How to Know What Collection to Use
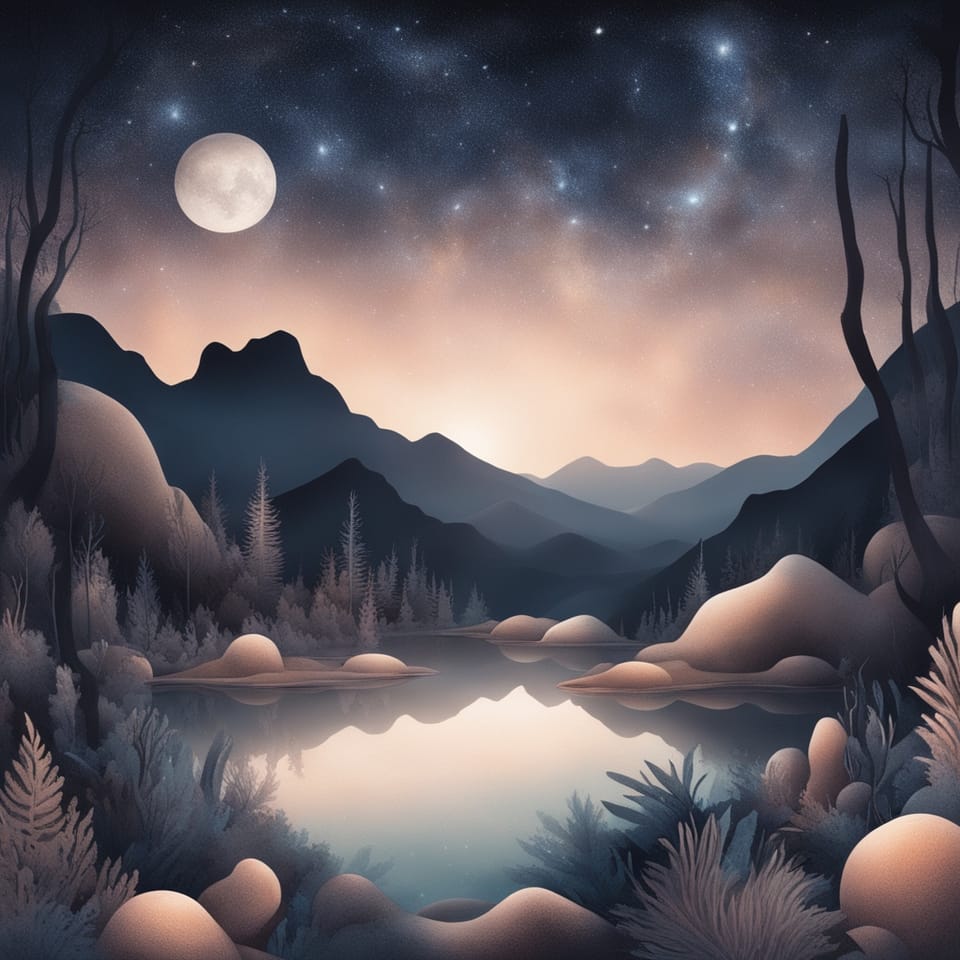
Collections
Collection types are the same regardless of your language of choice; however, they might be called by different names. The examples in this article will use Java.
Before you decide what implementation you need, you must first understand collections and the data that needs to be stored in them.
Arrays
Arrays are a non-unique collection of items that are fixed in length. Arrays are used more in legacy code, low-level code, or to build other collections. Arrays are what are used when working with bytes, such as reading a file.
// Java code
final var array = new String[2];
array[0] = "a";
array[1] = "b";
// returns "a"
array[0];
// returns "b"
array[1];
Lists
Lists are a non-unique collection of items sorted by the order in which they are added. Lists are used when order matters and are not fixed in size. This is generally going to be the collection you are going to use more often than not.
// Java code
final var list = new ArrayList<String>();
list.add("a");
list.add("b");
// returns "a"
list.get(0);
// returns "b"
list.get(1);
Sets
Sets are an unsorted, unique collection of items. Sets are used when you need to guarantee that the items are unique. When you need to store items that are unique and order doesn't matter, use this collection.
// Java code
final var set = new HashSet<String>();
set.add("a");
set.add("b");
// Prints each item in an unsorted order
set.forEach(item -> System.out.println(item))
Stacks
Stacks are a first-in, last-out collection of items. Items are added through a push() method and removed with a pop() method. Stacks are what are used for stacktraces to track the method calls.
// Java code
final var stack = new Stack<String>();
stack.push("a");
stack.push("b");
// returns "b"
stack.pop();
// returns "a"
stack.pop();
Queues
Queues are a first-in, first-out collection of items. Items are added through the add() method and are removed with the poll() method. Queues are used when you need to queue something up, like a song on a playlist.
// Java code
final Queue<String> queue = new LinkedList<>();
queue.add("a");
queue.add("b");
// Returns "a"
queue.poll();
// Returns "b"
queue.poll();
Deque
Deques are both a stack and a queue. They can have items added or removed from both the front and end of the collection. They have the methods add() and poll() like queues do, and they have push() and pop() like stacks do. Deques also have their own methods for adding and removing items from both the front and the back of the collection. Deques are used in features such as undo/redo.
// Java code
final Deque<String> deque = new ArrayDeque<>();
deque.addLast("a");
deque.addLast("b");
deque.addFirst("c");
// returns "c"
deque.getFirst();
// returns "b"
deque.getLast();
Maps/Dictionaries/Associative array
Maps, sometimes called dictionaries or associative arrays, are a key/value pair of items. Each key is unique and is associated with a value.
// Java code
final Map<String, Integer> map = new HashMap<>();
map.put("a", 10);
map.put("b", 20);
// returns 10
map.get("a");
// returns 20
map.get("b");
Conclusion
Using the right collection for the job will simplify your code and boost performance. You will avoid writing code that has already been written for you.