Extends vs Implements In Java
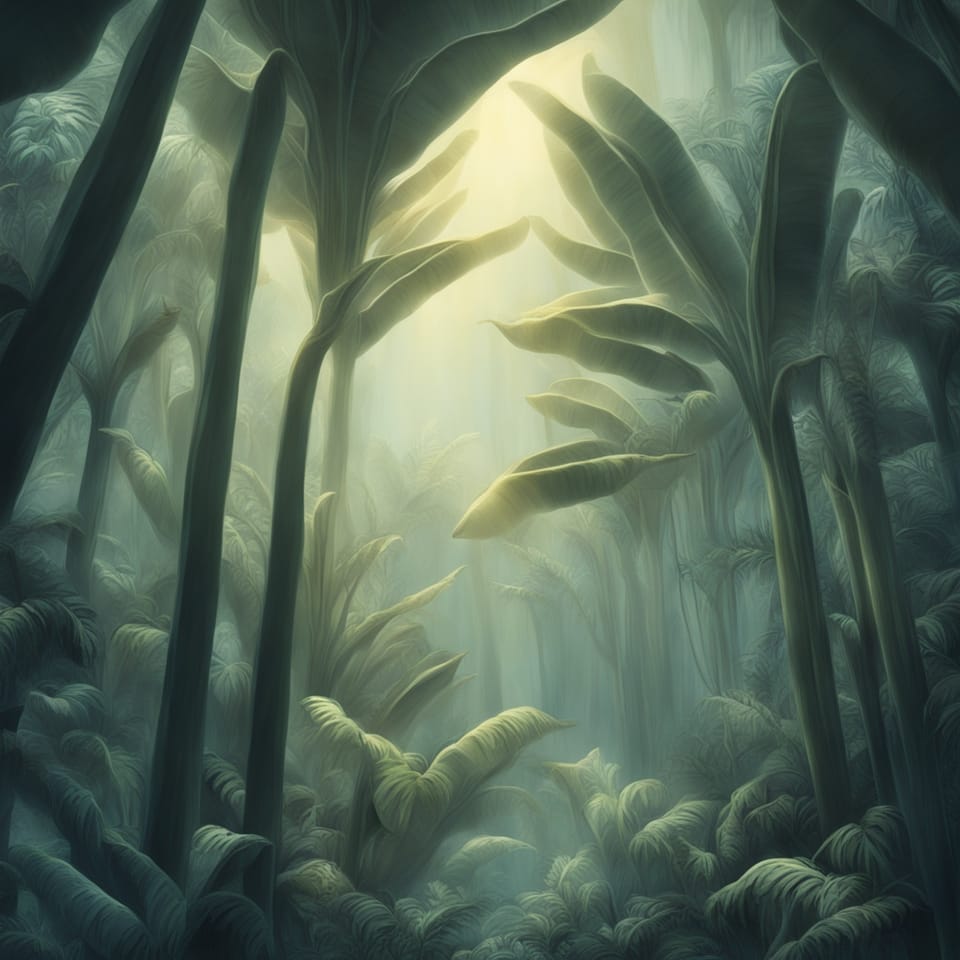
extends Keyword
The extends keyword is used for both class inheritance and interface inheritance. The extends keyword comes after the class or interface declaration. The following shows a class that inherits another class.
public class Message {
// ...
}
publi class Comment extends Message {
// ...
}
In this example the Comment class inherits the Message class. Java doesn't support multiple inheritance, so a class can only extend a single class.
The extends keyword is also used with interface inheritance. Interfaces unlike classes support multiple inheritance. An interface can inherit one or more interfaces. These are written with a comma separating each interface.
public interface Identifiable {
Object id();
}
public interface Copyable {
Object copy();
}
public interface Message extends Identifiable, Copyable {
// ...
}
implements Keyword
The keyword implements is used when a class implements one or more interfaces. It comes after the class declaration and after the extends clause if there is one.
public interface Copyable {
Object copy();
}
public class Message implements Copyable {
@Override
public Object copy() {
// ...
}
}
When a class implements an interface, it must provide an implementation of each method in that interface unless the class is an abstract class. The previous example shows the Copyable interface that has a copy() method declared in it. The Message class is not marked as abstract, so it has to implement the copy() method, or you will get a compiler error.
An implements clause can have one or more interfaces that a class must implement. This is written with a comma separating each interface.
public class Message implements Copyable, Comparable, Serializable {
// ...
}
Interfaces cannot use the implements keyword to implement another interface. They can only inherit other interfaces using the extends keyword.
Using Both extends and implements
A class can both inherit another class and implement one or more interfaces. The class declaration using both of these would look like the following:
public class Comment
extends Message
implements Copyable, Serializable {
// ...
}
A class must use this order when using extends and implements.
Conclusion
When using both class inheritance and interface inheritance, you use the extends keyword. When a class implements an interface, you use the implements keyword. Don't confuse interface inheritance with using the implements keyword instead of extends.