Java Static Initializers
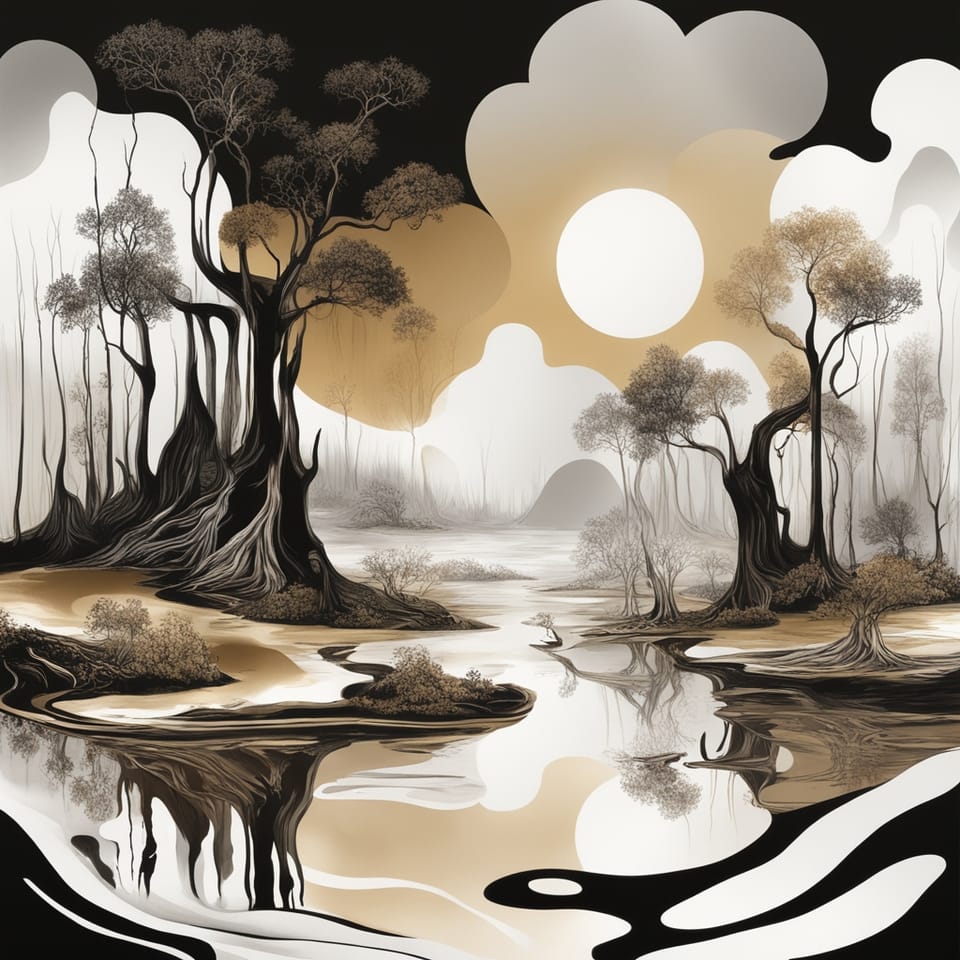
What are Static Initializers?
Static initializers, also referred to as static initialization blocks, are blocks that are used to assign a value to a class variable or constant. They are only called once and are guaranteed to be called before the class is used. Static initializers can be placed anywhere in a class, but it is good to place them after constants, class variables, and instance variables and before constructors. An example of a static initializer is the following:
public class Table {
// Constant
public static final int HEADER_INDEX;
// Class variable
private static int counter;
// static initializer
static {
HEADER_INDEX = 0;
counter = 1;
}
// ...
}
This is a simple example, and both variables could be assigned their value when they are declared. This example is just showing how to write a static initialization block and assigning values to a class variable and a constant. Static initialization blocks are used when it is more complex than a single line of code to assign a value to a class variable or constant.
A class can have any number of static initialization blocks. They are executed from top to bottom in the class in the order they are defined.
public class Table {
public static final int HEADER_INDEX;
private static int counter;
// Executes first
static {
HEADER_INDEX = 0;
}
// Executes second
static {
counter = 1;
}
// ...
}
When do Static Initializers Execute?
A static initializer will execute at different times depending on a few different conditions. A static initializer in a class that contains a main() method will execute before the main method.
public class App {
static {
System.out.println("static initializer");
}
public static void main(final String[] args) {
System.out.println("main");
}
}
Running this will produce the following output:
static initializer
main
If the class doesn't contain a main() method, it will execute the first time the class is accessed. This can happen one of two ways. A static initializer will execute the first time a class instance is created.
public class Example {
static {
System.out.println("Example static initializer");
}
// ...
}
public class App {
public static void main(final String[] args) {
System.out.println("main");
new Example();
}
}
Running this will produce the following output:
main
Example static initializer
A static initializer will also execute the first time a class variable or constant is accessed. It works the same for either variable type, so in the following example only a class variable is shown.
public class Example {
public static int counter;
static {
counter = 1;
System.out.println("Example static initializer");
}
// ...
}
public class App {
public static void main(final String[] args) {
System.out.println("main");
System.out.println(Example.counter);
}
}
Running this will produce the following output:
main
Example static initializer
1
There are three different times a static initializer could be called. A static initializer will only execute once. Once one of these three things happens, it will never be executed again.
Conclusion
Static initializers are guaranteed to execute before a class variable or constant is used. They are only executed once and are a way to assign class variables and constant values when the code is more complex than a single line.