Function Parameters vs Function Arguments
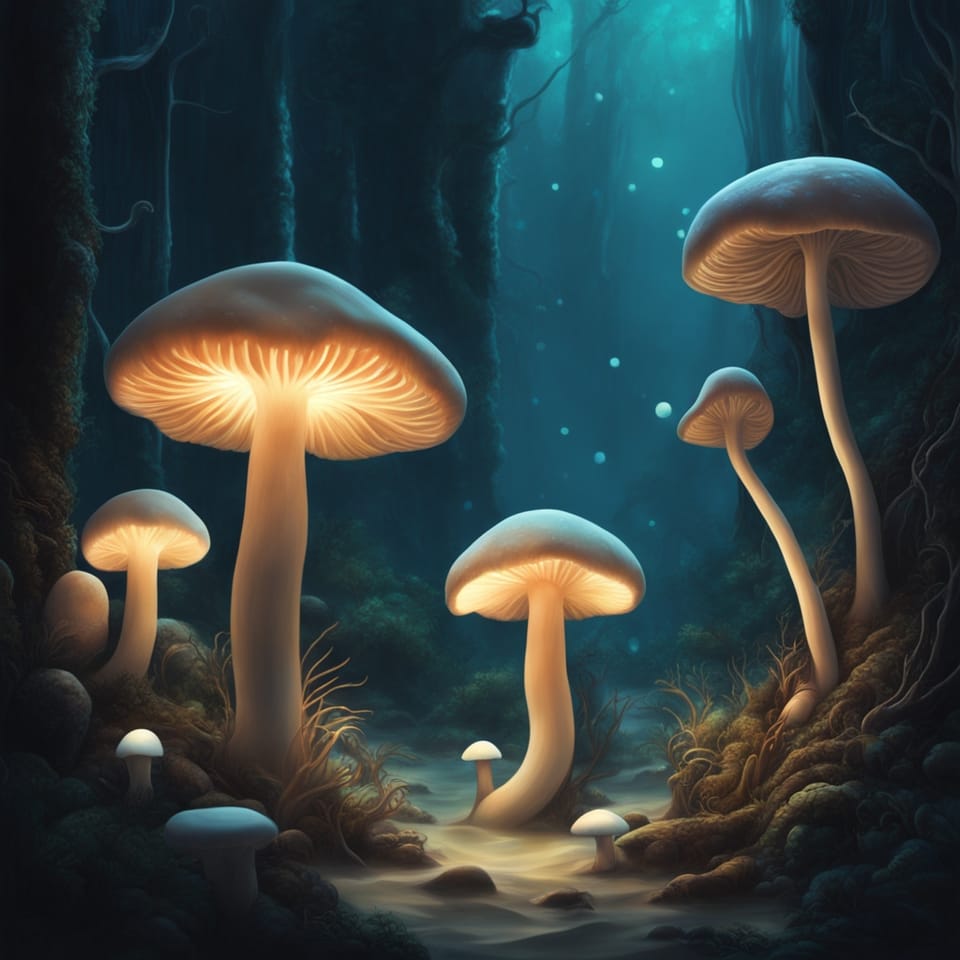
Function and Method Parameters
A function or method parameter is both a name and a type. Function and method parameters are defined in the signature of the function or method. For example, this function has two parameters.
// Java code
public int add(final int num1, final int num2) {
return num1 + num2;
}
Just like when you declare a variable, a variable has a name, just like a parameter has a name.
// Java code
// Declare a variable named greet
final var greet = "Hello";
Function and Method Arguments
Arguments are what is passed to a function or method when it is invoked. The following shows an example of invoking the method above and passing arguments 1 and 2 to it.
// Java code
add(1, 2);
The parameter num1 is passed the argument 1, and the parameter num2 is passed the argument 2. Just like when you assign a variable a value, arguments are the values for the method parameters.
// Java code
// Assigned value "Hello"
final var greet = "Hello";
When you pass values to a command line program, these values are considered arguments.
cp /path/to/file1 /path/to/file2
This example passes the arguments /path/to/file1 and /path/to/file2 to the cp command.
Functions in Swift
The Swift programming language adds an additional concept to what is being discussed. In Swift, you also have named arguments. In Swift, by default, when you invoke a function, you provide the parameter names. For example, given the following function:
// Swift code
func add(num1: Int, num2: Int) -> Int {
return num1 + num2
}
When you invoke it, you do so with the following:
// Swift code
add(num1: 1, num2: 2)
This code is kind of weird at first. Swift's goal is to make methods read like sentences. Because of this, you can name the arguments separately from the parameter names. Given the following function:
// Swift code
func showSearchForm(for user: String, themedIn theme: String) {
setUser(user)
setTheme(theme)
}
The first name is the argument name; the second is the parameter name, followed by the type. This allows you to use different names depending on if you are working in the function or invoking it. You could invoke the above with the following:
// Swift code
showSearchForm(for: adminUser, themedIn: "darkmode")
This code reads just like a sentence.
Conclusion
Function and method parameters and arguments are related concepts but are two separate things. Parameters are the name and type, while the argument is the value for the parameter.