Creating Custom Exceptions in Java
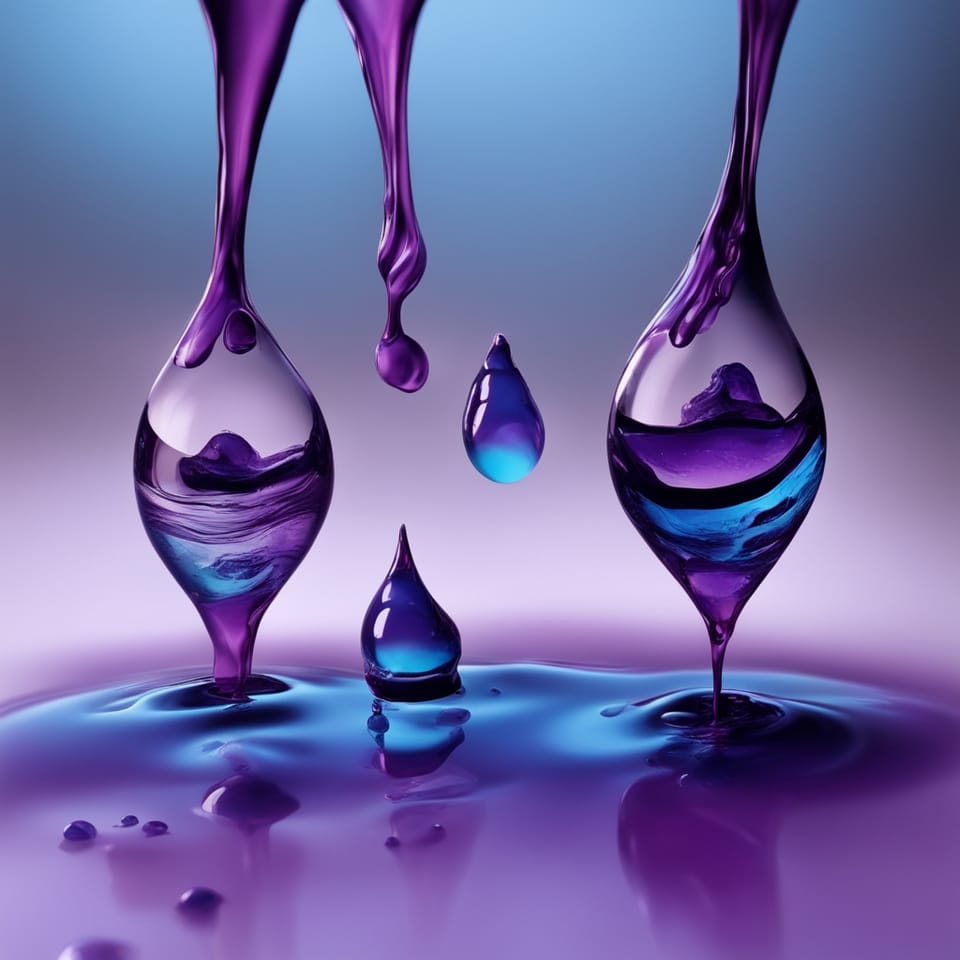
Custom Checked Exceptions
Checked exceptions are exceptions that are checked by the compiler to see if the exception is handled or not. If a method or constructor throws a checked exception, you must either provide a throws clause or handle it in a try block.
Checked exceptions extend the java.lang.Exception class. To implement your own custom checked exception, create a new class and extend Exception.
package press.bytesize.exceptions;
public class MyCheckedException extends Exception {
}
Custom Unchecked Exceptions
Unchecked exceptions are not checked by the compiler and work similar to exceptions in most other programming languages.
Unchecked exceptions extend the java.lang.RuntimeException class. The RuntimeException class actually extends the Exception class, but it isn't a checked exception. To implement your own custom unchecked exception, create a new class and extend RuntimeException.
package press.bytesize.exceptions;
public class MyUncheckedException extends RuntimeException {
}
Constructors
Whether you are creating a checked exception or an unchecked exception, there are multiple constructors you can provide for your own custom exception depending on how you are wanting to use it.
You can provide a constructor that takes a detailed message with the following:
package press.bytesize.exceptions;
public final MyException extends RuntimeException {
public MyException(final String message) {
super(message);
}
}
You can provide a constructor that takes a Throwable of the cause of the exception.
package press.bytesize.exceptions;
public final MyException extends RuntimeException {
public MyException(final Throwable cause) {
super(cause);
}
}
If you would like to provide both of these values, you can do it with the following constructor:
package press.bytesize.exceptions;
public final MyException extends RuntimeException {
public MyException(
final String message,
final Throwable cause) {
super(message, cause);
}
}
If you don't need any of these for your custom exception, you do not have to provide any constructor and can just use the default no argument constructor.
Using Custom Exceptions
Using a custom exception is the same as using any other exception or class. First import the custom exception if it isn't in the same package. Second, you create and throw it just like any other exception.
import press.bytesize.exceptions.NotAuthenticatedException;
// ...
if (!isAuthenticated(user)) {
throw new NotAuthenticatedException();
}
Conclusion
Exceptions are simply a class that extends either Exception or RuntimeException. If you want a checked exception, extend Exception. If you want an unchecked exception, extend RuntimeException.