For Loops in Swift
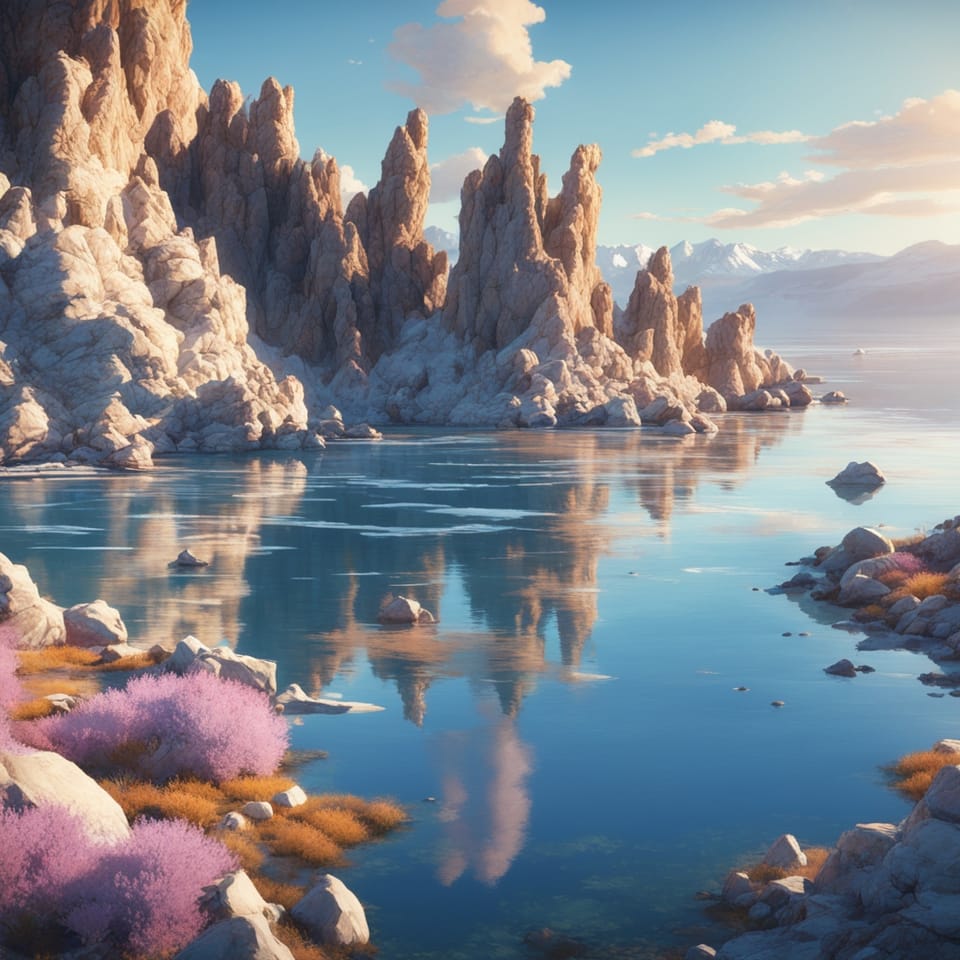
For Loops
A for loop allows you to iterate over a sequence of elements. These can be elements in a collection or a range. The following shows iterating over the elements of an Array:
let numbers = [1, 2, 3, 4, 5]
for number in numbers {
print(number)
}
This will produce the following output:
1
2
3
4
5
You can iterate over a key/value pair of a dictionary using a for loop with the following:
let inventory = [
"Eggs": 5,
"Bananas": 10,
"Apples": 3
]
for (item, amount) in inventory {
print("\(item): \(amount)")
}
This approach, you name the key and value variables that will be used in the for loop. This will produce the following:
Bananas: 10
Apples: 3
Eggs: 5
You can also use a for loop to iterate over a range.
for number in 1...5 {
print(number)
}
This will produce the following:
1
2
3
4
5
Unnamed Variables
There are some cases where you are going to want to iterate over values, but you do not need a variable defined for each value. In this case, you can ignore the variable with the following:
var size = 0
for _ in 1...10 {
size += 1
}
print(size) // 10
Where Clause
It is common to have to filter the data in a for loop and only iterate over the filtered data. For example, the following for loop only prints even numbers:
for number in 1...10 {
if number % 2 == 0 {
print(number)
}
}
For loop support this type of filtering in a where clause. Using where allows you to eliminate the if statement and an additional indentation in the code. The previous example can be written using a where clause with the following:
for number in 1...10 where number % 2 == 0 {
print(number)
}
This allows for a much cleaner approach to the iteration in your for loop. Both of these examples will produce the following output:
2
4
6
8
10
Stride
The stride() function allows you to implement a for loop similar to how you would in a C-like language but with much more readable syntax. There are two stride() functions. The difference between the two is if the number you are iterating to is inclusive or exclusive. The following shows the stride function where the number to iterate to is exclusive.
for number in stride(from: 0, to: 10, by: 2) {
print(number)
}
This starts at 0 and goes until 10 and increments each iteration by 2. This will produce the following output:
0
2
4
6
8
The second stride() function is a closed range, and the number to iterate to is inclusive. Instead of a to parameter, it has a through parameter.
for number in stride(from: 1, through: 10, by: 1) {
print(number)
}
This will produce the following output:
1
2
3
4
5
6
7
8
9
10
Conclusion
For loops are a way to iterate over collections and ranges. They allow you to add a where clause to filter each iteration. You can also use the stride() function for more of a C-like style for loop.