Java Naming Conventions
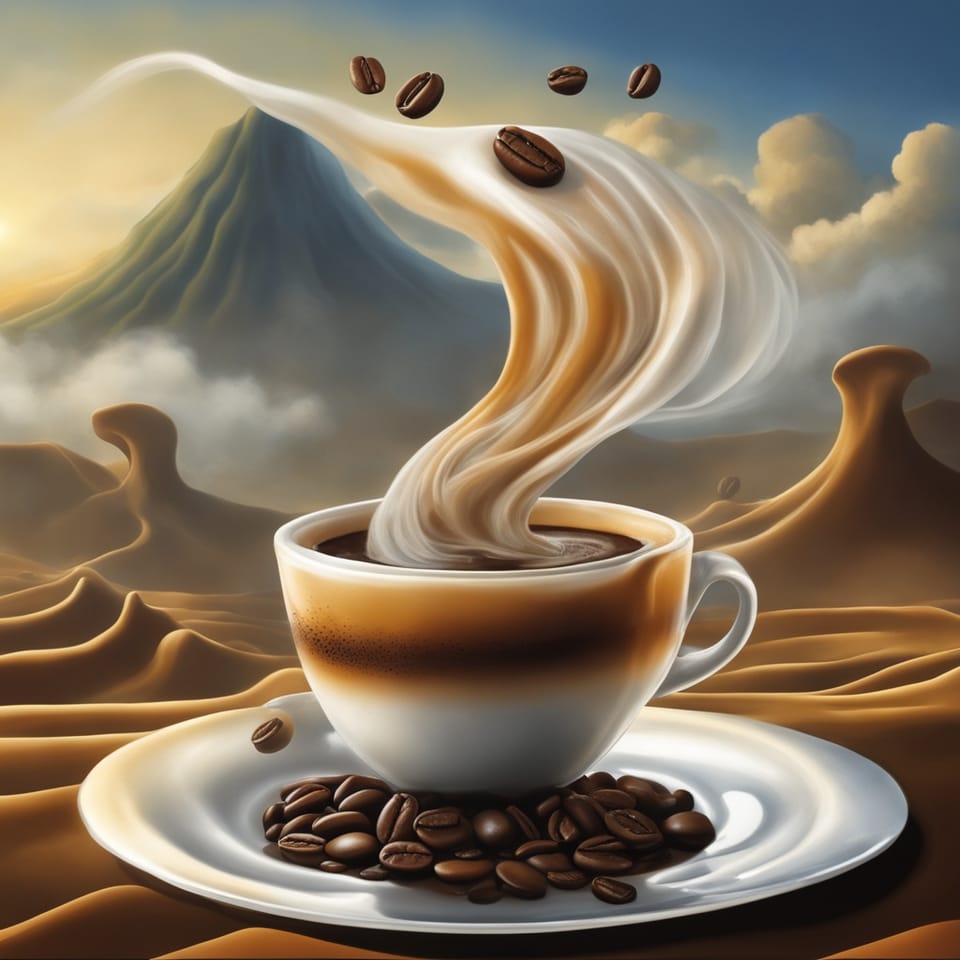
Java Source Files
Java source files are named with the same name of the class defined in the source file and end with a .java file extension.
User.java
Packages
Package names are written in all-lowercase ASCII letters. Package names start with a company's internet domain name reversed. They can be singular or plural and should be a single word.
package press.bytesize.domain;
package press.bytesize.daos;
Classes
Class names should be a noun and are written in pascal case. Class names should be whole words and avoid abbreviations and acronyms unless they are more widely used. Class names should be singular not plural.
public class User {}
public class Animal {}
public class HtmlParser {}
Interfaces
Interfaces follow the same standard as classes and are more commonly named using adjectives. Although not required, it is common for interfaces in Java to have a suffix of able.
public interface Closeable {}
public interface JsonAware {}
Exceptions
Exceptions are written in pascal case and have a suffix of Exception.
public class AuthenticationException {}
public class UserNotFoundException {}
Annotations
Annotation names are written using pascal case and are named using a verb, noun, or adjective depending on what meta data it is describing.
public @interface Deprecated {}
public @interface Override {}
public @interface Secure {}
Methods
Method names are written using camel case and start with a verb. Methods that return a boolean are prefixed with is.
public void run();
public String parse();
public boolean isEnabled()
public boolean isAuthenticated();
Variables
Instance variables, method variables, and local variable names are all written using camel case. Variables start with a letter and should be meaningful based on their intent and usage. Variables should never be a single character, with the exception of a counter in a for loop or an exception in a catch block.
final var toDelete = new User();
final var greeting = "Hello";
Constants
Constant variable names are written using screaming snake case.
public static final int HEADER_INDEX = 0;
public static final String HOME_URL = "www.bytesize.press";
Enumerations
Enumerations follow the same naming as classes. Enumeration constant names are written using screaming snake case.
public enum Color {
RED,
YELLOW,
GREEN;
}
Conclusion
Code is read and maintained more than it is written. Coding conventions help with readability and standardize the code based both on the language and the project. This makes it easier for others to maintain and enhance the code.