Code Indentation and Code Simplicity
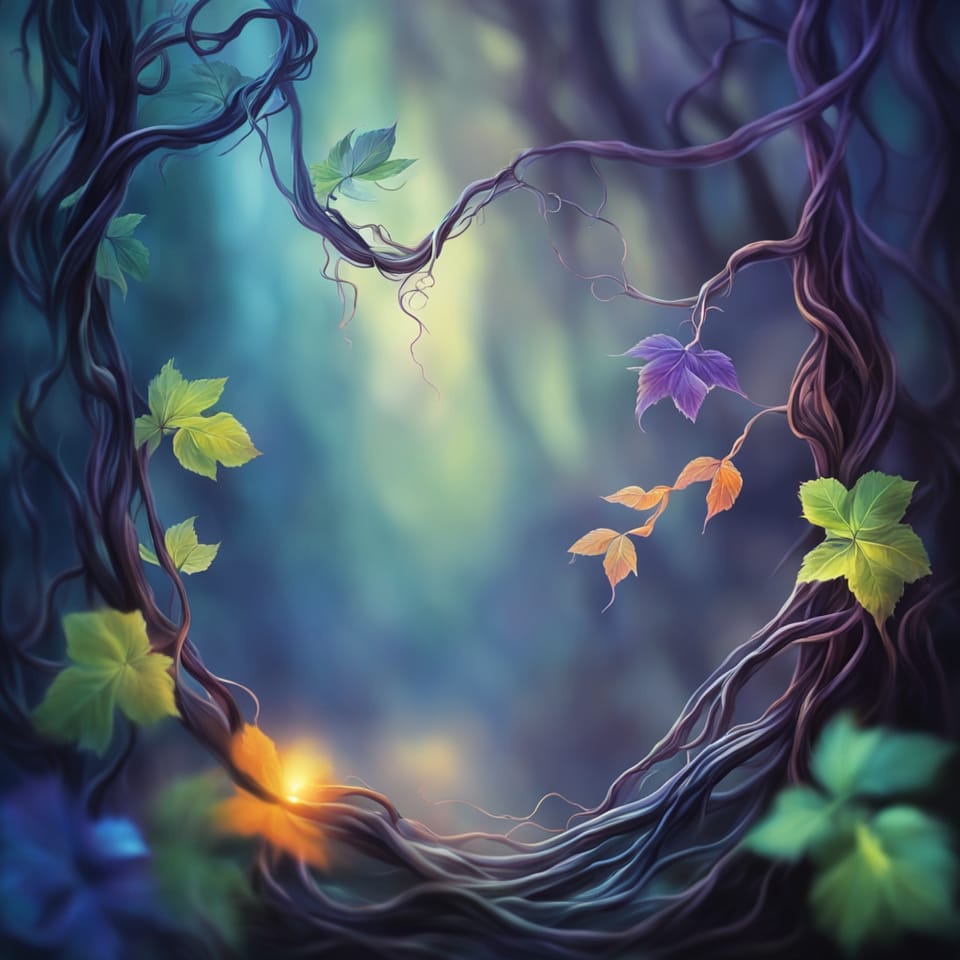
Linux Kernel Coding Standard
The Linux Kernel Coding Style has some simple and common sense standards for code. The standard in this document that stands out the most, in my opinion, would be how many times code is indented. This document states:
"...if you need more than 3 levels of indentation, you’re screwed anyway, and should fix your program." Linux kernel coding style
This could be one of the best code standards for simplifying code.
Benefits of the Three-Indentation Max
If you were to implement one standard in your code base to give the biggest benefits without having to learn anything, restricting the number of times code can be indented would be one of the top things that could be done. If you think about this, there are full books on how to write code well. A lot of what is taught in those books will naturally be done by sticking to this standard.
Arguably the best coding standard is to do one thing. The hardest thing about doing one thing is what is one thing? One thing changes based on context. One thing could be a business concept or a control flow statement. By keeping a three indentation max standard in your code, the code stays simple, will more than likely do one thing, and still allows some room to not introduce the complexity that can come from oversimplifying the code.
If you were a new developer and started with this standard, this would force you to learn how to write code better.
Implementing a Three-Indentation Max
The Linux kernel is predominantly C code. When writing C, you can write functions without indenting your code. The following shows code with a three-indentation maximum:
// C code
void myFunction() {
int i;
if (condition) {
for (i = 0; i < 5; i++) {
printf("Iteration number: %d\n", i);
}
}
}
In Java, everything has to be a class. This causes the code to be indented once before you can start coding.
// Java code
public class App {
public void myMethod() {
int i;
if (condition) {
for (int i = 0; i < 5; i++) {
System.out.println("Iteration number: " + i);
}
}
}
}
This is the same code as the C example, but in Java it has an additional indentation. If you had an inner class in Java, this would require one more indent.
// Java code
public class App {
// ...
public class Example {
public void myMethod() {
int i;
if (condition) {
for (int i = 0; i < 5; i++) {
System.out.println("Iteration number: " + i);
}
}
}
}
}
This now pushes one of the lines to be indented five times. If you were using the three-indentation max standard, you can't put any type of control flow in an inner class. This standard becomes harder to implement when you get into cases like this.
Looking at it a different way would be, in the C example, you are allowed three indentations inside a function. The more generalized standard could be that methods and functions should not contain more than three levels of indentation.
method_signature() {
indentation_1
indentation_2
indentation_3
}
This allows you to follow this standard regardless of how you are forced to write code in the programming language you are using.
Markup Languages
Markup languages such as HTML (HyperText Markup Language) can be indented a lot. This three-indentation maximum standard shouldn't be applied to markup languages. It doesn't make sense because it isn't a programming language. You would end up having more problems than benefits.
Conclusion
Stick to a standard of methods and functions that shouldn't be indented more than three times. This will naturally make your code better and simpler.