Grouping Statements by Concept, not by Variable
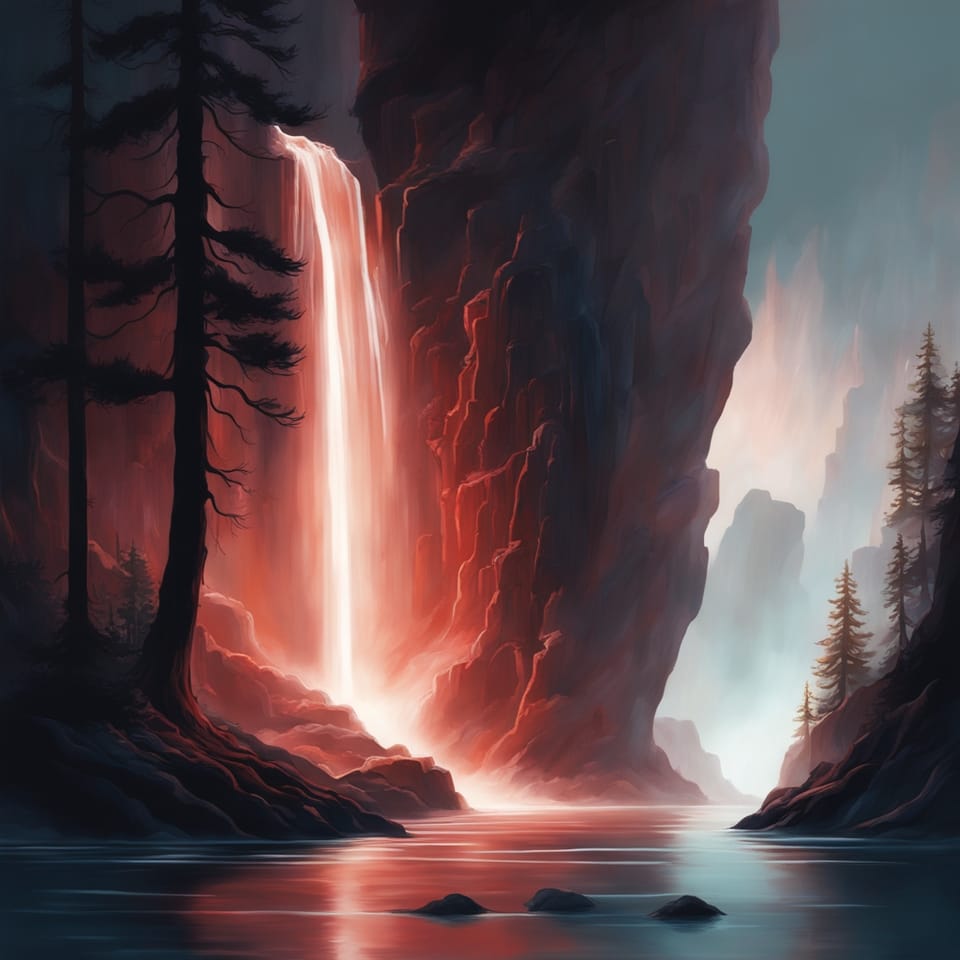
Organizing Statements
When someone starts to program, it is natural for them to group statements around a variable for whatever reason. This type of organizing doesn't make sense and is rarely necessary. The better way to organize statements is by a concept. If you were looking for a calculation in code, are you going to be looking for variables that are declared or are you going to look for a calculation?
Grouping by Variable
An example of grouping statements by variable would be the following code.
final var ok = new Button();
ok.setEnabled(true);
ok.setText("OK");
ok.addClickListener(this::okClicked);
layout.add(ok);
final var cancel = new Button();
cancel.setEnabled(false);
cancel.setText("Cancel");
cancel.addClickListener(this::cancelClicked);
layout.add(apply);
final var apply = new Button();
apply.setEnabled(false);
apply.setText("Apply");
apply.addClickListener(this::applyClicked);
layout.add(cancel);
This code is not a natural way to read instructions. It is hard to wrap your head around code grouped like this because the thought process is bouncing all over the place. It is like reading three books all at once, but only a sentence at a time from each book.
This type of grouping also encourages copying and pasting code, which can lead to code duplication. If an additional button were to be added to the previous code example, one of these code blocks would be highlighted and copied and pasted.
Grouping by Concept
Using the previous code example but grouping by concept instead would look like the following:
final var ok = new Button();
final var cancel = new Button();
final var apply = new Button();
ok.setEnabled(true);
cancel.setEnabled(false);
apply.setEnabled(false);
ok.setText("OK");
cancel.setText("Cancel");
apply.setText("Apply");
ok.addClickListener(this::okClicked);
cancel.addClickListener(this::cancelClicked);
apply.addClickListener(this::applyClicked);
layout.add(ok);
layout.add(apply);
layout.add(cancel);
This code is grouped by concept, easy to read, and quick to understand. This specific code example really gets cleaned up by having the layout code all in one spot. You don't have to jump all over the place trying to find out how it is laid out.
In addition to grouping statements by concept, it helps the readability of the code to add a new line to separate concepts.
Another benefit of grouping by concept is that it allows you to easily extract methods when you need to. The above example could have methods extracted for each concept.
Conclusion
Grouping by statements makes the code faster to understand and easier to read. It allows you to filter the code to the concepts that are relevant for the task you are working on.