Java Import Statement
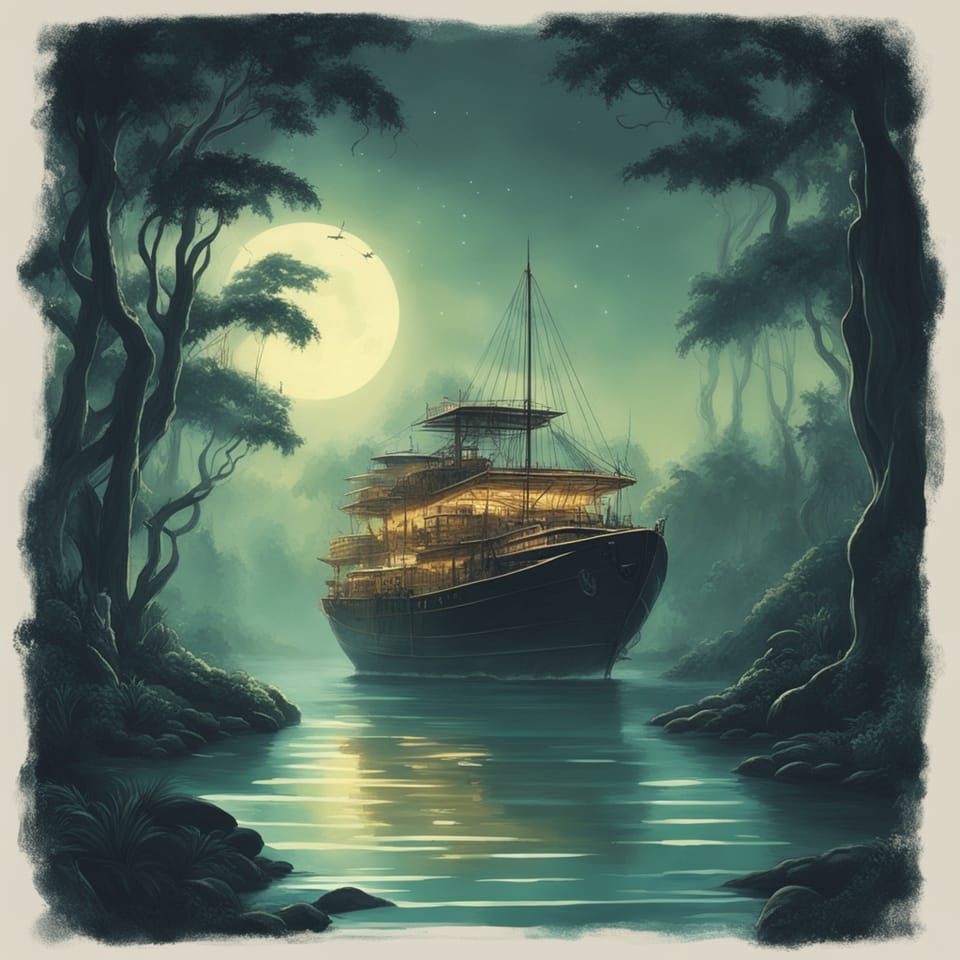
Classes in Other Packages
When you want to use a class that exists outside of the package you are working in, you can do one of two things. The first is to import the class using an import statement. Import statements come after a package declaration and before the class declaration in a Java file. The second is to use the fully qualified name of the class.
Fully Qualified Name
This is the package, followed by a period and the class name. Consider you want to use the following class:
package press.bytesize.examples;
public class Example {
// ...
}
Using the fully qualified name, this could be used outside of the press.bytesize.examples package with the following:
final var example =
new press.bytesize.examples.Example();
Using the fully qualified name is usually only done if you have two different classes with the same name used in the same file.
Importing a Class
Classes that exist outside of the package you are working in can be imported. This allows you to use a class by its name instead of the fully qualified name. The class in the previous example can be imported with the following:
package press.bytesize.application;
import press.bytesize.examples.Example;
public class App {
private final Example example = new Example();
}
Since an import statement has been provided for the Example class, the class can be used as though it were in the same package.
Importing a Package
You can import all of the classes in a package using the * wildcard. If you wanted to import all the classes in the press.bytesize.examples package, this can be done with the following:
import press.bytesize.examples.*;
Be cautious when using this. You can introduce naming conflicts by importing classes that are not used. If you run into this, add an import statement for each individual class you are using in that package and remove the wildcard * import.
Importing a Static Method
You can import individual static methods into a class. This allows you to use a static method as though it were defined inside of that class. For example, the StaticImportExample class has a static method called sayHello().
package press.bytesize.examples;
public class StaticImportExample {
public static void sayHello() {
// ...
}
}
You can import this static method using a static import.
import static press.bytesize.examples.StaticImportExample.sayHello;
This is common in unit tests when importing methods such as assertEquals() or assertTrue().
You can import all of the methods using * wildcard as well.
import static press.bytesize.examples.Example.*;
Conclusion
Imports allow you to use a class that exists outside of the package you are in by just its class name. This makes the code shorter and more readable. Only use a fully qualified class name when you have two classes with the same name in a file.