If Statement vs Guard Statement
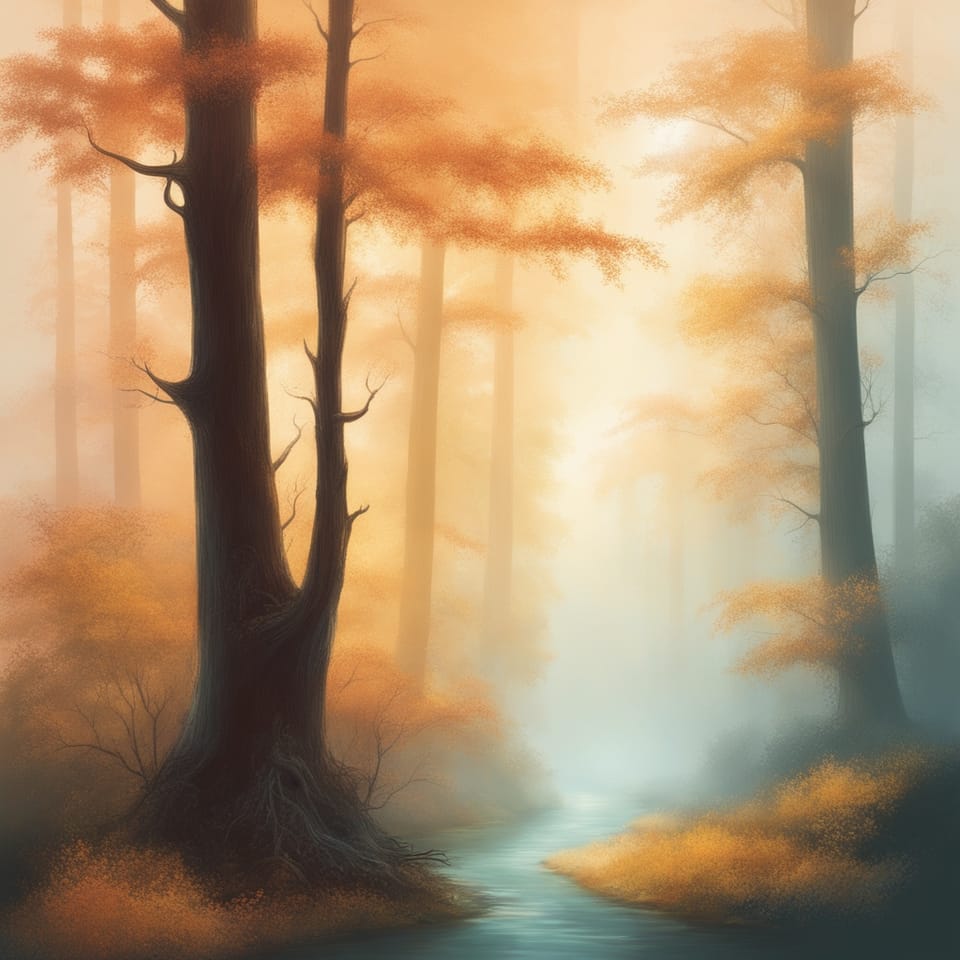
If Statement
If statements control the flow of code execution. It checks to see if an expression evaluates to true. If it does, it then executes the code block. If statements have multiple blocks of code but will only execute the block for the expression that evaluates to true first. If statements are used to execute a block of code when something is true. An example of an if statement would be.
// Java
public void doSomething() {
if (index == 0) {
// ...
} else {
// ...
}
}
Guard Statement
Most languages, guard statements are written with if statements. You use guard statements to ensure something isn't false before executing a block of code. Guard statements help insure preconditions of the code are as expected before executing a block of code. They are usually written at the beginning of a function. Guard statements allow you to exit out of a function early and also remove a level of code nesting. Guard statements can simplify code, especially when there are multiple guard statements involved.
With a guard statement, you are usually interested in if an expression evaluates to false instead of true. If you are using if statements to write guard statements, you have to reverse the boolean expression since you are wanting the false evaluation. The following is an example of writing a guard statement.
// Java
public void doSomething() {
if (!connection.isAlive()) {
// return or throws statement
throw new ConnectionException("Connection is dead");
}
// ...
}
In the Scala programming language, you have the require() method. It can be considered a type of guard statement. If the expression passed to the required() method is false, an exception is thrown.
// Scala
def doSomething(): Unit = {
require(connection.isAlive(), "Connection is dead")
// ...
}
Guard statements in Swift
In the Swift programming language, guard statements are built in at the language level. You write guard statements with the guard keyword. Swift's guard statements only execute if the expression evaluates to false instead of true, like an if statement. This makes the code simpler because you don't have to reverse the boolean expression like you do in an if statement. The same example above could be written in Swift using a guard statement.
// Swift
func doSomething() {
guard connection.isAlive() else {
// Must have a return, throw, continue or break.
return
}
// ....
}
Since you are wanting to exit out of a function early, Swift's compiler will actually generate an error if the guard statement doesn't have a throw or return statement.
The Swift compiler will also look for continue and break statements in guard statements when they are inside a loop. This allows you to guard against something in an iteration of a loop.
Conclusion
If statements and guard statements in most languages are both written with if statements. Use guard statements to guard against a condition so a block of code doesn't execute. Use if statements when you need different pathways of execution through the code.