Java Packages
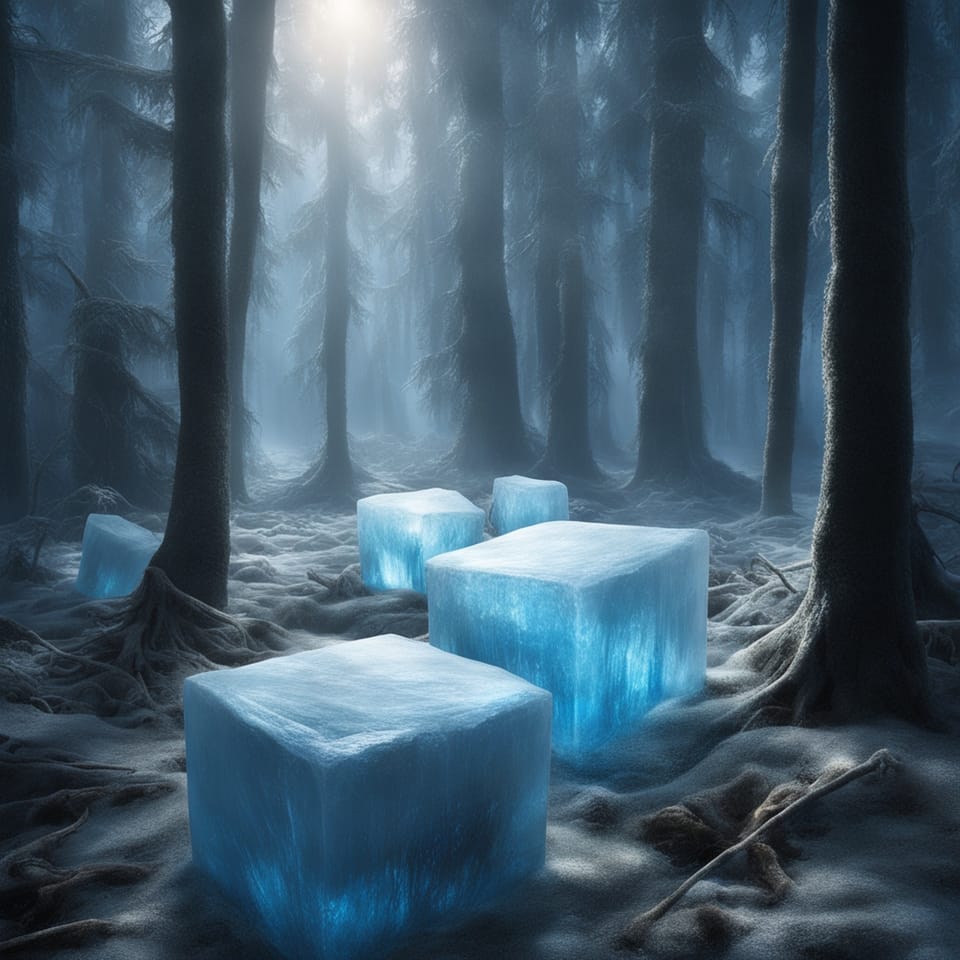
What Are Packages?
Packages are a way of organizing classes by grouping them together, similar to grouping files together in a directory. They allow you to control the accessibility of a class through an access modifier. They are also used to prevent naming conflicts if you have more than one class file with the same name in your project.
Defining a Package
A package is defined at the top of a Java file using the package keyword. Packages must be the first statement in a Java file.
package press.bytesize.example;
public class MyClass {
// ...
}
If your class files were located in a directory called myproject, the MyClass.java file would be located in the following directory.
myproject/press/bytesize/example/
The directory structure matches the package structure.
Naming Packages
Package names are written in all lowercase. Package names start with a company's internet domain name reversed. For example, www.bytesize.press would be written with the following:
package press.bytesize;
After this, you can create other packages in your project. For example:
press.bytesize.dao
press.bytesize.services
press.bytesize.domain.models
Each section in a package should be one word. If you have to use multiple words in a section, it is still written in all lowercase. Packages can be named either singular or plural.
Preventing Class Naming Conflicts
Class names inside of a package must be unique. A class name is actually prefixed with the package name. You can reuse the same class name in different packages because of this. This is referred to as an FQN (Fully Qualified Name). For example, the following classes FQN would be press.bytesize.example.MyClass.
package press.bytesize.example;
public class MyClass {
}
package-info.java File
Java 5 introduced the package-info.java file. Since a package is just a statement at the top of a class file, a package-info.java file allows you to declare a Javadoc and annotations for a package. To do this, create a file called package-info.java in the directory for that package, in this case, the package will be press.bytesize.examples. The contents of this class may look like the following:
/**
* This package contains examples used in this byte-size article.
/*
@MyAnnotation
package press.bytesize.examples;
import press.bytesize.annotations.MyAnnotation;
This also allows your IDE (Integrated Development Environment) to show this documentation when writing code.
Conclusion
Java packages allow you to group classes together and avoid naming conflicts. Package names start with your internet domain name reversed.