Java Do-While Loop
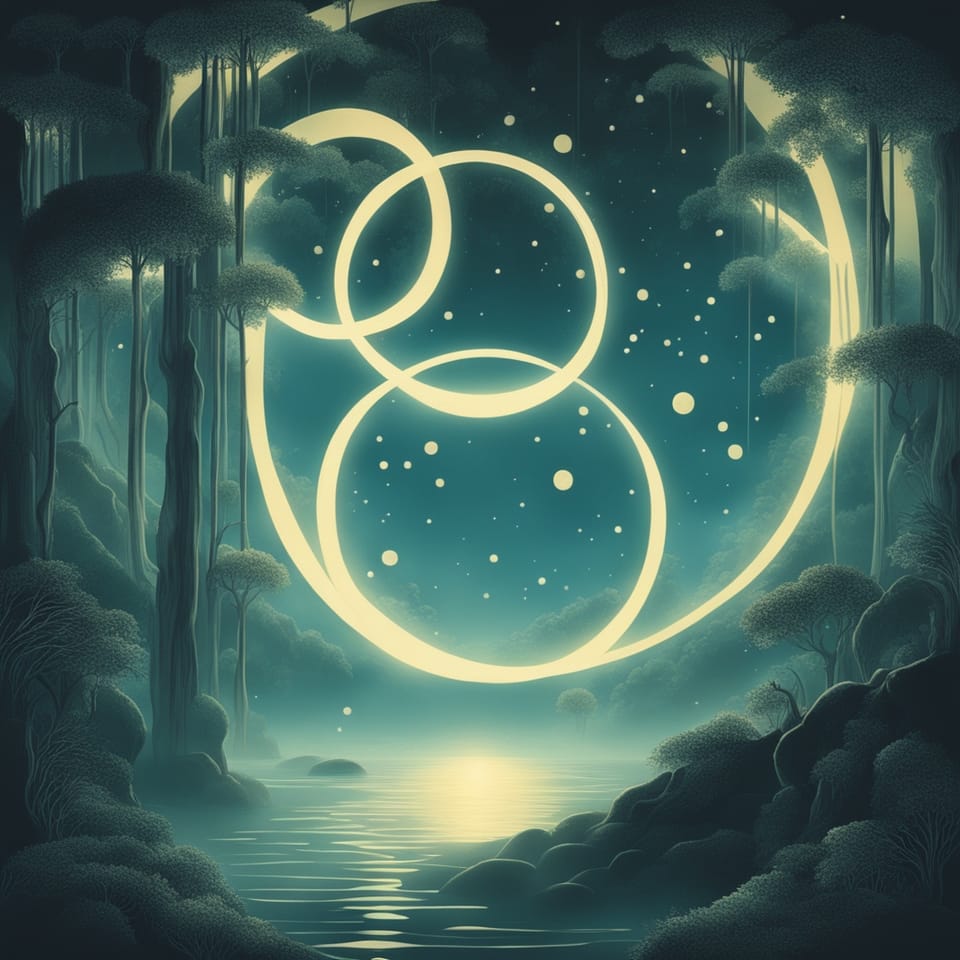
What Is a Do-While Loop?
A do-while loop is a loop that always executes at least one iteration, regardless of the condition. Once the first iteration executes, it then works the same as a while loop. It will continue to execute while a condition is true. Once the condition is false, it stops executing the loop.
Do-while loops only have a condition. You will need to update the variables for the condition inside the do-while loop to avoid an infinite loop.
Syntax
A simple do-while loop can be written with the following:
var index = 0;
do {
System.out.println("index = " + index);
index++;
} while (index != 5);
This first executes the block inside the do-while loop. It then checks if the condition is true. If it is, it repeats this process. If the condition is false, it stops executing the do-while loop. The previous example will output the following:
index = 0
index = 1
index = 2
index = 3
index = 4
Do-while loops are good to use when you have an unknown number of iterations but need to always execute at least one of those iterations. For example, the following code generates random numbers until it "guesses" the correct number.
final var max = 10;
final var random = new Random();
final var numberToGuess = random.nextInt(max);
var guess = 0;
var total = 0;
do {
System.out.println("Guess #" + total);
total++;
guess = randomNumber(max);
} while (numberToGuess != guess)
System.out.println("Answer = " + numberToGuess);
Conclusion
Do-while loops are the same as while loops except they always execute at least one iteration. Do-while loops are the least commonly used loop.