How to Simplify if Statements
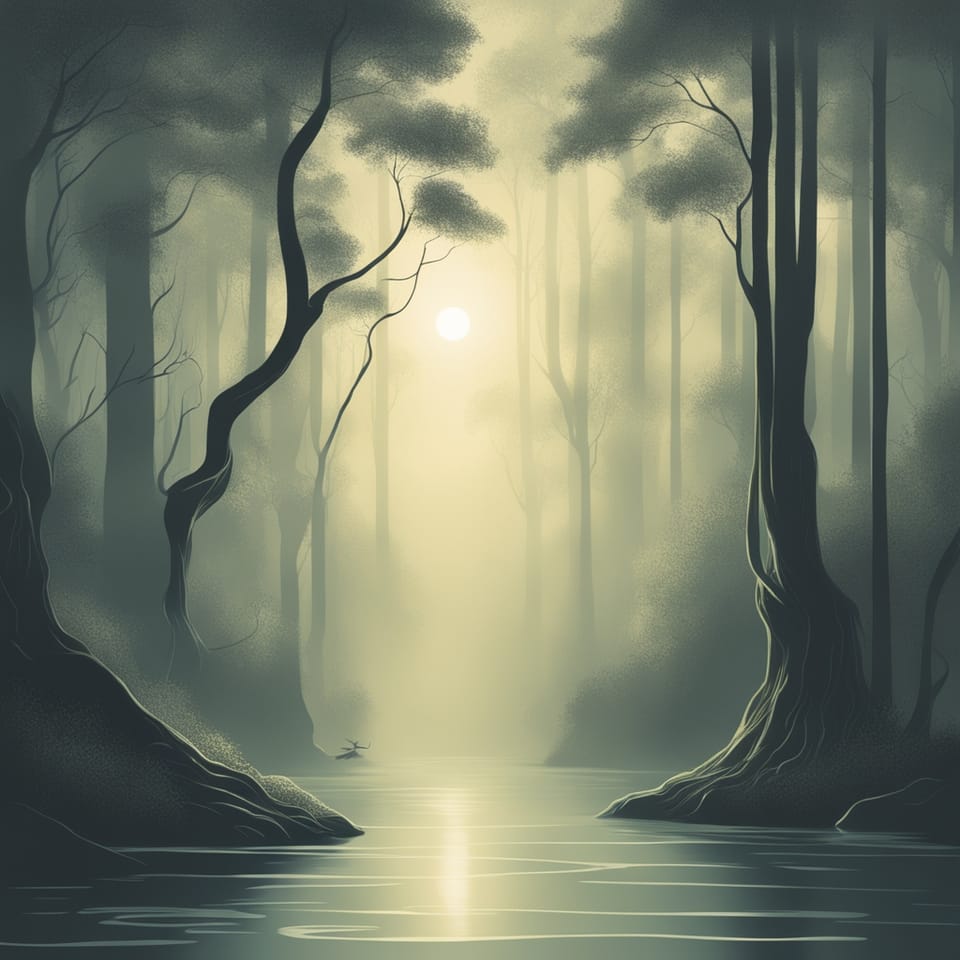
What You Usually See
Complex if statements are generally the result of fitting too many things into one concept. If statements should conditionally test one concept. If statements should always be simple, no matter how complex the logic is. Developers tend to think too much in code. Here is an example:
if (index == 0) {
// ...
}
What is the concept behind the condition that is being tested? The answer is not "if the index is equal to 0." That is simply explaining what the condition is checking. What is the underlining concept of index == 0? Nobody knows because the developer is thinking too much in code instead of concepts. To fix this generally you'd see the following.
// is the table header row selected
if (index == 0) {
// ...
}
Good if statements don't need comments describing what they do. Instead, code will be translated into a concept anybody can understand.
Thinking in Terms of Concepts
You need to think in terms of concepts that anybody can understand. This is how you are able to eliminate comments in if statements and simplify them. Rewriting the previous example to the following, you can now tell what it is and even get an idea of what type of application it might be in.
final var isTableHeaderRowSelected = index == 0;
if (isTableHeaderRowSelected) {
// ...
}
This code translates the conditional into a concept that anybody can understand. The hardest part about writing if statements is generally translating code into concept.
It is important that you take your code and create a concept that a non developer can understand. For example, the following code doesn't do anything for anybody.
final var isFirstOrLastNameEmpty =
firstName.isEmpty() || lastName.isEmpty();
if (isFirstOrLastNameEmpty) {
// ...
}
The only thing you have done is created a variable that explains the code. You now have two different ways to read the code for the same thing. You'd be better leaving it as the following:
if (firstName.isEmpty() || lastName.isEmpty()) {
// ...
}
A better way to write this would be:
final var formDataSubmittedIsIncomplete =
firstName.isEmpty() || lastName.isEmpty();
if (formDataSubmittedIsIncomplete) {
// ...
}
A more complex example of an if statement not using concepts would be.
if (
(!firstName.isEmpty() && firstName.length > MIN_FIRST_NAME_LENGTH) &&
(!lastName.isEmpty() && lastName.length > MIN_LAST_NAME_LENGTH) &&
(!email.isEmpty() && email.isValid())) {
// ...
}
You can simplify this by using concepts with the following:
final var isFirstNameValid =
!firstName.isEmpty() && firstName.length > MIN_FIRST_NAME_LENGTH;
final var isLastNameValid =
!lastName.isEmpty() && lastName.length > MIN_LAST_NAME_LENGTH;
final var isEmailValid =
!email.isEmpty() && email.isValid();
final var userDataSubmittedIsValid =
isFirstNameValid && isLastNameValid && isEmailValid;
if (userDataSubmittedIsValid) {
// ...
}
This example builds concepts upon concepts.
Typically, you will use a local boolean variable to create these concepts. If you need it in more than one place, or if you have expensive operations that need to be lazily executed, you'll be using a method or function instead.
Conclusion
The rules for writing if statements are simple when it comes to programming constructs. Think instead of concepts and not programming logic.