Java For-Each Loop
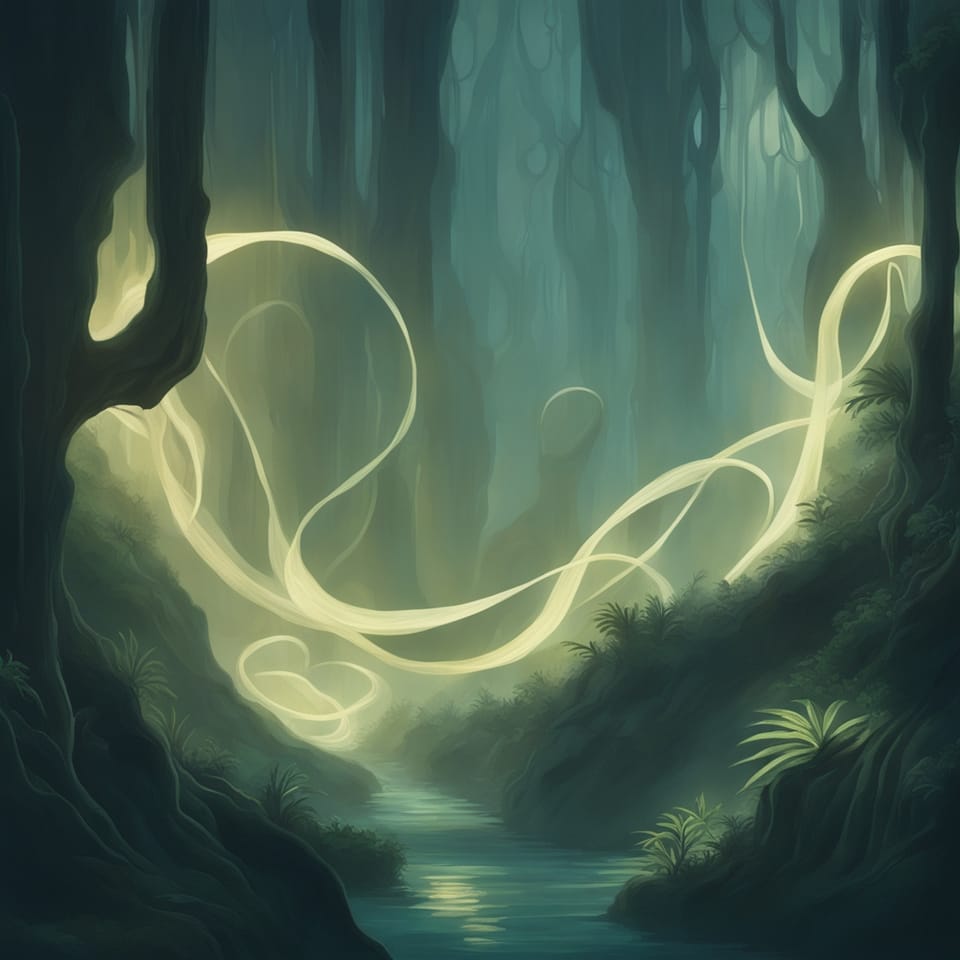
What Is a For-Each Loop?
For-each loops introduced in Java 5 allow for a simpler way to iterate over collections than a for loop. Instead of using a for loop and accessing the items in a collection or array by index, for-each loops execute a block of code for each item in a collection. For-each loops eliminate off-by-one errors. Arrays and classes that implement the java.util.Iterable interface can be used in for-each loops.
Syntax
For-each loops are written with the following syntax:
final var metals = List.of(
"Copper",
"Gold",
"Silver",
"Nickle"
);
for (final var name : metals) {
System.out.println(name);
}
The name variable is assigned each iteration of the loop. The order will go off of the ordering of the collection you are using.
For-each loops are best any time you are dealing with a collection or an array. They eliminate the complexity of for loops and indexes when they aren't needed. The previous example produces the following output:
Copper
Gold
Silver
Nickle
A for-each loop is equivalent to for loops that use iterators.
for (final Iterator<String> i = names.iterator(); i.hasNext();) {
// ...
}
Conclusion
If you have a collection or array you need to execute a block of code against, use a for-each loop instead of a for loop. For-each loops eliminate off-by-one errors.