Java Break Statement
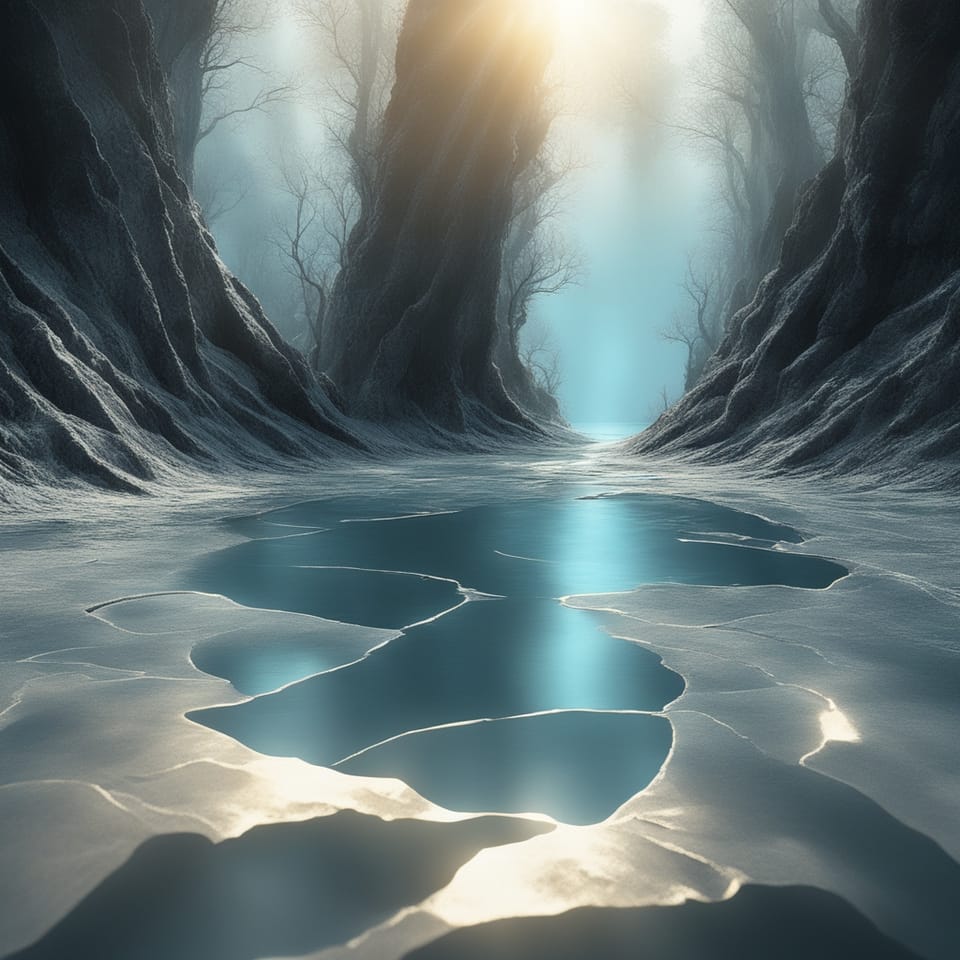
What Are Break Statements?
Break statements are statements used inside of loops. They allow you to terminate a loop regardless of what iteration you are on. This is separate from the condition the loop evaluates. One use case for a break statement is if you are waiting for an operation to complete. If it doesn't complete in a certain amount of time, you could break out of the loop. Break statements can be used in any loop statement.
Syntax
The syntax for a break statement is simple.
break;
An example of using a break statement would be the following:
while (!isCompleted()) {
// ...
if (isTimedOut()) {
break;
}
}
The while loop will terminate if the while condition is false, or if the isTimedOut() method returns true. If the isTimedOut() method returns true, it will execute the break statement in the if block, terminating the loop.
Conclusion
Break statements allow you to terminate a loop outside of the condition the loop is evaluating. If you find yourself in a case where you need to use a break statement, try to find a better approach. Break statements add complexity to code.