Compiling and Running Your First Java Program
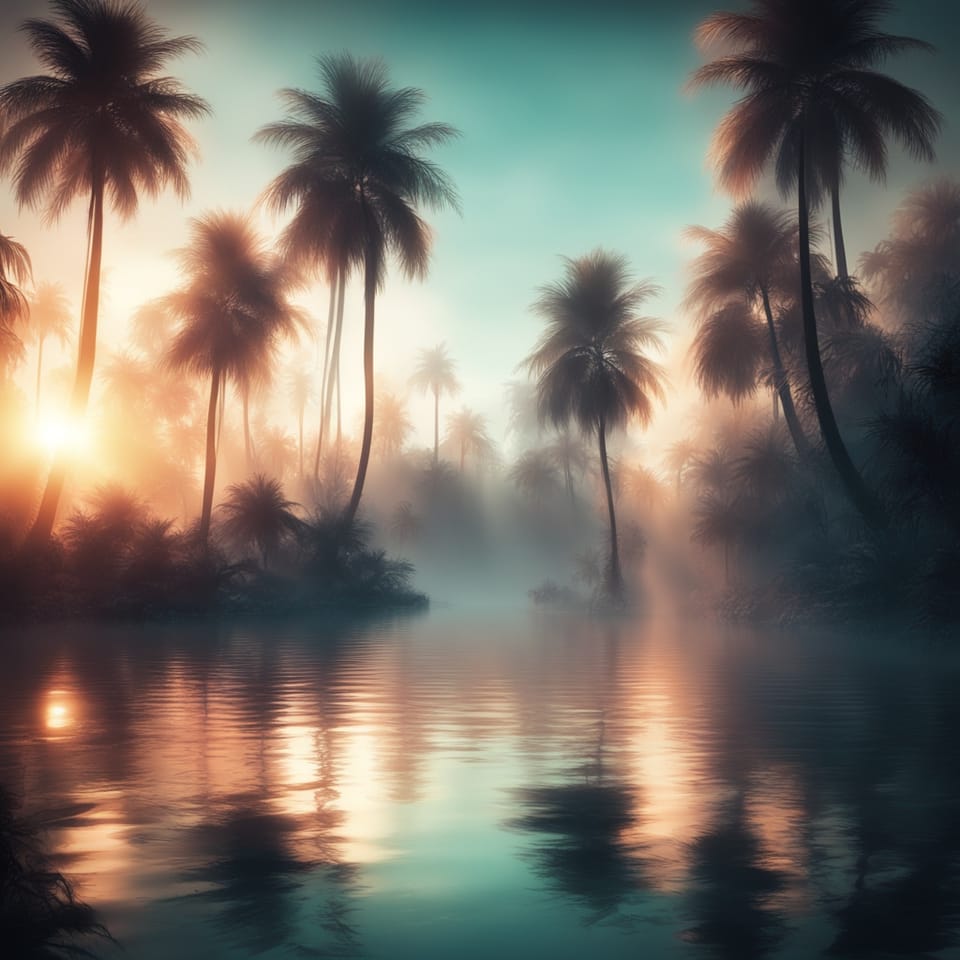
About Java
Java is a compiled language. This means you write Java code and then compile it. When Java code is compiled, it is converted to Java bytecode. Java bytecode is what is executed when the program is run. Java runs through a virtual machine called the JVM (Java Virtual Machine). This allows for a "code once, execute anywhere" approach.
Hello World
Create a file called HelloWorldApp.java. Then put the following code inside of it:
public class HelloWorldApp {
public static void main(final String[] args) {
System.out.println("Hello World");
}
}
Breaking this down, the first line defines a class.
public class HelloWorldApp {
}
Each Java file is required to contain a class. This class is named HelloWorldApp and needs to match the file name, excluding the file extension.
The next line defines the main method.
public static void main(final String[] args) {
}
This is the method that executes when the program starts.
The last line is what actually outputs the "Hello World" text.
System.out.println("Hello World");
Compiling a Java Program
To compile a Java program, on the command line, go to the directory your class file is located in. Once there, execute the javac (Java Compiler) command and pass it the name of the file that was just created.
javac HelloWorldApp.java
This converts the HelloWorldApp.java file into Java bytecode and saves it to a file named HelloWorldApp.class.
Running a Java Program
After the class has been compiled, you can execute the compiled class using the java command and pass it the class name.
java HelloWorldApp
This will run the class HelloWorldApp's main method, which will output "Hello World" to the console.
Conclusion
Writing "Hello World" is a common approach to teaching the basic structure of a software program, how to compile it, and how to execute it. This article covers the structure of the main entry point for an application and the tools used to turn Java code into an executable program.