Comparison Operators in Swift
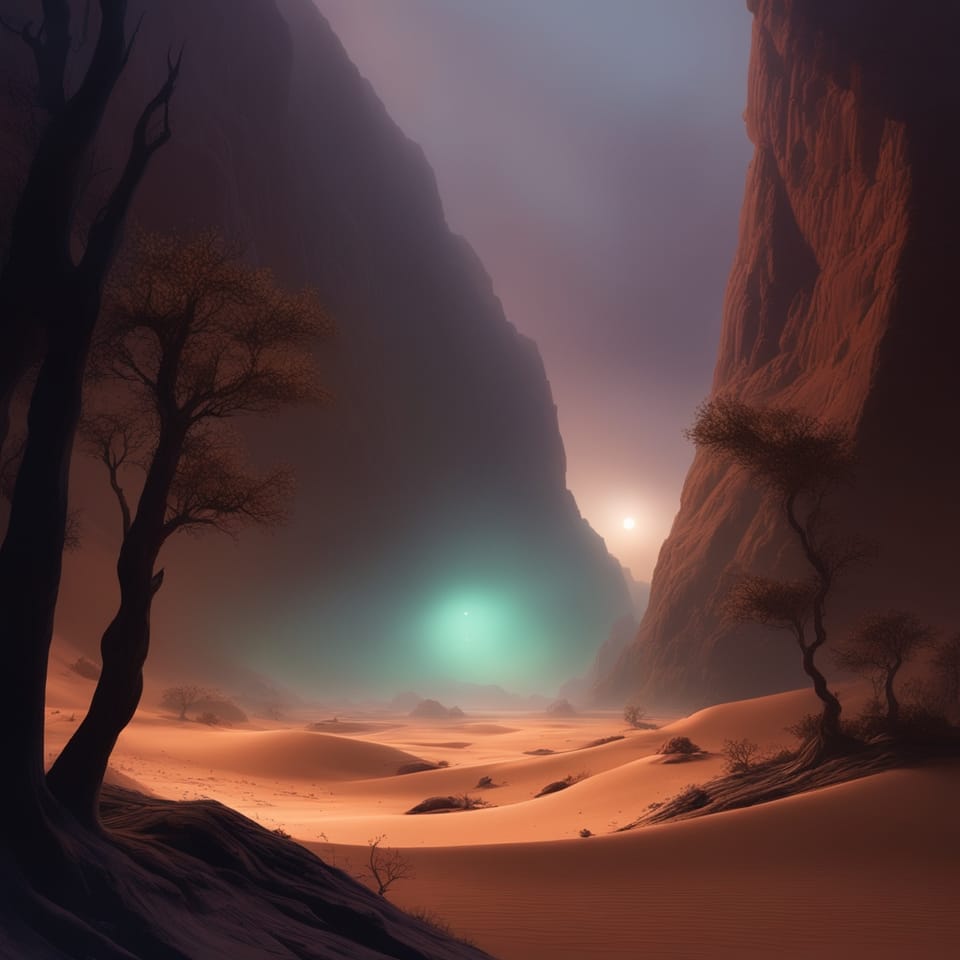
Equality
Checking to see if another value equals another value or doesn't equal a value is something that is commonly done in programming. You can check if a literal value or a variable value is equal to another value. This is done using the equals operator and is written using ==.
let x = 1
let y = 1
print(x == y) // true
print(7 == y) // false
You can check equality regardless of type by converting it to the type you are wanting to check.
let x: Int = 1
let y: String = "1"
print(String(x) == y) // true
print(x == Int(y)) // true
This checks to see if two strings are equal or two ints are equal by converting the type.
You can check to see if two values are not equal using the not-equal operator. This is written using !=.
let x = 1
let y = 1
print(x != y) // false
print(7 != y) // true
Less Than and Greater Than
Just like checking for equality, it is common to provide logic depending on if a value is less than or greater than another value. You can check to see if a value is less than another value using the < operator.
print(4 < 2) // false
print(2 < 3) // true
You can check to see if a value is greater than another value using the > operator.
print(4 > 2) // true
print(2 > 3) // false
If you want to check to see if a value is equal to or greater than a value or if a value is equal to or less than a value, you can use the following operators:
print(4 >= 4) // true
print(3 <= 2) // false
Each of these examples is using numbers, but you can actually use these same operators for strings as well. Using these operators with strings allows you to check if one string comes before another string alphabetically.
let hello = "Hello"
let goodbyte = "Goodbye"
print(hello > goodbye) // true
print(hello < goodbye) // false
Conclusion
Comparison operators can be used to check to see if a value is equal to or not equal to another value. You can also check to see if a value is less than or greater than another value using comparison operators.