Java Switch Expression
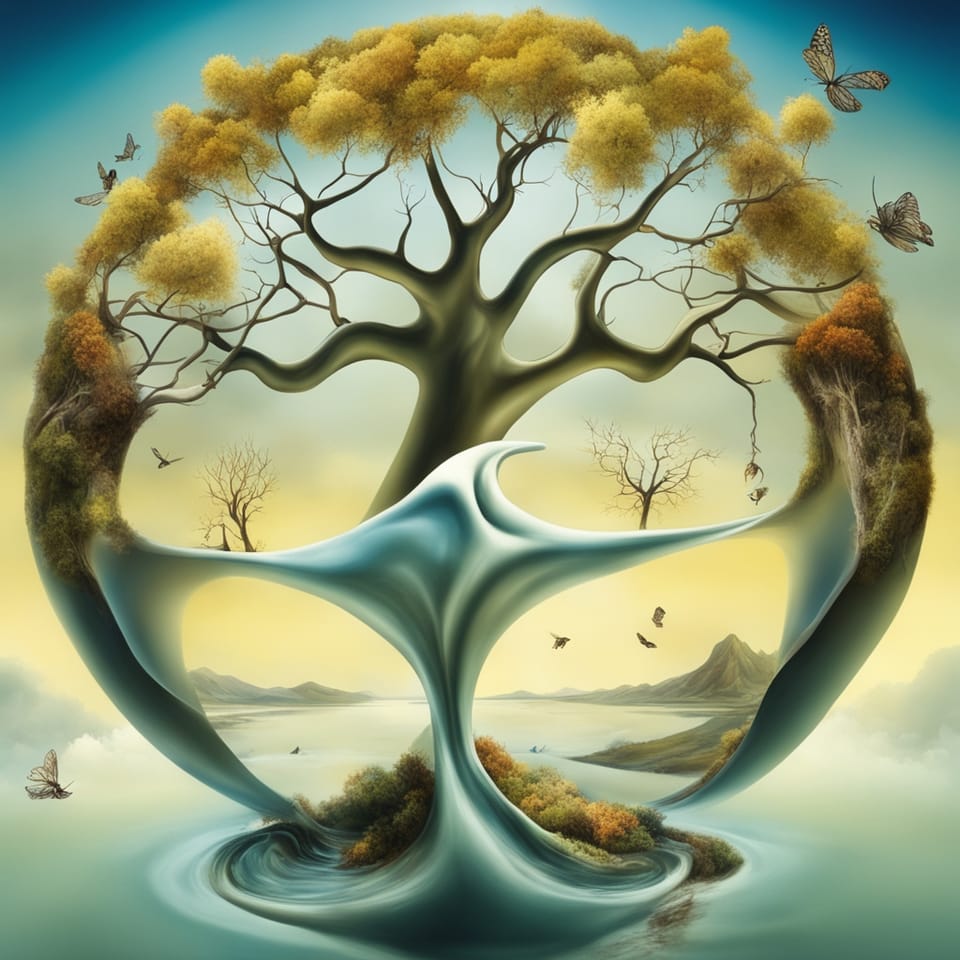
Switch Expressions
Java 17 introduced the ability to write switch expressions. Switch expressions can be used with any constant value that can be used in a switch statement. Switch expressions support char, byte, short, int, Character, Byte, Short, Integer, enum, and String.
A switch expression is similar to a switch statement but is simpler and safer to use. Switch expressions match one or more values and execute a block of code if it matches. They are written using -> instead of a : after the label section.
Looking at the following switch statement:
String text;
switch (color) {
case GREEN:
case YELLOW:
text = "go";
break;
case RED:
text = "stop";
break;
default:
throw new RuntimeException("Unknow value: " + color);
}
This switch statement can be written using a switch expression with the following:
final String text = switch (color) {
case GREEN, YELLOW -> "go";
case RED -> "stop";
default -> throw new RuntimeException("Unknow value: " + color);
}
Switch expressions allow for immutable variables, where using a switch statement, the variable has to be mutable as shown in the previous examples.
Switch expressions do not support fall though, like a switch statement. Because of this, break statements are not used in a switch expression.
Default Clause
A switch expression must have a default clause. Omitting a default clause will result in a compiler error.
final int number = 1;
// Compiler error:
// the switch expression does not cover all possible input values
final String value = switch(number) {
case 1 -> "one";
case 2 -> "two";
};
To fix this, simply add a default clause to the switch expression.
final int number = 1;
final String value = switch(number) {
case 1 -> "one";
case 2 -> "two";
default -> "everything else";
};
Enumerations
Enumerations used in a switch expression may or may not have a default clause. If a switch expression covers all enum constants, the compiler will insert an implicit default clause so a developer doesn't have to provide one.
public enum Color {
RED,
GREEN;
}
final Color color = Color.RED;
final String text = switch (color) {
case RED -> "stop";
case GREEN -> "go";
};
If you were to add another color to the Color enumeration, the previous switch expression will now have a compiler error.
public enum Color {
RED,
YELLOW,
GREEN;
}
final Color color = Color.RED;
// Compiler error:
// the switch expression does not cover all possible input values
final String text = switch (color) {
case RED -> "stop";
case GREEN -> "go";
};
Yield Statements
Switch expressions introduce the yield keyword. Yield statements act similar to a return statement but for a specific case label. Yield statements take a single value and are what value a specific case label yields. Yield statements are only needed if the code block is more than one line of code. If you are working with multiple lines, you need to introduce braces and end it with a yield statement.
final String text = switch (color) {
case YELLOW, GREEN -> {
System.out.println(color);
yield "go";
}
case RED -> "stop";
};
Using Switch Expressions as Statements
Switch expressions do not have to yield a value. They can be used to execute different code blocks without yielding a value, making them so you can use them like a statement.
switch (color) {
case GREEN, YELLOW -> {
System.out.println("Executing Go");
executeGo();
}
case RED -> executeStop();
default -> throw new RuntimeException("Unknow value: " + color);
}
Using a switch expression as a statement, the compiler will not enforce a default clause. Although this is the case, it is best practice to always provide a default clause.
Conclusion
Switch expressions offer a better, less error-prone approach than a switch statement. They should be used instead of switch statements. Switch statements should be used more in legacy code where switch expressions are not supported. Switch expressions can also be used to replace some if statements where you are simply mapping a constant to a value or block of code.