Java If Statement
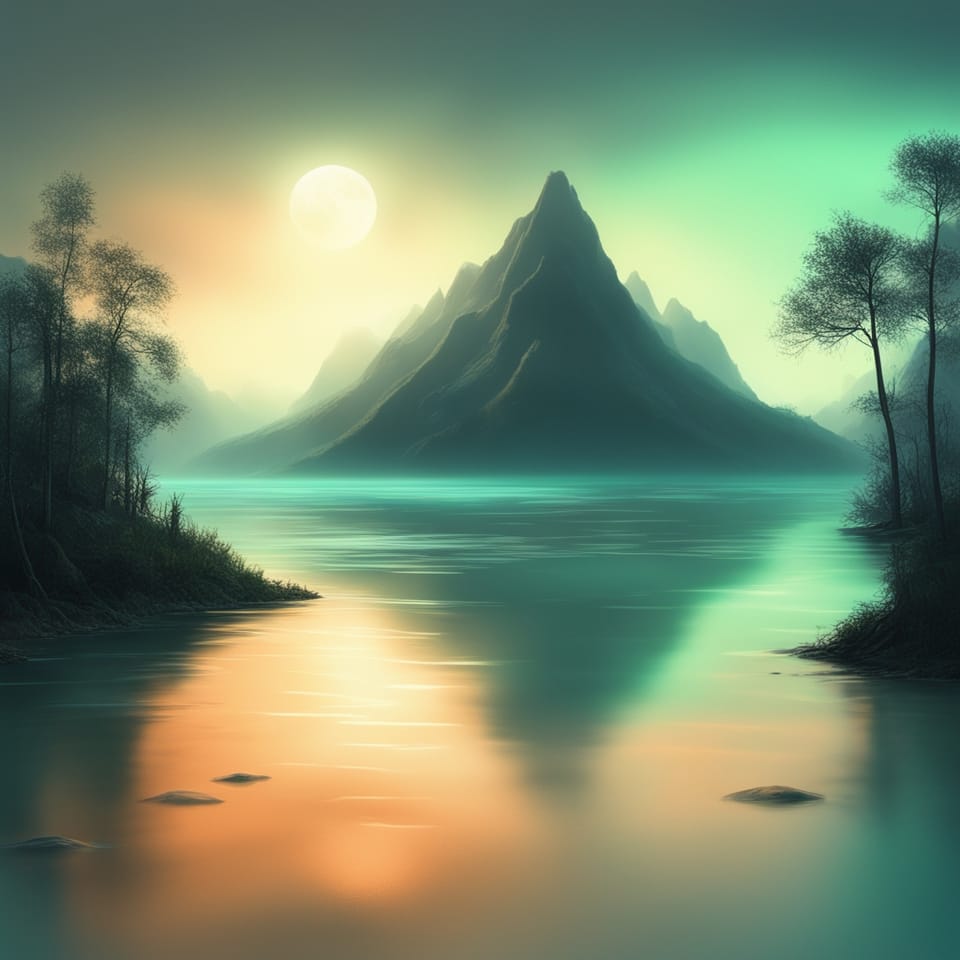
What is an If Statement?
If statements are what allow you to make decisions in your code based off of a condition. If the condition evaluates to true, the code inside of the if block will execute. If the condition evaluates to false, it will not execute the if block.
How to Write an If Statement?
Java's if statements have the following basic structure.
if (condition) {
// code
}
If an if statement has one line of code, you can omit the braces. Although you can do this, it is still better to always use braces for consistency.
Multiple Conditions
If statements can test for multiple conditions. To do this, you use an else if.
if (condition1) {
// ...
} else if (condition2) {
// ...
} else if (condition3) {
// ...
}
This will check each condition in the order listed until one condition evaluates to true. Once a condition evaluates to true, it will execute that if block's code and then break out of the if block all together.
No Matches
If you want to execute a block of code in an if statement when no condition evaluate to true, use else.
if (condition) {
// ...
} else {
// ...
}
If statements that contain an else will always execute one of the blocks of code in the if statement.
If statements always start with an if. After that, they will be followed by zero or more else if statements, and then with an optional else clause.
Relational Operators
If statements can have multiple expressions that evaluate a condition. Each expression evaluates either to true or false. These expressions then combine together to form a condition of either true or false.
&& (and)
If you have multiple expressions and want to execute the block of code if both conditions are true, you use the and operator, which is written with &&.
if (a && b) {
// ...
}
Both a and b must be true for the block of code inside the if to execute.
|| (or)
If you have multiple expressions and want to execute the block of code if only one of them is true, you use the or operator, which is written with ||.
if (a || b) {
// ...
}
Only a or b has to be true for the if block to execute.
! (not)
Conditions in if statements can be reversed using the not operator at the beginning of the condition. This is written with the ! character.
if (!isLoggedIn) {
throw new LoginRequiredException();
}
If isLoggedIn is false, the code block will execute since the value is reversed.
Logical Operators
Expressions can be more than just variables in an if statement. You can compare if something is equal to, less than, or greater than.
> (greater than)
You can check if a number is greater than another value using the greater than operator >.
if (x > 0) {
// ...
}
This will execute the if block if x is greater than 0.
< (less than)
You can check if a number is less than another value using the less than operator <.
if (x < 0) {
// ...
}
This will execute the if block if x is less than 0.
== (equal to)
You can check if a number is equal to a value or an object instance is the same using the == operator.
if (x == 0) {
// ...
}
This will execute the if block if x is equal to 0.
In Java, if you are checking if two objects are equal, you need to use the equals() method and understand the difference between == and equals().
Instanceof Operator
Java has the instanceof operator for checking to see if an object is an instance of a specific class or interface. This is common when working with polymorphism, and you need to know the type you are working with.
final var metal = new Gold();
if (metal instanceof Silver) {
final var silver = (Silver) metal;
// Do something...
} else if (metal instanceof Gold) {
final var gold = (Gold) metal;
// Do something...
}
In this example, since metal is an instance of the Gold class, the else if block of code will execute.
In Java 14, the instanceof operator was enhanced to allow you to also be able to declare a variable. The above code could be simplified to the following:
final var metal = new Gold();
if (metal instanceof Silver silver) {
// Do something...
} else if (metal instanceof Gold gold) {
// Do something...
}
The variables declared with instanceof are only created if that if block evaluates to true, and the variable declared is only available while inside that if block.
You can also use the variable in the condition after it is declared.
final var metal = new Gold();
if (metal instanceof final Silver silver && silver.isCoin()) {
// Do something...
} else if (metal instanceof final Gold gold && gold.isCoin()) {
// Do something...
}
Conclusion
If statements are what you use to conditionally execute different blocks of code. You can combine multiple expressions in each condition and have multiple conditions for different code execution paths.