Constants in Java
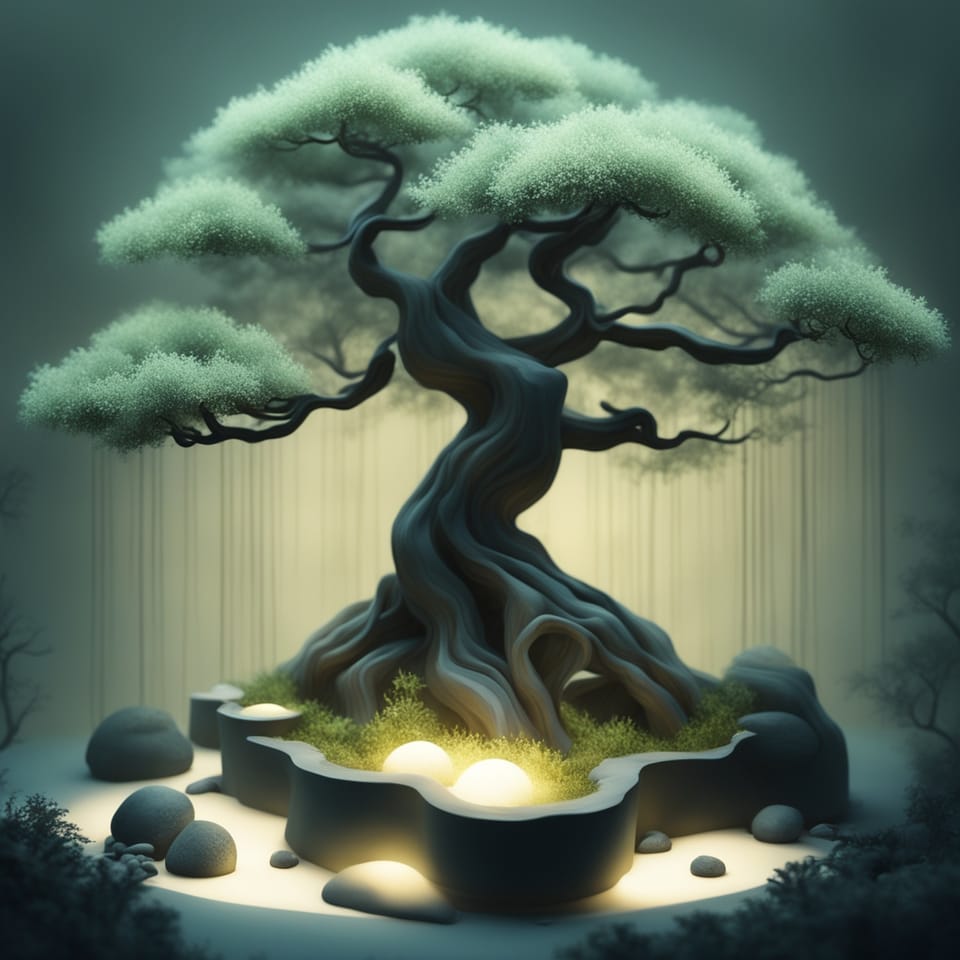
Class Variables
Constants are immutable values. Constant variables can only be declared as class variables. Class variables are static variables defined in a class. Class variables only have one copy of the variable, and it is shared across all instances of that class. To make a class variable constant, you add the final keyword to it.
Constants
Constants start with an access modifier. They are then marked with both static and final keywords, followed by the type and then the constant name. Constant names are written using screaming snake case. An example of a public accessible constant is shown in the following example:
public class Table {
public static final int HEADER_ROW_INDEX = 0;
}
An example of how you access this variable is the following:
if (index == Table.HEADER_ROW_INDEX) {
// ...
}
Static Initialization Blocks
Constants must be assigned a value either when they are defined or in a static initialization block. A static initialization block is similar to a constructor but specific for static variables. The following example shows initializing the previous example in a static initialization block.
public class Table {
public static final int HEADER_ROW_INDEX;
static {
HEADER_ROW_INDEX = 0;
}
}
A value as simple as the one shown in this example wouldn't make sense to initialize in a static initialization block because of the unnecessary complexity it adds. You would use a static initialization block when you have a more complicated object to initialize, such as a map or something that is going to take multiple lines to initialize.
Conclusion
Constants in Java can only be class variables. They are marked with both static and final keywords. More complex constants can be initialized using a static initialization block.