Checked vs Unchecked Exceptions in Java
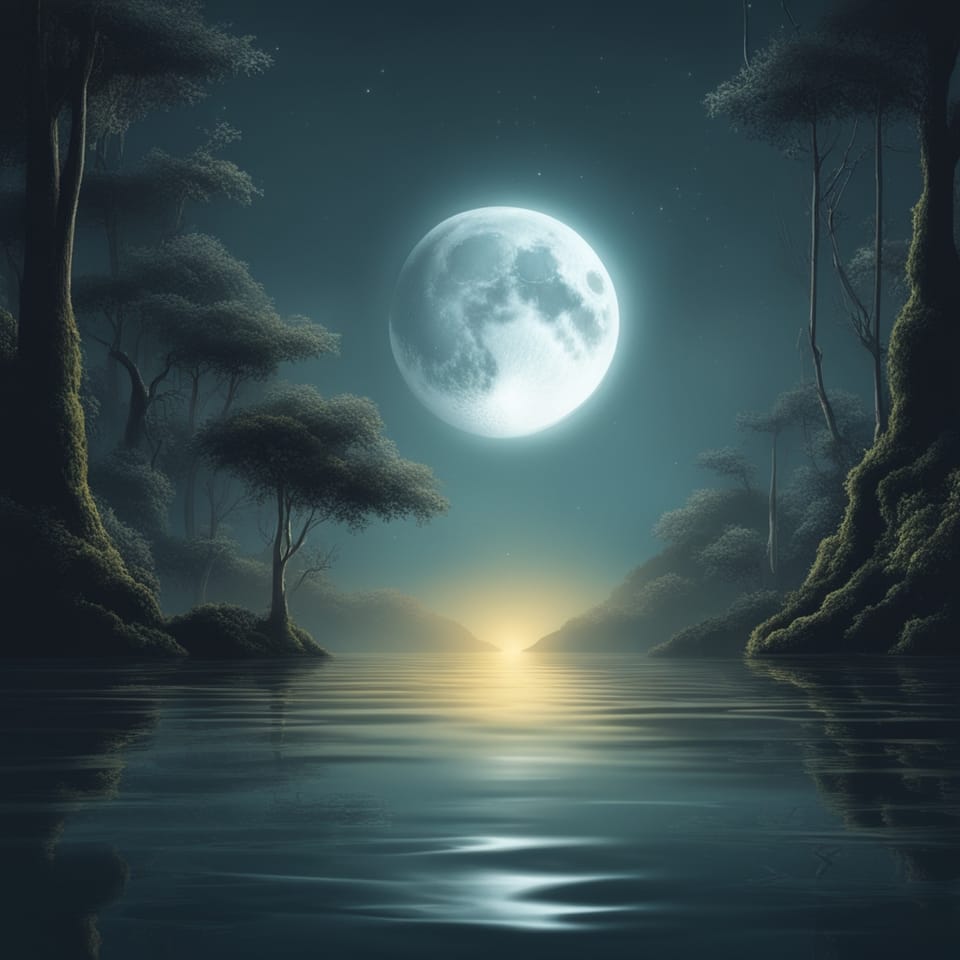
Unchecked Exceptions
If you are coming from a language that uses exceptions other than Java, unchecked exceptions are probably similar to what you are used to. They are exceptions that are thrown at runtime and not checked by the compiler. Some examples of this are NullPointerException and IndexOutOfBoundsException. Unchecked exceptions can be handled using a try block.
try {
methodThatThrowsException();
} catch (final Exception e) {
// handle exception
}
Unchecked exceptions extend the RuntimeException class. The following defines an unchecked exception:
public class NegativeNumberException extends RuntimeException {
// ...
}
Checked Exceptions
Checked exceptions are exceptions that are checked at compile time. The compiler ensures that they are either caught or declared in a method or constructor's throws clause. Examples of checked exceptions are IOException and FileNotFoundException. To handle a checked exception, you can do two things. The first is to handle it in a try block, the same as an unchecked exception. The second is to add the exception to the throws clause on the signature of a method or constructor. In the following example, the test() method throws a TestFailedException. This can be handled with a throws clause as follows:
public void doSomething() throws TestFailedException {
// This throws the checked exception
// TestFailedException somewhere in this method.
test();
}
By adding it to the throws clause, the compiler guarantees that each caller of the doSomething() method must handle the TestFailedException.
Checked exceptions extend the Exception class. The following defines a checked exception:
public class ConfigurationMissedException extends Exception {
// ...
}
When to Use One Over the Other
There is a lot of controversy over when to use one over the other. However, there are two recommendations worth noting. The Oracle Java documentation states the following for when to use them:
"If a client can reasonably be expected to recover from an exception, make it a checked exception. If a client cannot do anything to recover from the exception, make it an unchecked exception"
In Joshua Bloch's book, "Effective Java" it says:
"Use checked exceptions for recoverable conditions and runtime exceptions for programming errors" (Item 58 in 2nd edition)
These are both saying essentially the same thing but with different wording.
Conclusion
Checked exceptions are checked at compile time, while unchecked exceptions are not. Use checked exceptions when they can be recovered from and unchecked exceptions for programming errors.