Java Class Layout Standard
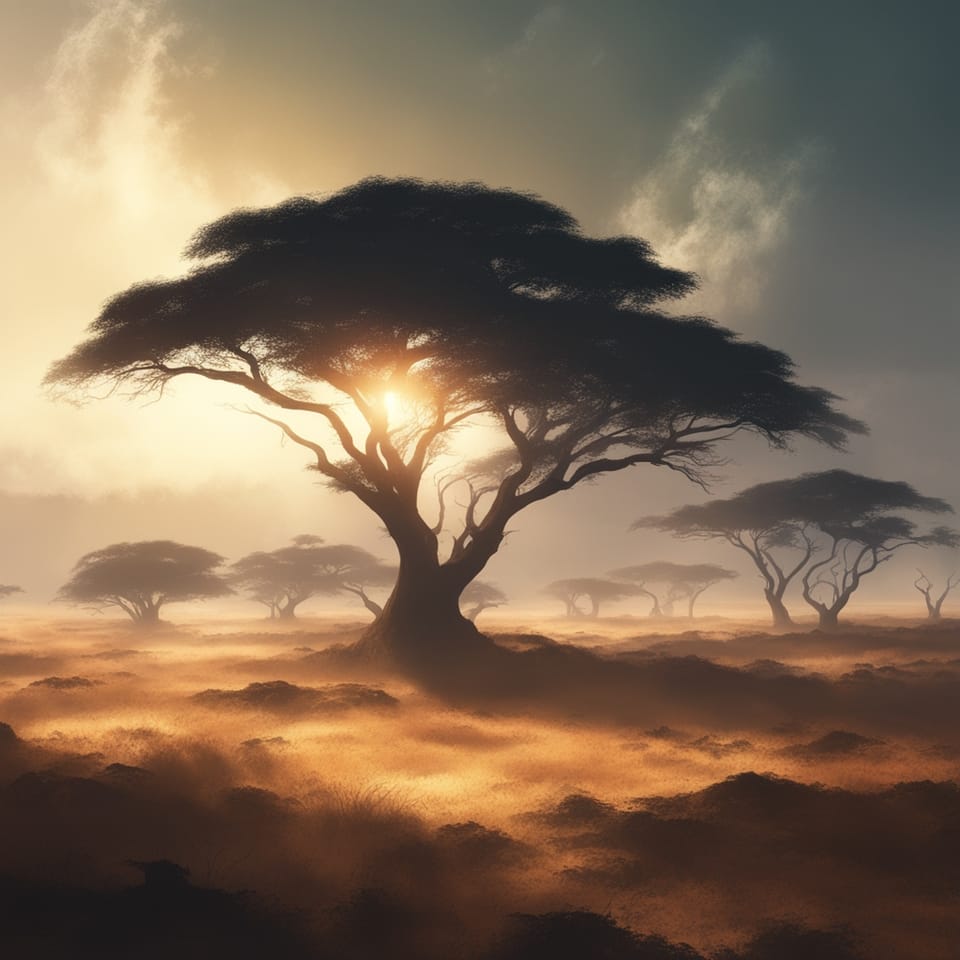
Standardized Class Layout
In Java, you can actually put methods, variables, constructors, etc. in any order in a class. Although you can do this, there is a standard way of where each of these things should be placed.
Variables
Variables should always be declared at the top of a class. There are three different types of variables a class can have.
Constants
Constants are the first type of variable that is listed in a class. Constants are variables marked with both static and final keywords.
public class User {
// Constants
public static final String ADMIN_USER_ID = "admin";
}
Class Variables
Class variables are the second type of variables in a class. Class variables are static variables.
public class User {
// Class Variables
public static int counter = 0;
}
Instance Variables
Instance variables come last in the list of variables. Instance variables are variables created for each instance of an object. Generally, instance variables will be marked as private.
public class User {
// Instance variables
private String firstName;
private String lastName;
}
Object Initialization
Object initialization is placed in a class after variables. Object initialization can be done in either static initializers, instance initializers, or constructors.
Static Initializer
Static is the first thing to be listed in object initialization.
public class User {
// ...
// Static initializer
static {
// ...
}
}
Instance Initializer
Instance initializers should come after each of the static initializers and before constructors.
public class User {
// ...
// Instance initializer
{
// ...
}
}
Constructors
Constructors should come after instance initializers. The first constructor listed should be the main constructor, where all of the work is done. Each overloaded constructor should come after the main constructor and always calls the main constructor with the appropriate parameters.
public class User {
// ...
// Main constructor
public User(
final String s1,
final String s2,
final String s3) {
// ...
}
public User(final String s1, final String s2) {
this(s1, s2, null);
}
public User(final String s1) {
this(s1, null, null);
}
public User() {
this(null, null, null);
}
}
Methods
Methods come in three different forms, static methods, instance methods, and abstract methods.
Static Factory Methods
Static factory methods are methods that are used to obtain an instance of a class. These should come after constructors.
public class User {
// ...
// Static factory method
public static User instance() {
// ...
}
}
Methods
Instance methods come after static factory methods. Classes should have very few to no static methods. They can be mixed and matched with instance methods where it makes sense.
public class User {
// ....
public void static staticMethod1() {
// ...
}
public void method1() {
// ...
}
public void method2() {
// ...
}
}
Abstract Methods
Abstract methods should come at the end of all of the methods. They are the least relevant and least likely to change.
public abstract class User {
// ...
public abstract void method1();
public abstract void method2();
}
Inner Classes
Inner classes should come at the very end of the file. Inner classes are kind of ugly, so it is good to keep them at the end.
public clas User {
// ...
public class InnerClass {
}
}
Full Class Example
The following shows a class with each of the pieces listed above and where you'd place them.
public abstract class AbstractUser {
//
// Variable section
//
// Constants
public static final String ADMIN_USER_ID = "admin";
public static final String DEFAULT_USER_ID = "default";
// Class Variables
public static int counter = 0;
public static int lastId = 10;
// Instance variables
private String firstName;
private String lastName;
//
// Initialization section
//
// Static initializer
static {
// ...
}
// Instance initializer
{
// ...
}
// Main constructor
public User(final String firstName, final String lastName) {
// ...
}
// Default constructor
public User() {
this(null, null)
}
//
// Method section
//
// Static factory method
public static AbstractUser instance() {
// ...
}
public static void staticMethod1() {
// ...
}
public void method1() {
// ...
}
public void method2() {
// ...
}
// abstract methods
public abstract void method3();
public abstract void method4();
//
// Inner or nested classes
//
// Inner class
public class MockUser {
// ...
}
}
Conclusion
It is important to have a standardized layout for anything across your team. It makes the code more uniform and easier to read. Stick to these standards for each of your Java classes.