Java Switch Statement
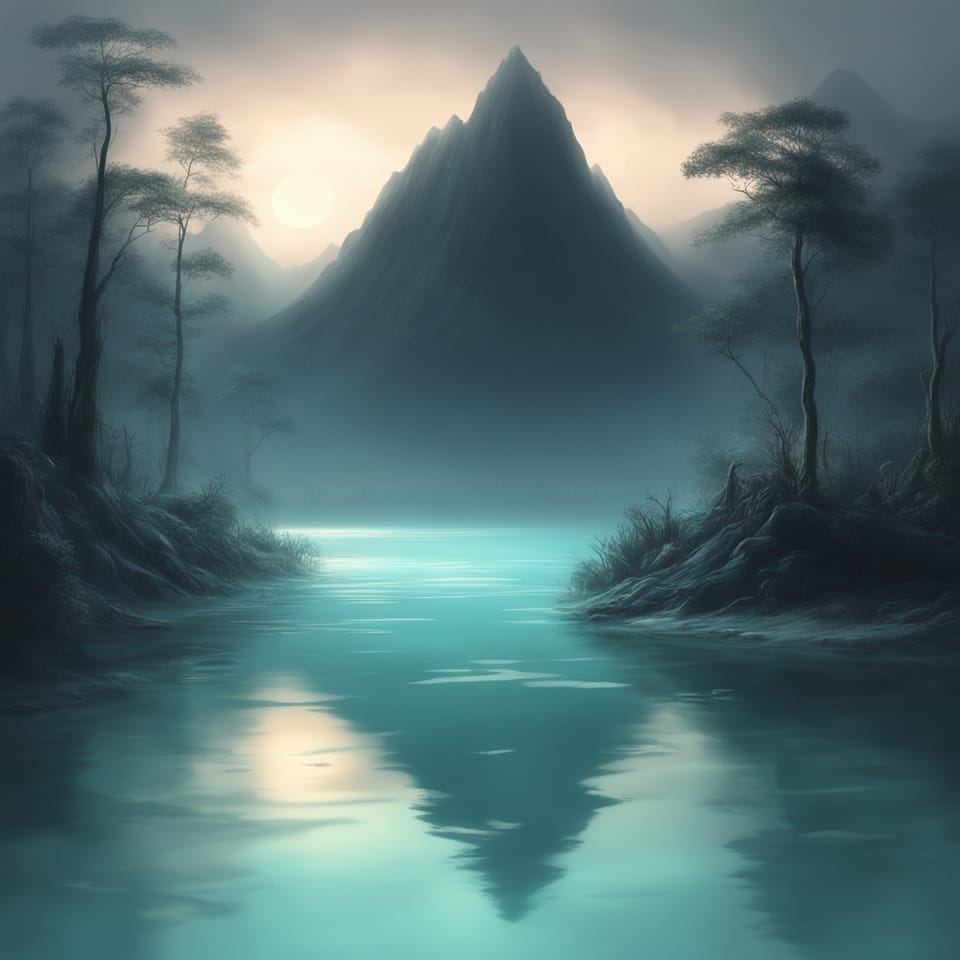
What are Switch Statements?
Switch statements evaluate an expression and then match the result based on a label. The body of a switch statement is called a switch block. Switch statements support char, byte, short, int, Character, Byte, Short, Integer, enum, and, as of Java 7, they also support String.
Switch statements are written with the following syntax:
final int dayOfTheWeek = 6;
String result = "";
switch (dayOfTheWeek) {
case 1:
result = "Sunday";
break;
case 2:
result = "Monday";
break;
case 3:
result = "Tuesday";
break;
case 4:
result = "Wednesday";
break;
case 5:
result = "Thursday";
break;
case 6:
result = "Friday";
break;
case 7:
result = "Saturday";
break;
}
// Outputs: Friday
System.out.println(result);
A switch statement starts with the expression to evaluate. In this case it is just an int variable. The body of a switch statement has one or more case labels. A case is defined for each day of the week. Whatever the expression evaluates to, it will check each case from top to bottom. In this example, it matches 6, so "Friday" will be the value assigned to the result variable.
Break Statements
Break statements are used to break out of the switch statement. Without a break statement, it will fall through to the next case. Taking the previous example and removing the break statements, "Saturday" will be the value assigned to the result variable.
final int dayOfTheWeek = 6;
String result;
switch (dayOfTheWeek) {
case 1:
result = "Sunday";
case 2:
result = "Monday";
case 3:
result = "Tuesday";
case 4:
result = "Wednesday";
case 5:
result = "Thursday";
case 6:
result = "Friday";
case 7:
result = "Saturday";
}
// Outputs: Saturday
System.out.println(result);
This will first match case 6 and assign the value "Friday" to the result variable. It then falls through to case 7 since there isn't a break statement and then reassigns the value "Saturday" to the result variable. If the example was changed to use the operator += in each case instead of the = operator, "FridaySaturday" would end up being the value assigned to the result variable.
When using switch statements, it is easy to introduce a bug by forgetting to add a break statement. Always make sure you add break statements unless you are wanting it to fall through.
Return Statements
Return statements can be used in switch statements to break out instead of using a break statement. Using the previous example and using an enumeration for the day of the week instead of an int, you could do the following for a method that returns if it is the weekend or not.
public enum DayOfTheWeek {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY;
}
public boolean isWeekend(final DayOfTheWeek dayOfTheWeek) {
switch (dayOfTheWeek) {
case SUNDAY:
case SATURDAY:
return true;
case MONDAY:
case TUESDAY:
case WEDNESDAY:
case THURSDAY:
case FRIDAY:
return false;
default:
throw new RuntimeException(
"Invalid value: " + dayOfTheWeek.name()
);
}
}
Notice that both SUNDAY and SATURDAY fall through to return true and the other values fall through to return false.
Default Clause
Default clauses are similar to an else in an if statement. Default clauses serve as a catch-all case for any value that doesn't match. They are optional and should be the last thing in a switch statement since switch statements execute from top to bottom.
It is good practice to have default clauses in switch statements. If you are writing a switch statement and have covered all options, for example, in an enumeration, your default clause should throw an exception. This way, when a new value is added, it can be added to all places.
Conclusion
Switch statements allow you to make one or more case labels and execute one or more blocks of code. They can fall through to the next case statement or break out. Default labels should be used and are similar to else statements in an if statement.