Comments in Swift
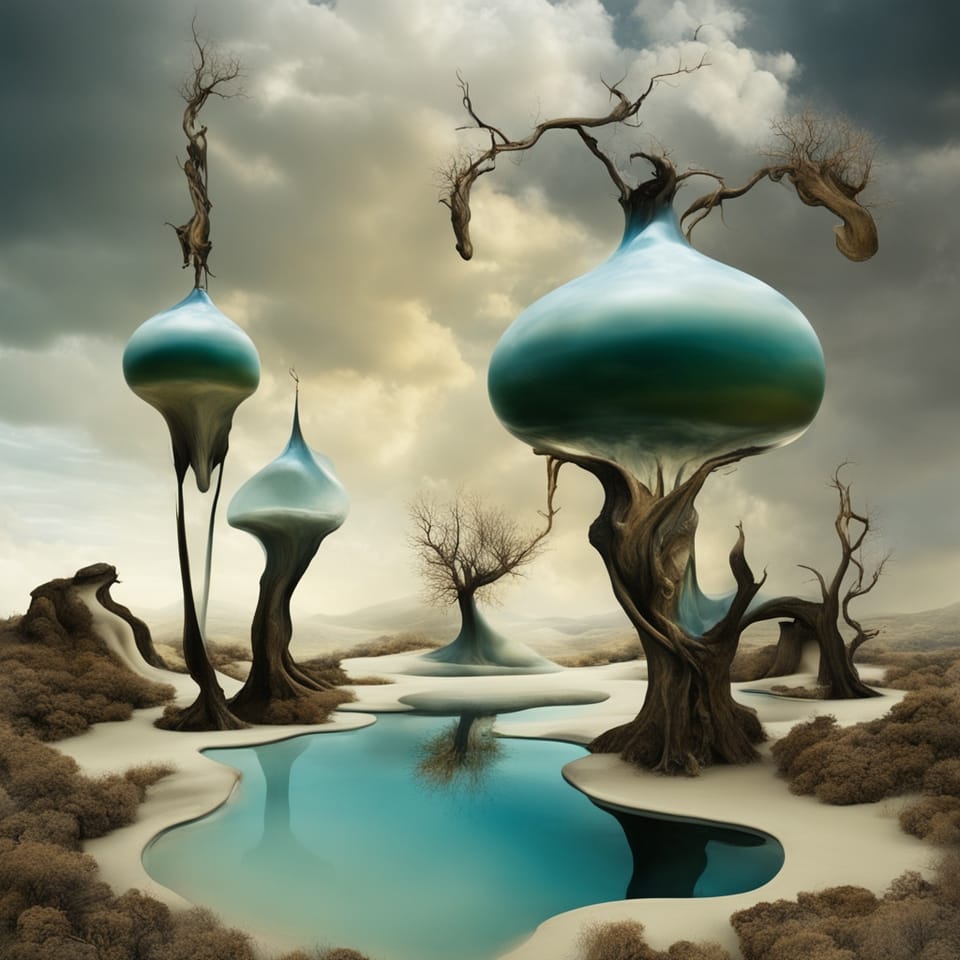
What are Comments?
Comments are used to document code. They are ignored and removed at compile time. After the comment definition, everything that follows on that line of code will be ignored and removed by the compiler at compile time. Comments are usually placed on their own line to document the following line of code. Swift supports three different forms of comments.
Single-line Comments
Single-line comments are written starting with // and then the comment.
// This is a single line comment
They can also come at the end of a line of code.
var orderId = -1 // default value
Multi-line Comments
Multi-line comments start with /* and end with */.
/*
This is
a mult-line
comment in Swift
*/
Documentation Comments
Documentation comments, also called markdown comments, are used to document your public API. They are a special type of comment that allows you to write Swift Markup inside them. This can be done two different ways.
/// Description
/// - Parameters
/// - firstName: firstName description
/// - lastName: lastName description
/// - Returns: description
func concat(firstName: String, lastName: String) -> String {
// ...
}
The other way is similar to the multi-line comment shown above. It is written almost the same but has an additional * at the start of the comment.
/**
Description
- Parameters
- firstName: firstName description
- lastName: lastName description
- Returns: description
*/
func concat(firstName: String, lastName: String) -> String {
// ...
}
In a good IDE, it will usually generate a general template for the parameters, return type, etc.
Conclusion
Comments are used to document code when it is difficult to express what the code is doing with code. They are ignored and removed at compile time.