Classes vs Objects
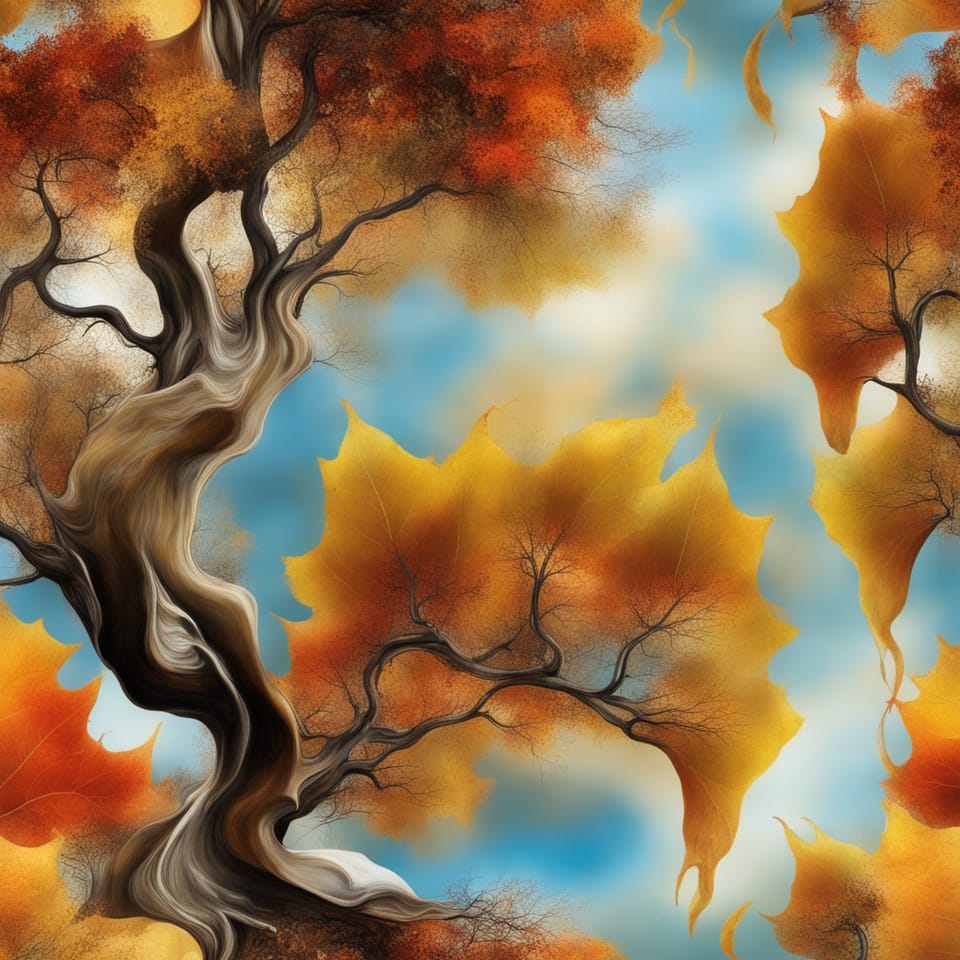
What Are Classes?
A class definition is a template for creating objects. A class defines variables, methods, constructors, etc. Each of these things also has an access modifier associated with it. Access modifiers control what is encapsulated in the class and what is publicly accessible. An example of a class in the Java programming language is the following:
// Java code
public class User {
// Variables
private long id;
private String name;
// Constructors
public User() {
}
// Methods
public long id() {
return id;
}
public void setId(final long id) {
this.id = id;
}
public String name() {
return name;
}
public void setName(final String name) {
this.name = name;
}
}
This defines a class called User that has variables, a constructor, and methods. A class by itself doesn't do anything without an object.
What Are Objects?
An object is an instance of a class and is also called an instance. Each object contains its own set of variables that are declared in a class.
Objects are created by instantiating a class. In the Java programming language, this is done using the new keyword.
// Java Code
final User user = new User();
This creates an object of the User class. When you create an object, you invoke the code in the constructor.
Once an object is created, you can access the methods and variables declared in that class if the access modifier allows it. In Java, you do this starting with the variable name, followed by a period, followed by what you are wanting to access.
// Java code
user.setId(1L);
user.setName("new user");
This calls the setId() and setName() methods on the user object. Both of these methods also modify the variables in the object.
// Java code
final long id = user.id(); // 1
final String name = user.name(); // new user
Calling the methods above after the two set methods have been called will result in the values shown in the example.
Conclusion
Classes are a way to define a template for creating objects. Objects are instances of a class. Each object has its own set of variables that are defined in the class.