If Statements in Swift
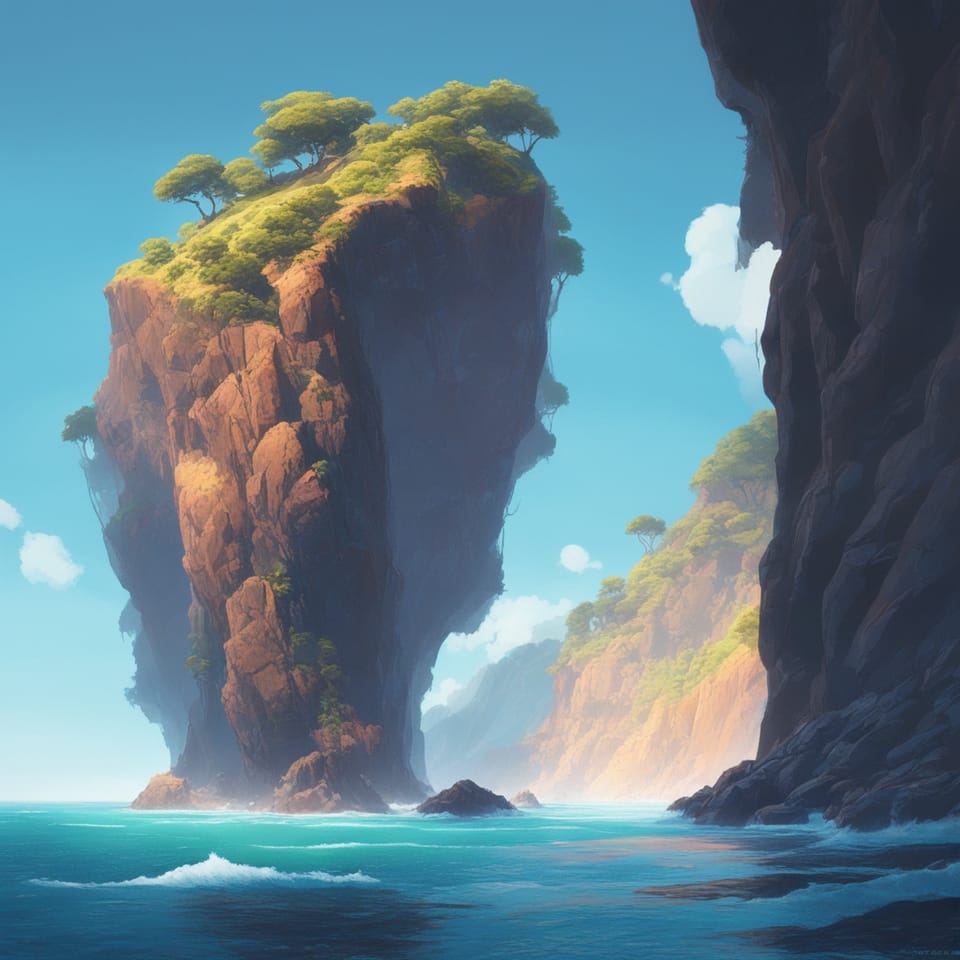
What is an If Statement?
If statements are a way to execute code when a particular condition is true. If statements allow you to have multiple pathways of execution through code. For example, when validating user input, if the data is valid, then you submit it. If the data is not valid, you show the validation errors to the user. This is an example of when you'd use an if statement.
How to Write an If Statement?
If statements are written with the following:
if index > 0 {
print(index)
}
If the condition in the if statement evaluates to true, the block of code inside the if statement will execute.
Multiple Conditions
Sometimes you need to evaluate multiple conditions and execute a block of code when one of those conditions is true. This can be done with an else if statement. An if statement can have zero or more else if statements. They are evaluated in the order they are defined. Once a condition matches, it will execute that block and no other blocks.
if index == 0 || index == -1 {
// ...
} else if index == 1 {
// ...
} else if index > 1 {
// ...
}
This allows you to have more than one pathway through the code. Depending on what index evaluates to, it will change what block of code is executed.
No Matches
If you want to do something if a condition matches and something else if it doesn't, you can provide an else clause to your if statement. If statements can contain zero or one else clause.
if isValid {
print("Valid")
} else {
print("Invalid")
}
If it is valid, it will print "Valid" and if it isn't valid, it will print "Invalid".
Nested If Statements
If statements can be nested inside of an if statement. They are written the same as normal if statements.
if isAuthenticated {
if isAdmin {
// ...
} else {
// ...
}
} else {
// ...
}
If isAuthenticated evaluates to true, it will then execute the nested if statement.
If Expressions
If statements are also expressions. This means that the if statement will return a value. This is useful for when you need to assign a value to a variable. Instead of writing an if statement like the following:
let errorMessage: String
if isFormValid {
errorMessage = ""
} else {
errorMessage = "Form is not valid"
}
You can write an if expression instead with the following:
let errorMessage = if isFormValid {
""
} else {
"Form is not valid"
}
This is much similar and guarantees errorMessage will be assigned a value.
If expressions must have an else clause. If they do not, it will result in a compiler error:
// Compiler error:
// 'if' must have an unconditional 'else' to be used as expression
let errorMessage = if isFormValid {
""
}
The Ternary Operator
The ternary operator is a shorter form of an if/else statement. These are useful when you are assigning a value to a variable in an if statement.
let isValid: Bool
if formIsValid {
isValid = true
} else {
isValid = false
}
This can be written using a ternary instead.
let isValid = formIsValid ? true : false
Ternaries should be used only when they offer more code readability than an if/else statement does.
Conclusion
If statements must contain an if block. They can contain zero or more else if statement and zero or one else statements. They are used to execute different blocks of code depending on a condition.