Guard Statements in Swift
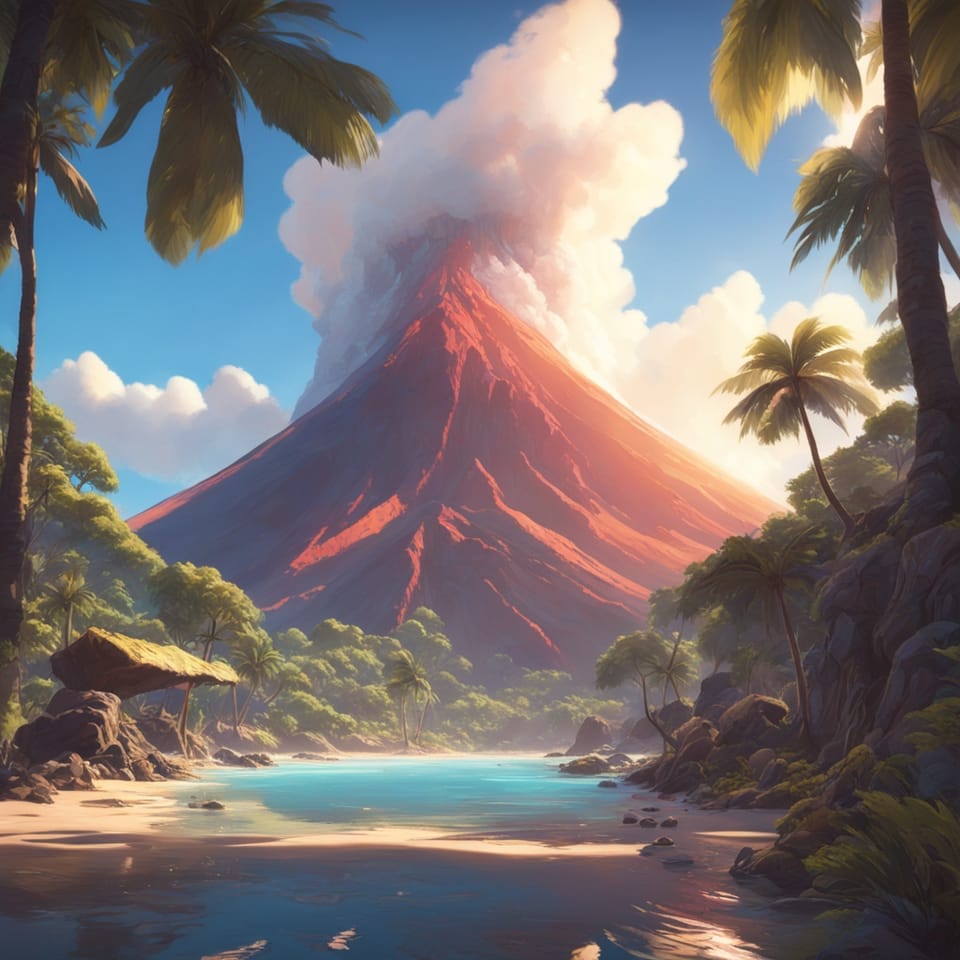
Guard Statements
A guard statement is a statement that is at the beginning of a method. They are used to prevent further execution of the method by either throwing an error or returning. In most programming languages, when you write a guard statement, you will usually use an if statement to do so. Swift has the concept of a guard statement as part of the language.
Guard Statement Syntax
Guard statements are written similarly to an if/else but only provide the else block. A guard statement is written with the following syntax:
func ping(_ connection: Connection) {
guard connection.isAlive() else {
return
}
print("Sending ping...")
}
A guard statement starts with the guard keyword followed by the condition that needs to be met for the method to continue to execute past the guard statement. It is then followed by the else keyword and the block of code to execute if the condition evaluates to false.
The compiler will ensure that you have a control transfer statement such as return, throw, break, or continue, or a call to fatalError(). If it doesn't contain a control transfer statement, it will result in a compiler error.
func ping(_ connection: Connection) {
// Compiler error:
// 'guard' body must not fall through,
// consider using a 'return' or 'throw' to exit the scope
guard connection.isAlive() else {
}
print("Sending ping...")
}
Option and Guard Statements
Guard statements can be used with Options. This allows you to check to see if an Option has a value as well as assigning the value of the Option to a variable that can be used through the rest of the method. This is done with the following:
func greet(_ name: String?) {
guard let personsName = name else {
return
}
print("Hello \(personsName)")
}
Conclusion
Guard statements are a great way to write the precondition a method must have in order for that method to execute. They are great because you get additional help from the compiler to make sure there is a control transfer statement.