Document the Why, not the What
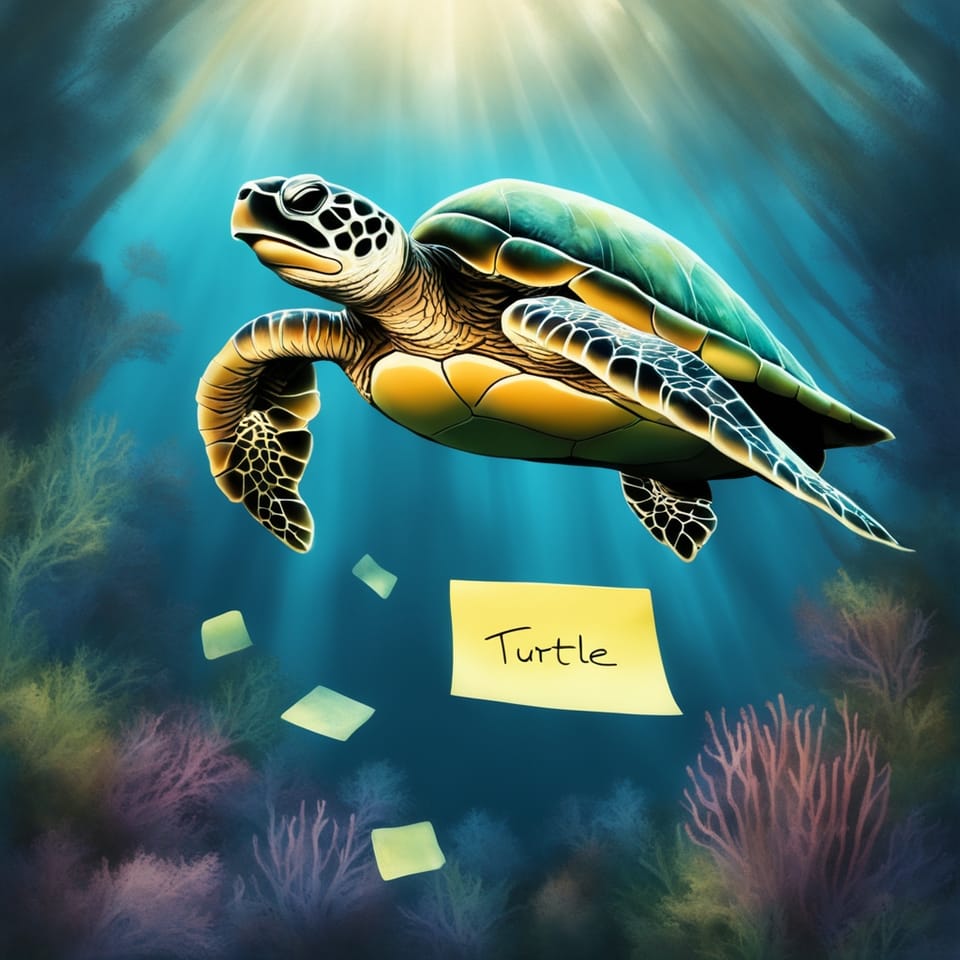
What Developers Are Taught
Comments in code can be a blessing, but more often than not they are useless. The value of the comment depends on its content. Early in a developer's career, they are taught to document their code. However, developers end up describing what the code is doing, instead of why the code is doing what it is doing.
Documenting the What
Here is an example of what you will commonly see in code.
// Java code
/**
* User class to store user information
*/
public class User {
// the user id
private String id;
// the user name
private String name;
// Constructs a new user
public User(
final String id,
final String name) {
this.id = id;
this.name = name;
}
/**
* Gets the user id
*/
public String id() {
return id;
}
/**
* Sets the user id
*/
public void setId(final String id) {
this.id = id;
}
/**
* Gets the user name
*/
public String name() {
return name;
}
/**
* Sets the user name
*/
public String setName(final String name) {
this.name = name;
}
}
None of these comments add any benefits to code. They are completely useless and are only making the code harder to read. They are simply restating what the code is doing.
Don't document with a comment what you can't document with code. Code is what developers read and what is being executed. The example above is straight forward and doesn't need any documentation.
Documenting the Why
Why you do something in code can't always be described with code. More often than not, you will have to use a comment. This is when you want to use a comment. Sometimes in code you may do things different than you normally would, maybe for performance reasons. This is a good example of documenting the why.
Another example is documenting design decisions that are made.
// Java code
public class Comment {
// ...
@Column(
name = "content",
nullable = false,
updatable = false,
// The database engine we are using doesn't take a performance
// hit by using text instead of varchar. We will enforce the
// length in code so we can easily change this if we need to.
columnDefinition = "text"
)
private String content;
// ...
}
This class is using JPA (Java Persistence API) to generate the database schema. The comment in this example describes why the decision was made to use the column data type that is being used.
The following code shows documenting the why due to having to support legacy code.
// Java code
public Optional<User> findById(final String id) {
try {
return Optional.of(super.findById(id));
} catch (final NoResultException e) {
// Due to having to support legacy code, we have to break
// the normal pattern here of throwing an exception and
// instead return an empty Optional.
return Optional.empty();
}
}
Conclusion
When documenting your code, don't clutter it with restating what the code is doing. Wait until you need to explain why you are doing what you are doing and describe it well.