Continue Statement in Swift
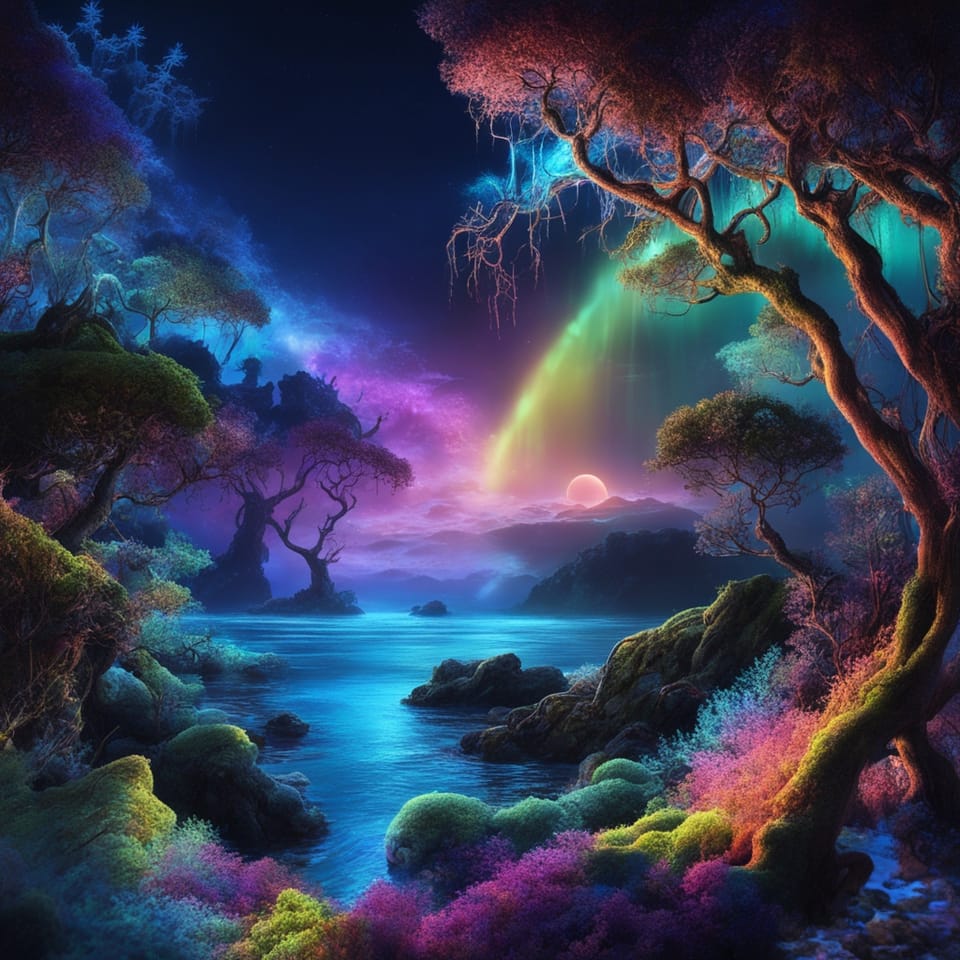
Continue statements allow you to skip to the next iteration in a loop. When a continue statement is encountered, it stops the execution of the current loop's iteration and starts the next one. Continue statements can be used in any type of loop and anywhere in the loop body.
Syntax
Continue statements are simple and are written with the following:
continue
An example of using a continue statement would be the following:
var counter = 0
while counter != 10 {
counter += 1
if counter % 2 == 0 {
continue
}
print(counter)
}
This would print the following:
1
3
5
7
9
Since the if statement checks to see if it is an even number, it will only print out the odd numbers and skip each even number iteration.
Conclusion
Continue statements allow you to skip an iteration of a loop. If you find yourself needing to use a continue statement, a similar approach might be to use an if statement instead of a continue statement to get the same behavior. Continue statements cause complexity in code.