Java Text Blocks
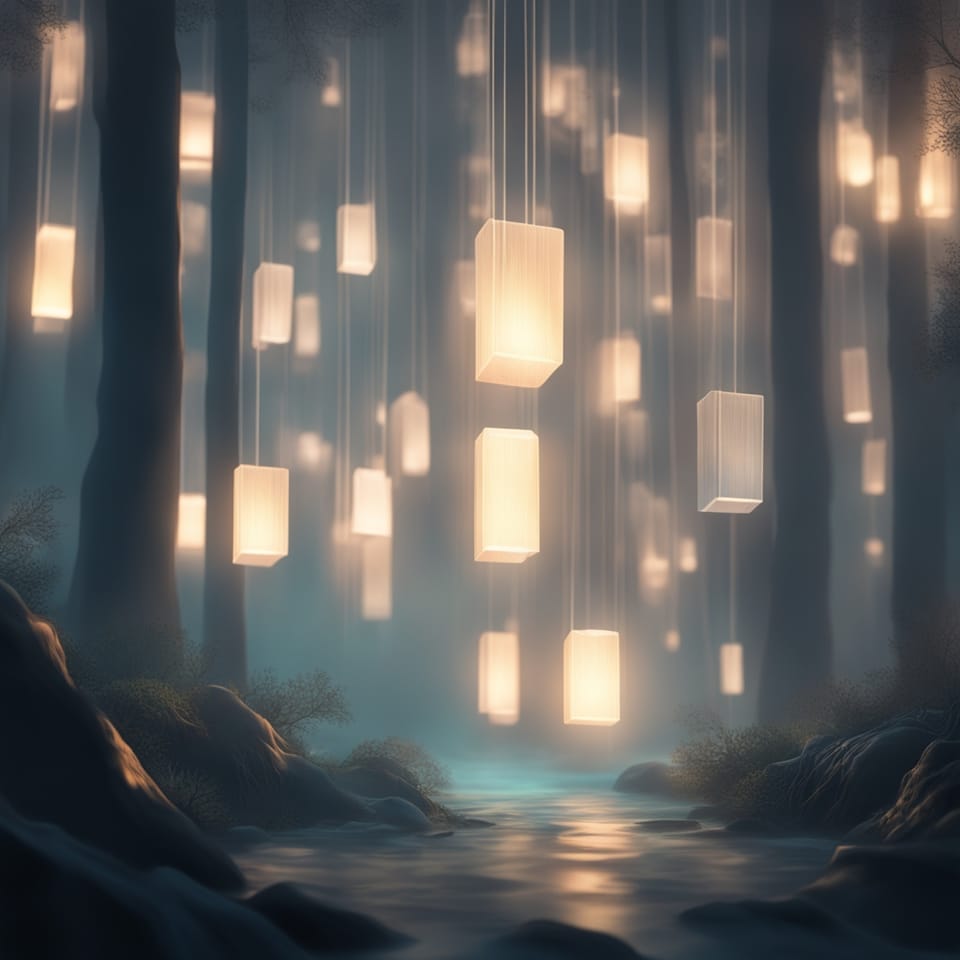
Text blocks
Java text blocks were introduced in Java 15. Text blocks are another way of defining a String value and can be used anywhere a String can be used. Since text blocks are just Strings, all String methods are available for text blocks.
Text blocks are defined starting with three double-quote characters, a new line, and ending with three double-quote characters. At the end of each line, excluding the first line, they will have a new line character. Double quotes inside a text block do not have to be escaped, but three double-quotes do.
An example of a text block is the following:
final var html = """
<html>
<head></head>
<body>
</body>
</html>""";
This will result in the following value.
<html>
<head></head>
<body>
</body>
</html>
The previous text block is equivalent to the following:
final var html =
"<html>\n" +
" <head></head>\n" +
" <body>\n" +
" </body>\n" +
"</html>";
Text blocks can't be a single line. After the opening three double-quote characters, a new line must follow. If it doesn't, this will result in a compiler error.
// This is invalid and won't compile
final var invalid = """Hello World""";
// This is valid
final var valid = """
Hello World""";
Indentation
Where the indentation of the value in the text block starts determines where the indentation starts. This includes the ending three double-quotes.
final var value = """
Hello
World
""";
This will result in the example below. The spaces have been replaced with periods.
Hello
....World
Moving the ending three double-quotes like the following:
final var value = """
Hello
World
"""
This will result in the example below. The spaces have been replaced with periods.
........Hello
....World
You can also use the indent() method to provide how many characters each line will be indented. This method takes an int of how many spaces to indent each line.
final var value = """
Hello
World"""
.indent(4);
This will result in the example below. The spaces have been replaced with periods.
....Hello
....World
Line Separator
The newline character used in text blocks is defined by the OS (Operating System). You can get the newline character with the following call.
final String newLine = System.lineSeparator();
If you want to remove new lines in text blocks, you can use \ to remove it.
final var greeting = """
How \
are \
you?""";
This will result in the following value.
How are you?
Spaces
The Java compiler will strip out all whitespace on the left and trailing whitespace on the right. To show what whitespace will be removed, the following example shows those characters as periods.
final var html = """
....<html>............
.... <head></head>.
.... <body>........
.... </body>.......
....</html>...........""";
Spaces can be added using \s where they would be stripped out.
If you want to add trailing spaces to each line, you can use | at the end of the line.
final var html = """
<html>............|
<head></head>.|
<body>........|
</body>.......|
</html>...........|""";
This will result in the following value showing spaces as periods.
<html>............
<head></head>.
<body>........
</body>.......
</html>...........
Conclusion
Java text blocks are a great way to simplify multi-line Strings. They reduce the syntax needed by not having to concatenate multiple strings together.