If Statement vs Switch Statement
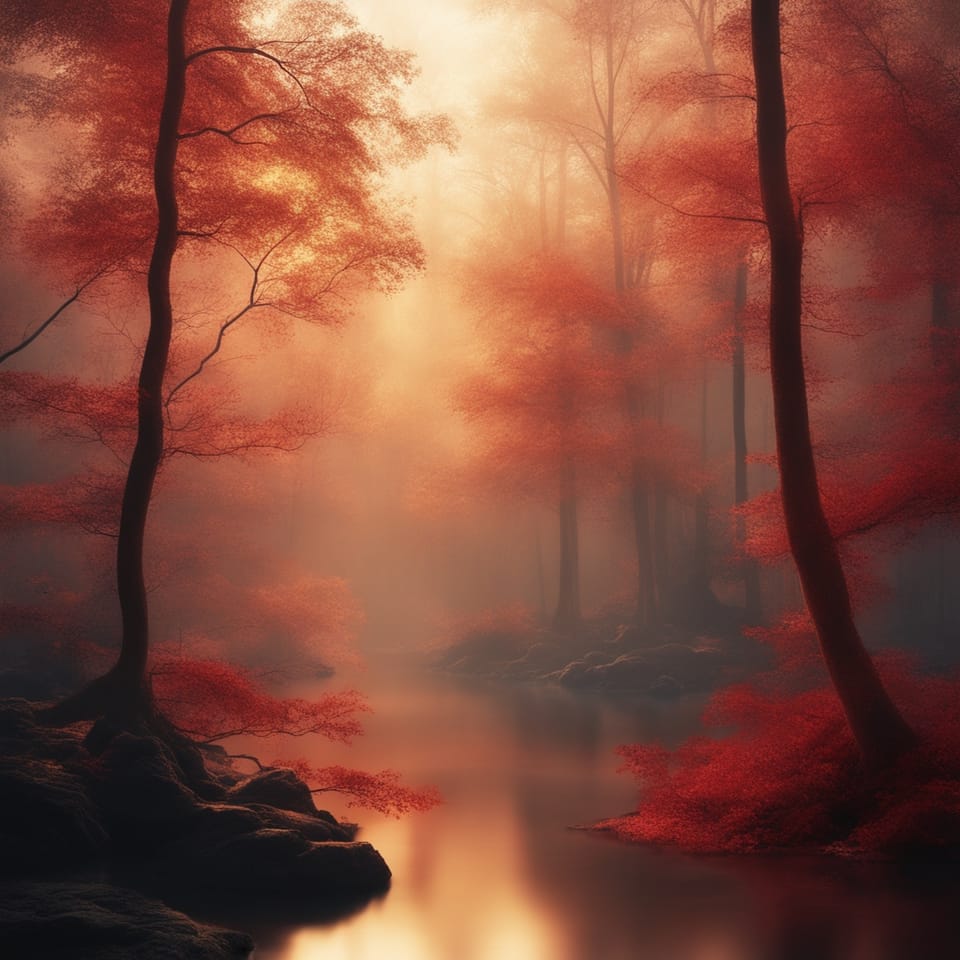
Similarities Between The Two
Both if statements and switch statements evaluate an expression and then execute code accordingly. They are both used for control flow statements. However, they are used in different contexts.
If Statements
The expression evaluated in an if statement is a boolean expression. If statements are used to conditionally offer multiple pathways through code using boolean logic. If you were determining what a user is able to do, you might have code that looks like the following:
if (isUserAuthenticated()) {
if (canView()) {
// code to execute
} else if (canEdit()) {
// code to execute
} else {
// code to execute
}
} else {
// code to execute
}
Switch statements are not used for this.
Switch Statements
Switch statements are used when you want to evaluate the same expression against various values. Unlike if statements, switch statements usually have multiple types of values the expression can return, although this is usually restricted to a set of different types. They can be more than just a boolean value, unlike an if statement. Switch statements also support fall through, where if statements do not.
A common use case for switch statements is mapping the result of an expression to a value or a block of code. For example:
// Java code
public enum DayOfTheWeek {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY;
}
public String toString(final DayOfTheWeek dayOfTheWeek) {
switch (dayOfTheWeek) {
case SUNDAY:
return "Sunday";
case MONDAY:
return "Monday";
case TUESDAY:
return "Tuesday";
case WEDNESDAY:
return "Wednesday";
case THURSDAY:
return "Thursday";
case FRIDAY:
return "Friday";
case SATURDAY:
return "Saturday";
default:
throw new RuntimeException(
"Invalid value: " + dayOfTheWeek.name()
);
}
}
Conclusion
Use if statements when you have complex conditions or multiple conditions and need multiple pathways through the code. Use switch statements for comparing a single expression against multiple values.