Instance Methods in Java
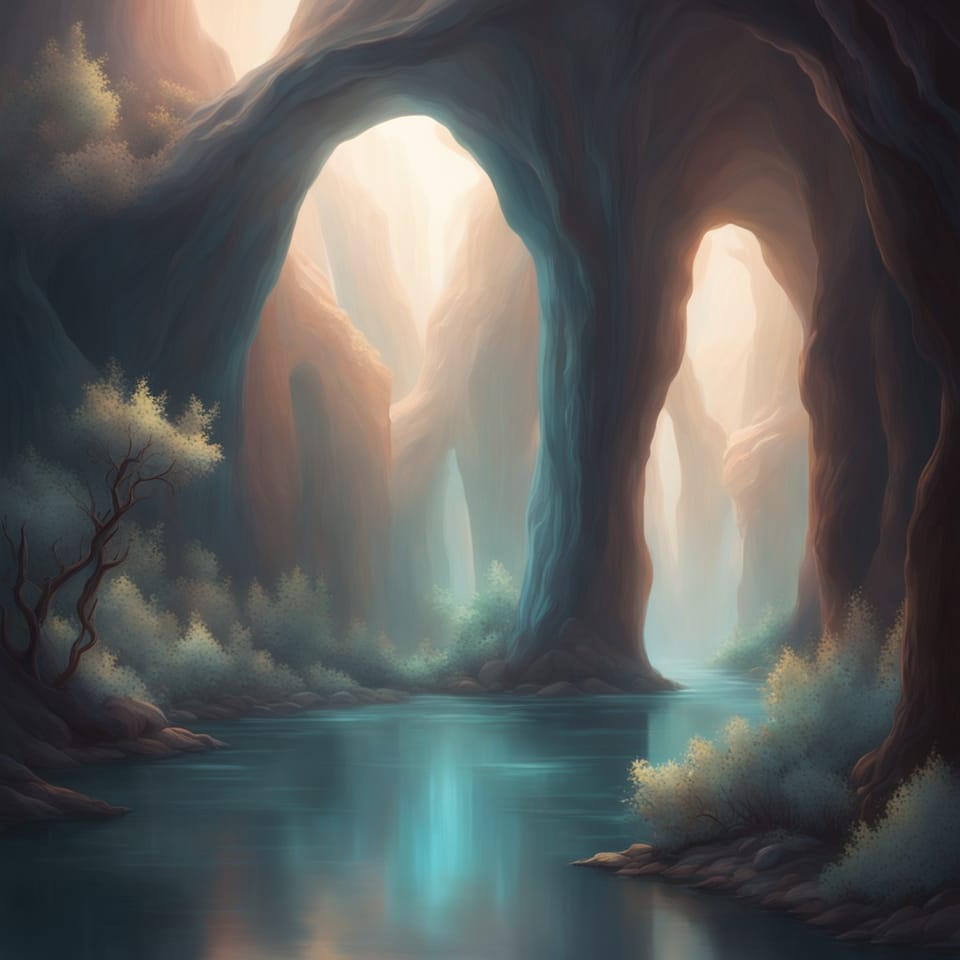
What is a Method?
Methods are a block of code that is a reusable operation. Methods must exist inside of a class. Here is a class with a method defined in it.
public class MyClass {
// Method signature
public void methodName(
final String parameter1,
final String parameter2) {
// Method body
System.out.println(parameter1);
System.out.println(parameter2);
}
}
A method signature has four parts: an access modifier, a return type, a name, and zero or more parameters. After the method signature is the method body. The method in the previous example is a public method named methodName that returns void and has two method parameters. When it is invoked, it will print each of the parameter values.
Access Modifiers
Methods support all access modifiers. They can be private, protected, package-private, or public.
// private
private void method1() {
}
// protected
protected void method2() {
}
// package-private
void method3() {
}
// public
public void method4() {
}
Method Return Type
Methods always have a return type. It can be either void, meaning it doesn't return anything, or a type such as a class or primitive type.
Returning Void
Methods that return void are methods with side effects. A side effect could be modifying some type of state, printing something, deleting a file, etc.
public void greet() {
System.out.println("Hello World");
}
This method returns void, and the side effect is printing something to the screen.
Returning a Value
Methods can return a value of any type. When a method returns a value, it is done using a return statement.
public String greeting() {
return "Hello World";
}
This method returns the value "Hello World" when it is invoked.
If a method returns a value, the compiler will guarantee all pathways through the method return something.
// Compiler Error:
// Missing return statement.
public String greeting() {
if (condition) {
return "Hello World";
}
}
This only returns a value if the if block is executed. If the if block doesn't execute, there isn't a return statement, so you get a compiler error. To fix this, you need to add a return statement for when the if block doesn't execute.
public String greeting() {
if (condition) {
return "Hello World";
}
return "Hello You";
}
When a return statement executes, it stops the execution of the method and returns the value in the return statement. You cannot have code after a return statement, or it will result in a compiler error.
public String greeting() {
return "Hello World";
// Compiler Error:
// Unreachable statement
final String value = "";
}
Method Names
Method names follow the same rules as variable names. Method names should be named starting with a verb.
Method Parameters
Method parameters allow for arguments to be passed to a method. They are declared the same as a variable with a type and a name. Methods can have zero or more parameters.
public int add(final int num1, final int num2) {
return num1 + num2;
}
This method adds both parameter values together and returns the result.
It is a good practice to not modify parameter values. Because of this, it is recommended to always mark your parameters as final.
Instance Variables
Instance variables, as well as class variables and constants, can be accessed inside of methods. This is only going to cover instance variables.
public class User {
// Instance variable
private int id;
public int id() {
return id;
}
public void setId(final int id) {
this.id = id;
}
}
In this example, the id() method returns the instance variable id. In setId(), it sets the instance variable id to the value of the id parameter.
You will notice the use of the this keyword. You use this to specify an instance variable when a local variable or method parameter has the same name as an instance variable. Without using this, the instance variable will be shadowed. This means you will use the local variable or method parameter with the same name instead of the instance variable.
Invoking Methods
When invoking a method, you write the method name, followed by (). If the method has parameters, you provide the arguments inside the parentheses, comma-separated. To show how to invoke methods, the following class will be used.
public class Printer {
private String previouslyPrinted;
public void print(final String text) {
System.out.println(text);
previouslyPrinted = text;
}
public String previouslyPrinted() {
return previouslyPrinted;
}
}
Invoking Methods in a Class
When inside a class, methods can only be invoked inside of constructors and methods. To show this, a greet method will be added to the Printer class.
public class Printer {
// ...
public void greet() {
print("Hello World");
}
}
This calls the print() method in the Printer class when greet() is called.
Invoking Methods on an Object
When you have an instance of a class, you access methods differently. You must first have an instance of a class before you can invoke the methods on that instance.
public static void main(final String[] args) {
final var printer = new Printer();
printer.print("Hey!");
final var lastMessage = printer.previouslyPrinted();
// ...
}
First an instance of Printer is created. Then the print() method is invoked on the printer instance. Last, a variable is defined and assigned the value from the previouslyPrinted() method.
Conclusion
Methods are a reusable block of code defined in a class. They can be invoked inside of the class in constructors and in methods or on objects. They can take parameters, making them very powerful and providing a lot of flexible behavior in your code.