Java try-with-resources Statement
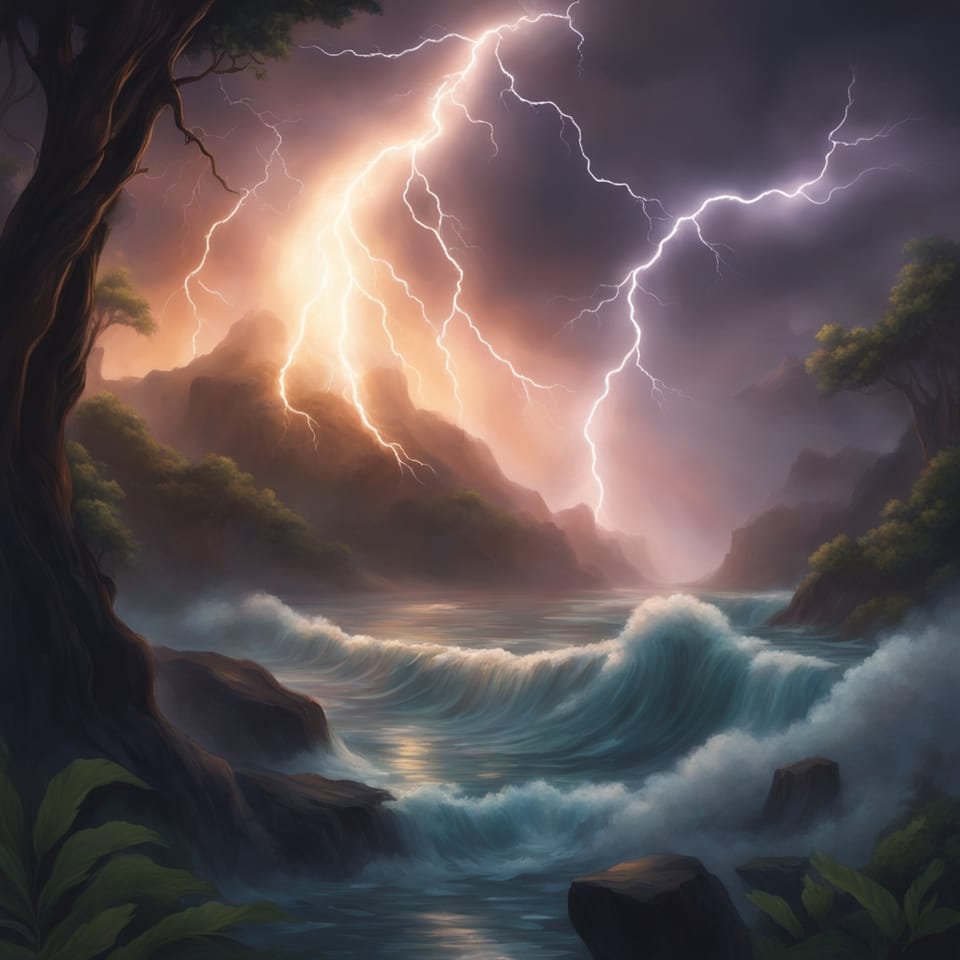
What is try-with-resources?
Introduced in Java 7, the try-with-resources statement is a try block that automatically handles the closing of one or more resources. When working with resources that need to be closed, you would typically write code like the following:
Connection connection;
try {
connection = openConnection();
// ...
} finally {
if (connection != null) {
connection.close();
}
}
This is where try-with-resources come in.
Using try-with-resources
The previous example can be simplified using try-with-resources with the following:
try (DatabaseConnection connection = openConnection()) {
// ...
}
Try-with-resources do not require a catch or finally block, like try blocks do.
Resources are closed after the try block executes. After the resources declared have been closed, then any catch or finally block is run.
You can also declare a variable outside of the try-with-resources block.
final var resource = new Resource();
try (resource) {
// ...
}
Working With Multiple Resources
Try-with-resources supports more than one resource, so you don't have to declare a new try block for each resource you have. Each resource, excluding the last one, must have a semicolon at the end.
try (Resource a = openResource("test1");
Resource b = openResource("test2");
Resource c = openResource("test3")) {
// ...
}
Resources will be declared and assigned in the order listed and will be closed in the reverse order they are listed.
Null Resources
Resources can be null. A null check is automatically performed before a resource is closed so you don't have to worry about a NullPointerException being thrown.
try (Resource a = openResource("test1");
Resource b = isAuthenticated ? openResource("test2") : null) {
System.out.println("Inside");
}
System.out.println("Outside");
This will print the following:
Inside
Outside
The AutoCloseable Interface
Classes that can use try-with-resources implement the java.lang.AutoCloseable interface. If you want your own classes to take advantage of try-with-resources, or are curious if other classes can be used, they need to implement this interface. AutoCloseable has one method on it: the close() method.
Conclusion
Java's try-with-resources is a try block that handles the closing of one or more resources. Implement the ability to use try-with-resources in your own classes by implementing the AutoCloseable interface. Try-with-resources makes closing resources safer, as it is done by Java instead of the compiler.