How Many Return Statements Should a Function Have?
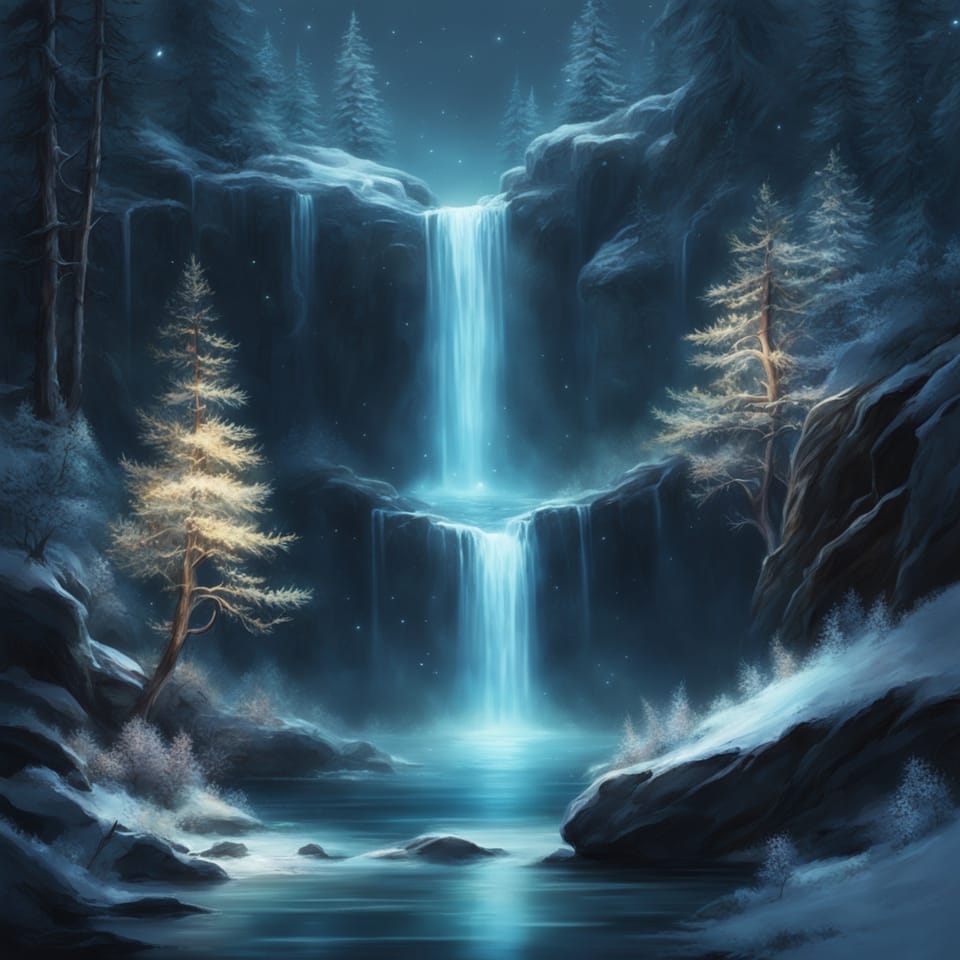
One Return Statement
There is an argument that functions should only have one return statement. This is a bad standard, especially for modern languages.
The following code example is attempting to show a complex function with multiple pathways through the code. Each pathway only has one line, but more real world examples could be much more complicated.
// Java code
public String doSomething() {
String result;
if (condition1) {
if (condition2) {
if (condition3) {
result = "condition3";
} else {
result = "condition3-else";
}
} else {
result = "condition2-else";
}
} else if (condition4) {
result = "condition4";
} else if (condition5) {
result = "condition5";
} else {
result = "else";
}
return result;
}
This gets confusing to follow. You have to analyze the whole function instead of just returning and being done. This makes it harder to enhance or change the function because of the different pathways.
Multiple Return Statements
Although having one return statement isn't necessarily bad in some cases, it should never be a standard. One reason for single return statements is an attempt to simply the function. The number of return statements a function has isn't the problem; it is the complexity of the function. Simpler functions never have problems with more than one return statement.
The previous example could be rewritten using multiple return statements.
// Java code
public String doSomething() {
if (condition1) {
if (condition2) {
if (condition3) {
return "condition3";
} else {
return "condition3-else";
}
} else {
return "condition2-else";
}
} else if (condition4) {
return "condition4";
} else if (condition5) {
return "condition5";
} else {
return "else";
}
}
You don't have to try to follow what may or may not be executing because of the single return statement. This could also make debugging easier and setting break points depending on what the code is doing and the complexities of it.
Guard statements are a great way to simplify code and reduce indentation.
// Java
public String doSomething() {
// Guard
if (isAuthenticated()) {
return "";
}
// ...
}
They couldn't be used if a function can only have one return statement.
Conclusion
The number of return statements isn't the problem, it is the complexity of the functions. Keep functions simple and doing one thing, and you don't have to worry about the number of return statements a function has.