Clean Method Overloading
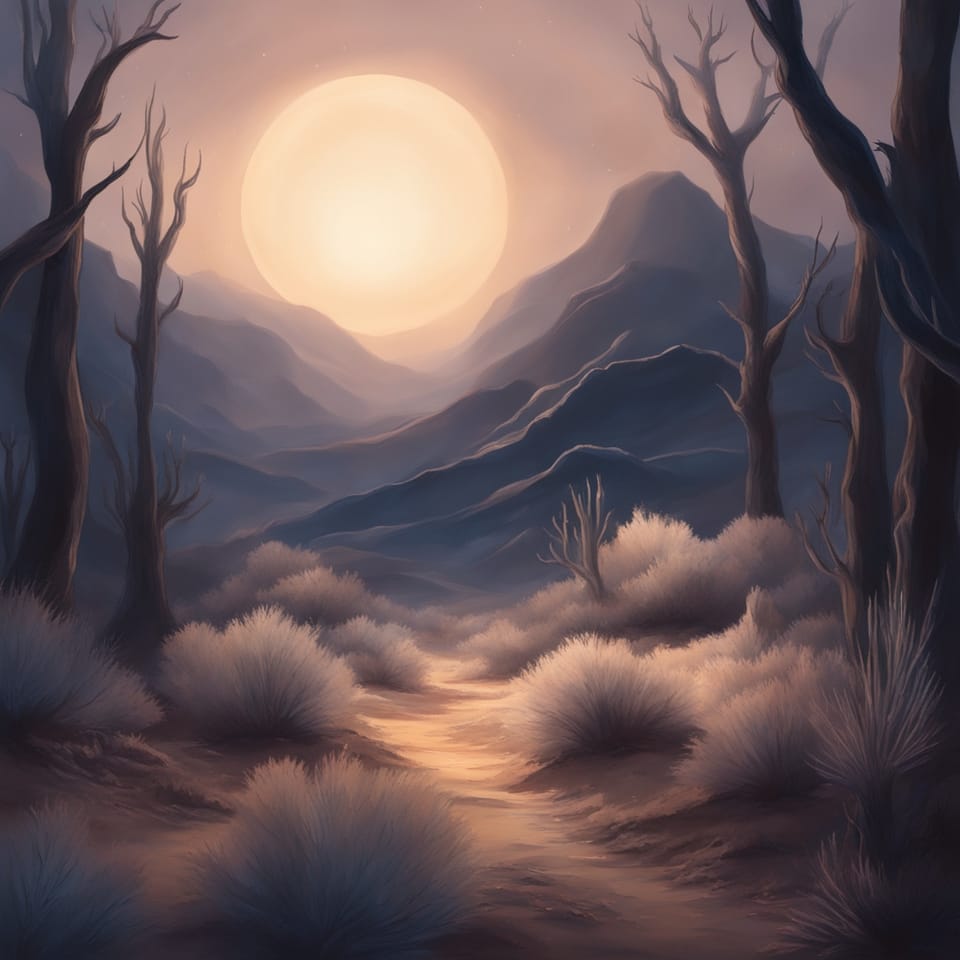
Method Overloading
Method overloading is where you have the same method name but the parameters are different. The parameters can be different types or a different number of parameters. There are two different reasons why you'd use method overloading. Understanding these two use cases will help simplify method overloading and implement it properly.
Overloading With Different Number of Parameters
When you are overloading methods with a different number of parameters, this is done to provide default values. You will only have a primary method that actually has an implementation. The primary method takes all parameters and is where you are going to put the code for the implementation of that method. The other methods will be one-line methods that simply call the next overloaded method with the default values.
// Java code
public void method(final int x) {
method(x, 0);
}
public void method(final int x, final int y) {
method(x, y, "");
}
// Primary method
public void method(final int x final int y, final String s) {
// ...
}
This is the proper way to implement this type of method overloading. You will notice that each method doesn't call the primary method directly. Instead, the one argument method calls the two argument method. The two argument method calls the three argument method. This avoids duplicating the default values.
You will also notice that the position of each parameter in the method stays in the same location. The method parameter x is always the first parameter, y is the second parameter, and s is the third parameter. If a method was added with a fourth parameter, it would be added at the end, and this would become the primary method. The three argument method would then call the four argument method.
Default Parameter Values
Some languages, such as Scala, support the ability to declare a method and give default values to the parameters. Default parameters exist so you do not have to use the method overloading pattern that was shown in the previous example. You can have one method with all of the default values and only provide what you want when calling the method.
// Scala code
def method(
x: Int,
y: Int = 0,
s: String = "")
If you have this feature in the programming language you are using, you should do this instead of overloading the methods. It is far less code and reads much better.
Overloading With Different Types
Method overloading using different types is used to simplify method naming.
// Java code
public class Report {
public String create(final SalesData salesData) {
}
public String create(final Inventory inventory) {
}
}
When you are using method overloading with different types, they are really independent methods but share the same name. Because of this, you write them like a normal method.
This type of method overloading is available to keep method names simple. If you had to name each of the methods in the previous example something else, it would make the method names more complicated. It would only cause confusion if this were implemented a different way.
Conclusion
When using method overloading, remember the two use cases it is used for. When you are overloading methods that have a different number of parameters, use default values if your language supports it or use the pattern shown above. Method overloading with types is there to keep method names simple.