Java Access Modifiers
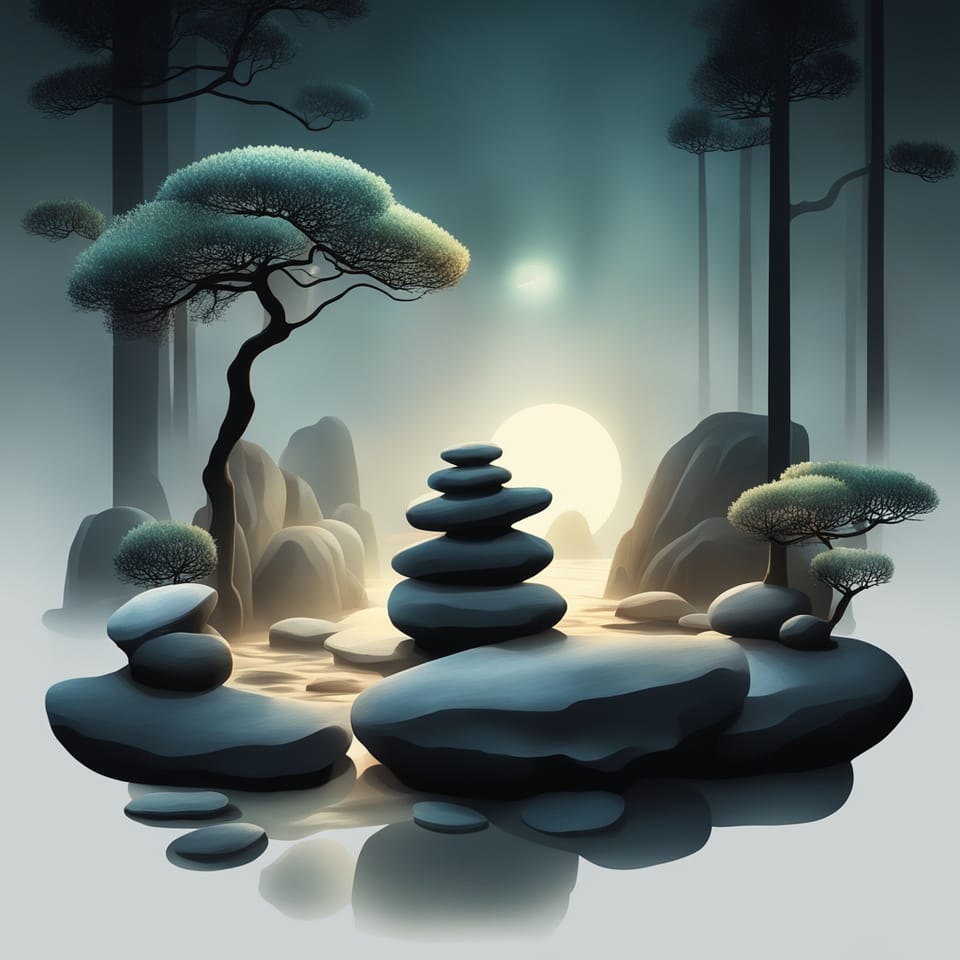
Class Members
In the Java programming language, every file must contain a class. Even if you have a static method somewhere, that method must be inside a class. Because of this, access modifiers apply to the members of a class.
Class members are pretty much anything that can be accessed using dot notation, such as fields, methods, inner classes, nested interfaces, enumerations, etc. This also includes constructors. They are called class members because they are part of the class.
Access Modifiers
Java supports four different accessibility levels and has three different keywords for access modifiers: public, protected, and private.
Public
Members marked with public can be accessed from any class.The following shows a method defined with public access.
public void doSomething() {
}
Package-private
Members that are package-private are available to classes in the same package. This is the default accessibility when no access modifier is provided. The following shows a method defined with package-private access.
void doSomething() {
}
Protected
Members marked with protected can be accessed within the same package (like package-private) and can also be accessed in subclasses, regardless of the package. The following shows a method defined with protected access.
protected void doSomething() {
}
Private
Members marked with private are only accessible inside the same class. The following shows a method defined with private access.
private void doSomething() {
}
Java Classes
Java classes also have access modifiers, but they can only use public and package-private accessibility levels.
Conclusion
Java supports four different access modifiers. Access modifiers are used on class members, although you can use public and package-private at the class level. Access modifiers define who can access the class members.