Constants vs Enumerations
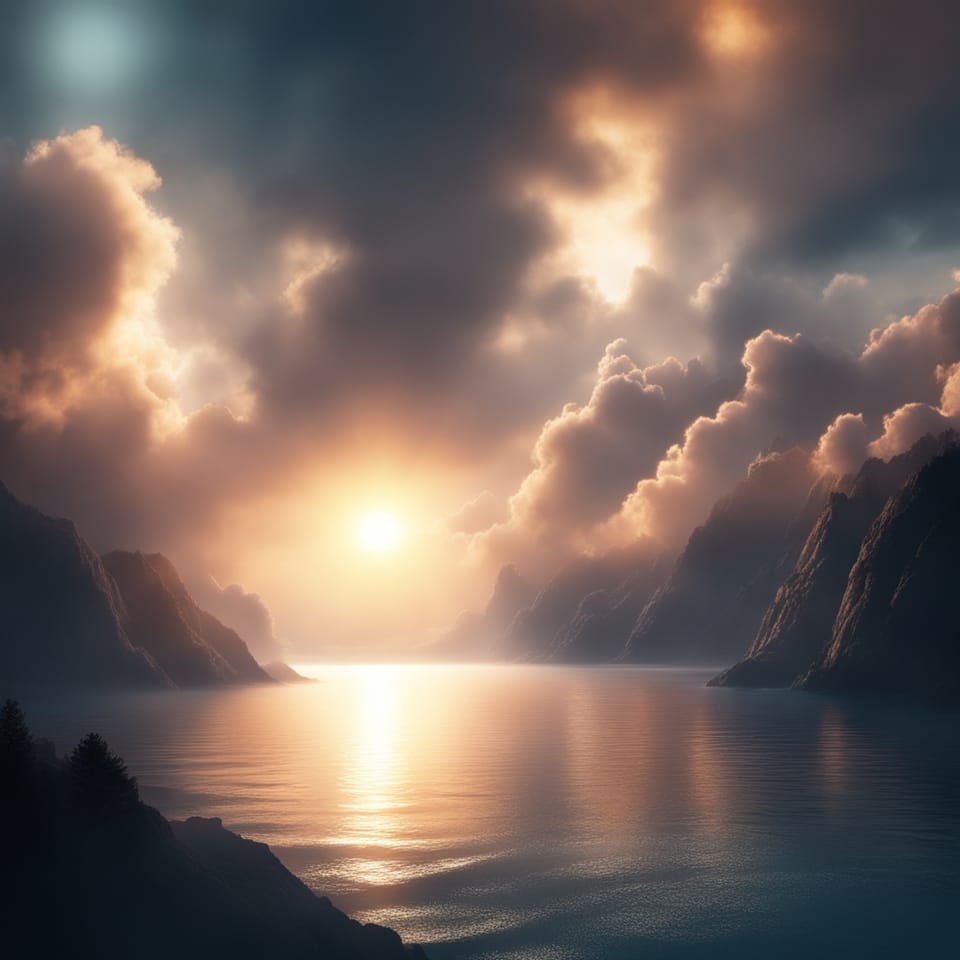
Constants
Constants are a single variable with a value. Constants can be any type but will usually be primitive. An example of a constant would be a string for storing a generic error message. Another example would be a class instance for a singleton pattern. Constants allow you to have a concept in the code of a literal value or a class instance. Constants provide readability and eliminate magic literals.
Enumerations
Enumerations are a set of constants defined as their own type. Enumerations are type safe and increases readability. An example of where you'd use an enumeration instead of constants would be for storing different color values. The enumeration would be called Color, and the values on it would be YELLOW, BLUE, GOLD etc. Some programming languages support enumerations with methods on them.
Using enumerations instead of constants in some languages allows the compiler to do additional work that wouldn't be possible with constants. For example, it is common in languages that support pattern matching to enforce matching on all values of the enumeration. If not all values are matched, the compiler will generate an error.
Enumerations in Action
A good example of the difference between the use of a constant and an enumeration can be shown with the following:
// Java code
public interface Event {
int ON_CLICK = 0;
int ON_KEY_PRESS = 1;
int ON_KEY_RELEASE = 2;
}
This defines an interface that has constant values for events using ints. This really is an enumeration, but it isn't using the language's enum feature. Instead, it is just declaring multiple constant values. You could use the previous code with the following:
// Java code
public void fireEvent(final int event) {
// ...
}
fireEvent(Event.ON_CLICK);
The problem with this is that the type you are dealing with is an int. Nothing is stopping a developer from passing in an invalid value, such as the following:
// Java code
fireEvent(99);
Changing the event parameter to an enumeration creates a new type and restricts what can be passed to that:
// Java Code
public enum Event {
ON_CLICK,
ON_KEY_PRESS,
ON_KEY_RELEASE;
}
public void fireEvent(final Event event) {
// ...
}
fireEvent(Event.ON_CLICK);
A developer can no longer pass in an invalid value to the fireEvent() method.
Conclusion
Constants are variables whose values are fixed and cannot be changed, while enumerations are a type that consists of a fixed set of values. Enumerations provide more flexibility and make your code more readable and type-safe than constants.