Java Continue Statement
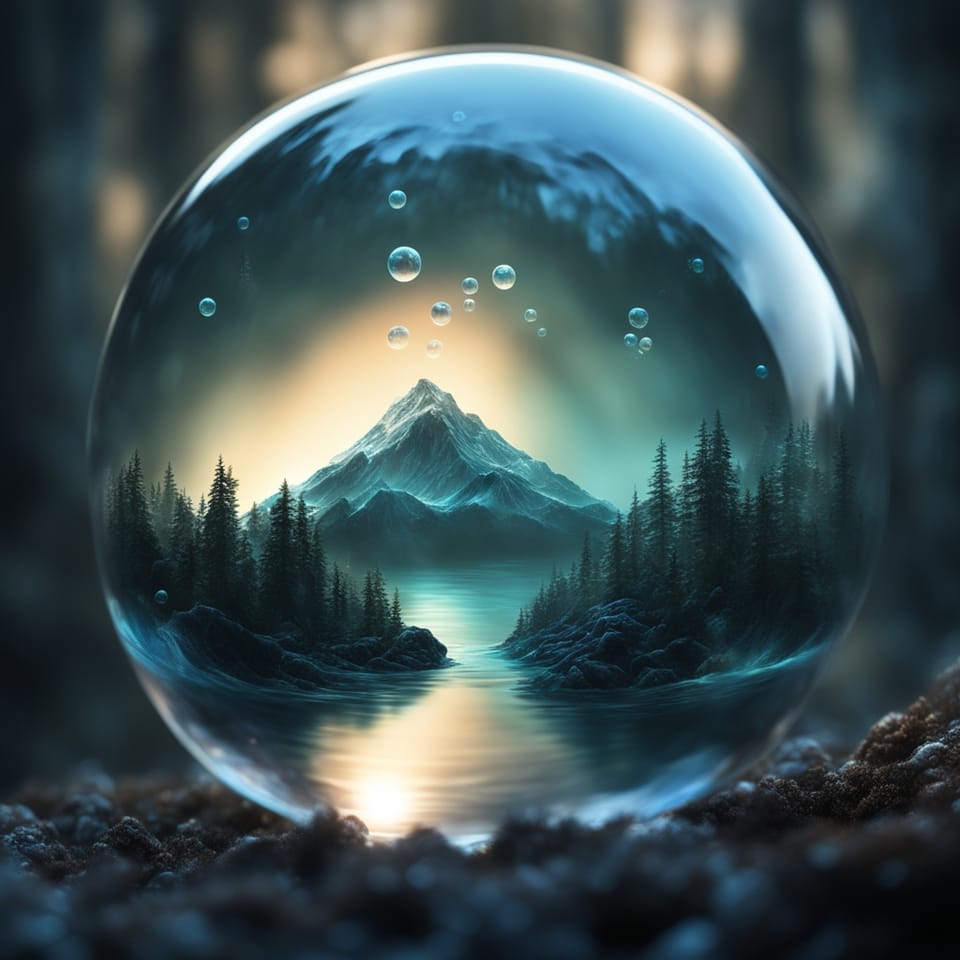
What Are Continue Statements?
Continue statements allow you to skip to the next iteration in a loop. When a continue statement is encountered, it stops the execution of the current loop's iteration and starts the next one. Continue statements can be used in any type of loop and anywhere in the loop body.
Syntax
Continue statements are simple and are written with the following:
continue;
An example of using a continue statement would be the following:
final var numbers = List.of(
1,
2,
3,
4,
5,
6,
7,
8,
9,
10
);
for (final var number : numbers) {
if (number % 2 == 0) {
continue;
}
System.out.println(number);
}
In the previous example, if number is an even number, it stops executing the iteration it is on and goes to the next iteration. If it is an odd number, it prints out the value of the number variable. The previous example would output the following:
1
3
5
7
9
Conclusion
Continue statements allow you to skip an iteration of a loop. Developers are used to if statements. If you find yourself needing to use a continue statement, a similar approach might be to use an if statement instead of a continue statement to get the same behavior.