Java Primitive Data Types
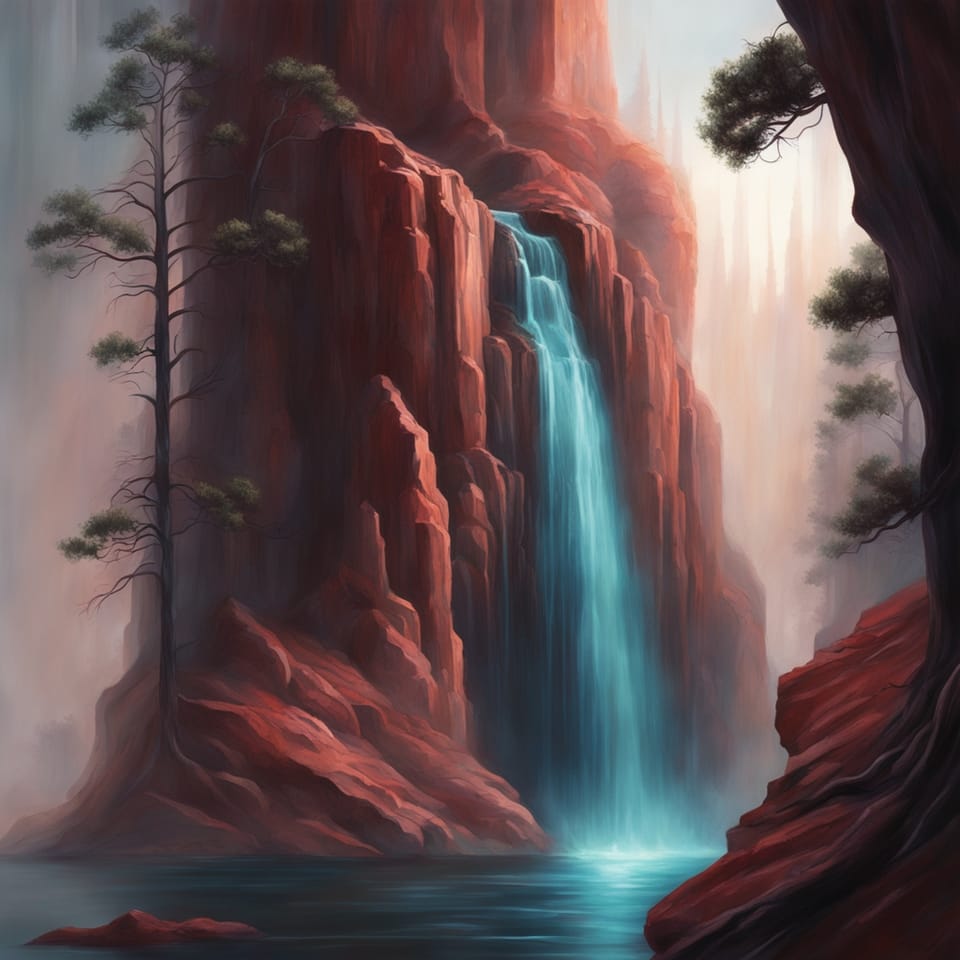
Different Primitive Data Types
Java has four different categories of primitive types: integers, floating-point numbers, booleans, and characters.
All number types can be boiled down to either integers or floating-point numbers. The difference between these two is whether they have a decimal. Integers are whole numbers. Floating-points are numbers that can contain a decimal. There are six different primitive types used for numbers. The difference between them is the size of the number they can hold.
Local primitive type variables that aren't assigned a value and then are used will result in a compiler error.
Booleans
Booleans are represented by either true or false using the true or false keywords. If a boolean doesn't have a value assigned to it, the default value will be false.
final boolean isValid = true;
final boolean isEnabled = false;
Characters
Char is a single 16-bit Unicode character. The default value for a char is '\u0000' which represents the null character. This isn't the same value as null in Java.
A char value is surrounded by single quotes and defined with the char keyword.
final char a = 'A';
Unicode characters can be used as well and are prefixed with \u.
final char a = '\u0041';
Chars can support special escape sequences such as \r.
final char r = '\r';
Integer Types
Java has four different integer types, byte, short, int, and long. Typically, you are going to use an int when working with integer types. If you need something bigger than an int, you'll use a long. Bytes and shorts are used more when you need to save space. Primitive integer types have a default value of 0 if a value is not assigned to them.
byte
Bytes are 8-bit signed integers ranging from -128 to 127 (inclusive) and are defined using the byte keyword.
final byte number = 1;
short
Shorts are 16-bit signed integers ranging from -32,768 to 32,767 (inclusive) and are defined using the short keyword.
final short number = 1;
int
Integers are 32-bit signed integers ranging from -2,147,483,648 to 2,147,483,647 (inclusive) and are defined using the int keyword.
final int number = 1;
long
Longs are 64-bit signed integers ranging from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 (inclusive) and are defined using the long keyword.
final long number = 1;
With long literals, you would end them with an uppercase L.
// Returns number as long
(100L + 500L);
Floating-point Types
Java has two different floating-point types: float and double. Typically, you will be working with doubles unless you need to save space, then use a float. You shouldn't use floating-point types for precise values, such as currency. Floating-point types, if a value is not assigned to them, have the default value of 0.0.
float
Floats are a 32-bit single-precision type and are defined using the float keyword. The value must be written, ending with a lowercase f.
final float value = 3.2f;
double
Doubles are a 64-bit double-precision type and are defined using the double keyword.
final double value = 3.2;
Scientific Notation
Floating-point literals can be written using scientific notation.
final double number = 2.13e2;
Underscores in Number Values
Java 7 introduced allowing underscores in numbers. This is used to make numbers more readable, similar to commas in a number.
final int value = 1_923_673;
You can't put underscores at the beginning or end of the number or next to a decimal.
What About Strings?
Strings are not primitive types. Although they are not primitive types in Java, Java gives them special treatment, so it can seem as though they are primitive types at times.
Conclusion
Java has eight different primitive types categorized as booleans, characters, or integers, and floating-point numbers. Integer numbers usually will use int, and floating-point numbers will usually use double.