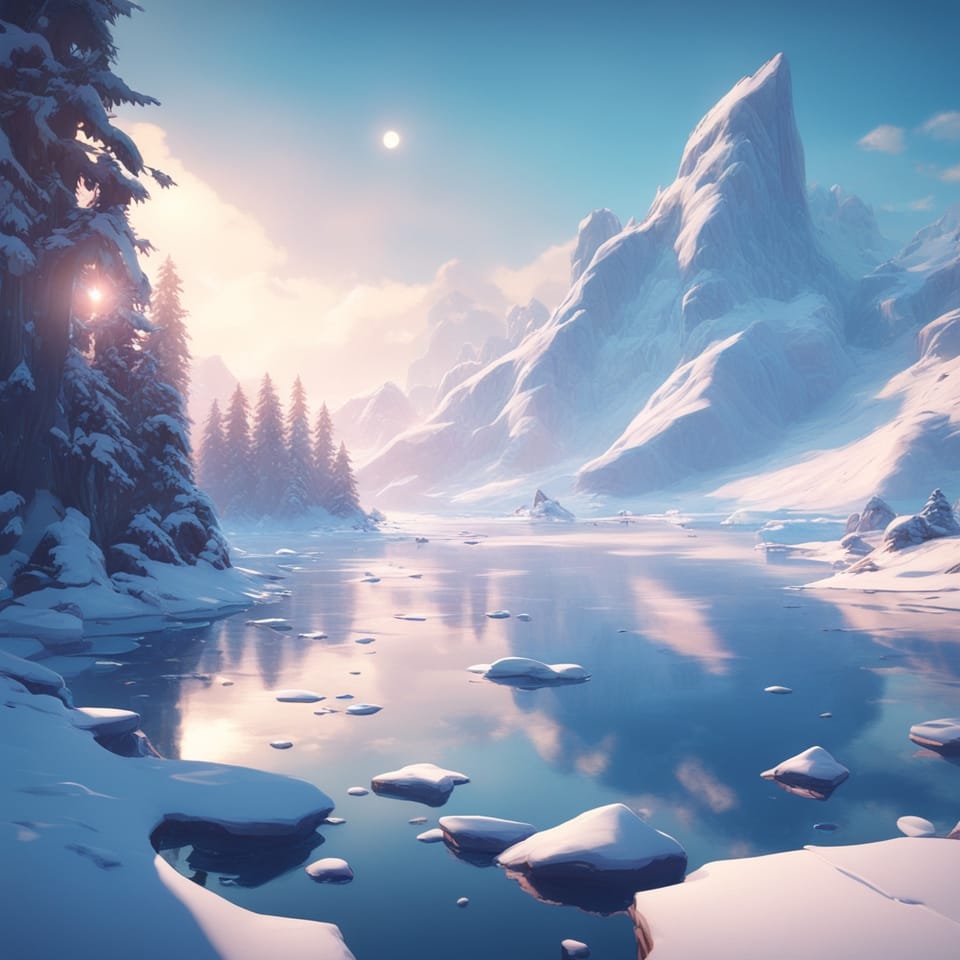
Booleans
Booleans, or Bools, in Swift are a value that represents either a true or false value. They are written with the following with or without the type annotations.
let isFalse = false
let isTrue: Bool = true
Boolean Operators
Boolean operators allow you to provide conditional logic and also reverse the boolean value.
Or Operator
The or operator is used to check to see if a condition or another condition is met. Using the or operator, only one condition needs to be true. Once a condition evaluates to true, it will not continue to execute any of the other conditions. An or operator is written using || and is shown in the following example:
let c = a || b
And Operator
The and operator is used to check to see if a condition and another condition are true. It will evaluate to true if both conditions are met. If a condition evaluates to false, it will stop evaluating any additional condition. An or operator is written using && and is shown in the following example:
let c = a && b
Not Operator
The not operator is used to reverse the value of a boolean. It is written using the ! character.
let isValid = true
let isNotValid = !isValid
This can also be used to reverse the result of a boolean expression.
Toggling Booleans
Booleans defined using the var keyword can be toggled one of two ways. The first is to use the not operator.
var myBool = true
myBool = !myBool // false
The second is to use the toggle() function.
var myBool = true
myBool.toggle() // false
Conclusion
Booleans are used for boolean logic. They can be both a variable value and the result of an expression.