Java Interfaces
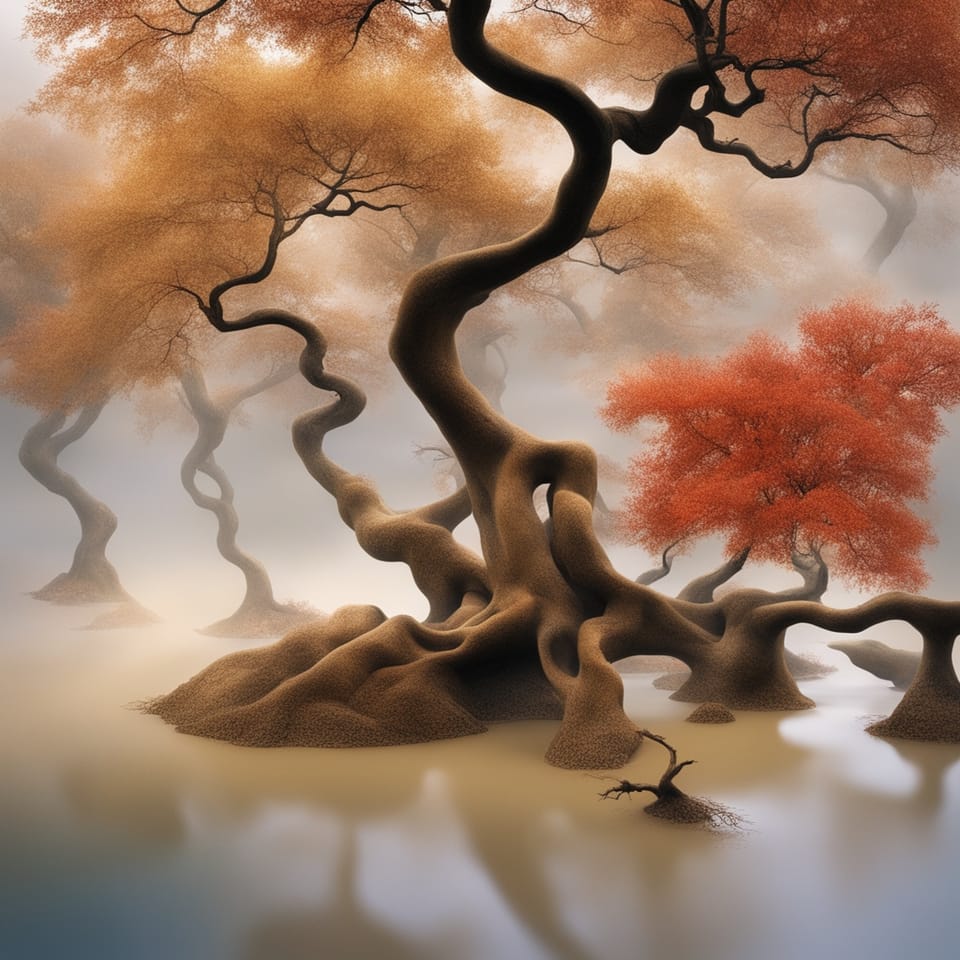
Interfaces
Interfaces are used to define a public contract that a class must implement. Interfaces are reference types, just like a class. Interfaces only define method signatures for a class to implement. They are different from abstract classes because they do not provide instance variables, constructors, or method implementations.
Interfaces are defined similar to classes but using the interface keyword instead of the class keyword.
package press.bytesize;
public interface MyInterface {
// ...
}
The Java source file must match the interface name, followed by a .java file extension.
Interfaces follow the same standard as classes and are more commonly named using adjectives. Although not required, it is common for interfaces in Java to have a suffix of able.
Methods
Methods in an interface are only the method signature and end with a semicolon. Methods defined in interfaces are implicitly public abstract, so providing these keywords on a method signature is redundant and unnecessary. An interface with methods would look like the following:
public interface Example {
String id();
void method(
final String parameter1,
final String parameter2);
}
Implementing an Interface
To implement an interface on a class, you use an implements clause. This comes after an extends clause on a class if the class has one. When implementing methods of an interface, they are annotated with the @Override annotation. Implementing the previous example in a class would look something like the following:
public class MyClass implements Example {
@Override
public String id() {
// ...
}
@Override
public void method(
final String parameter1,
final String parameter2) {
// ...
}
}
When implementing an interface, the method parameter names do not have to match what is in the interface, only the types. Both parameter1 and parameter2 could be renamed to anything else.
Extending an Interface
Just like classes can extend other classes, interfaces can extend other interfaces. Interfaces can extend any number of interfaces. Since you are extending an interface, not implementing it, you use the extends keyword.
public interface Tiger extends Cat, Animal {
// ...
}
You would then have to implement the methods on the Cat, Animal, and Tiger interfaces on whatever class implements Tiger.
Conclusion
Interfaces offer a level of abstraction by not providing an implementation but a contract that an implementation must follow. They are constants and method signatures that another class provides an implementation for.