Java While Loop
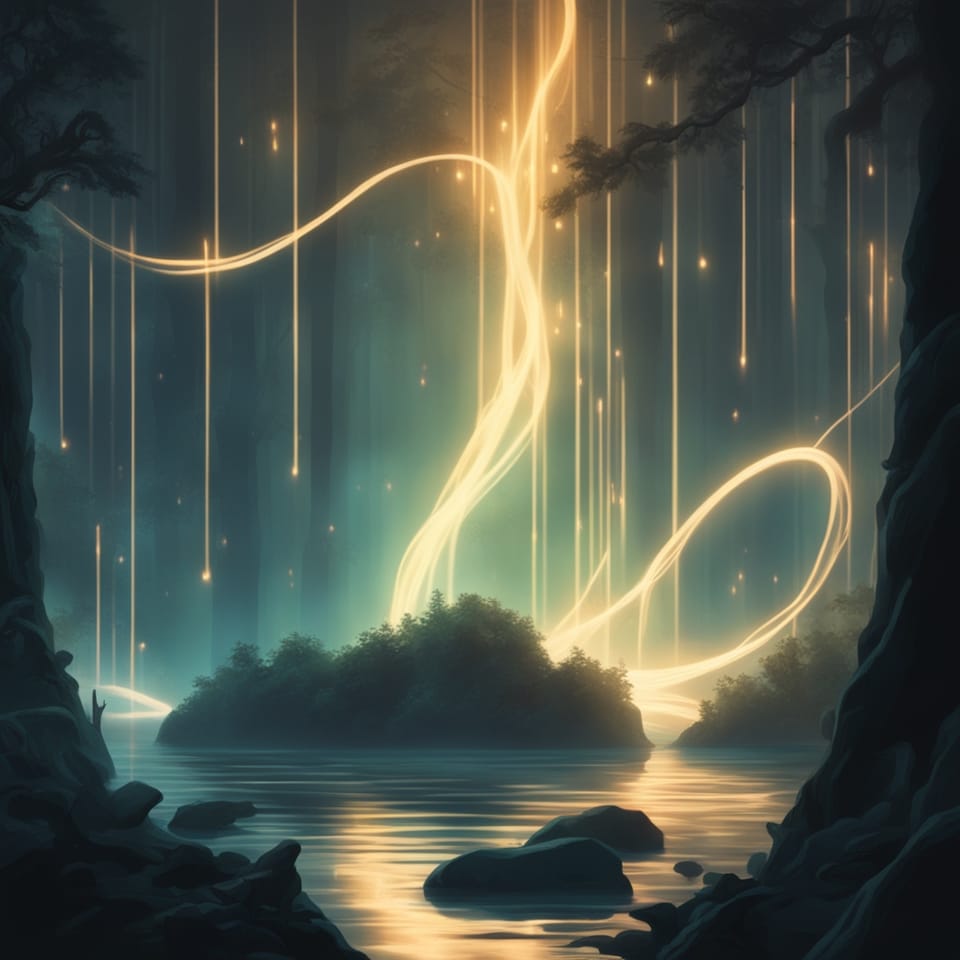
What Is a While Loop?
While loops allow you to execute a block of code while a certain condition is true. Once the condition is false, the loop will terminate.
While loops only have a condition. Any variables used in the condition need to be updated inside the while loop. This way you avoid having an infinite loop.
Syntax
A simple while loop can be written with the following:
var index = 0;
while (index != 5) {
System.out.println("index = " + index);
index++;
}
The while loop first checks if the condition is true before executing an iteration of the loop. If the condition is true, it will execute the block inside of the loop. After the block is executed, it then repeats this process. The previous example will produce the following output:
index = 0
index = 1
index = 2
index = 3
index = 4
While loops are best used when working with an unknown number of iterations. The following example shows a while loop used this way.
while (mugContainsCoffee()) {
sipCoffee();
}
Conclusion
While loops are a simple way to execute a block of code while a condition is met. Always remember to update the state that effects the while loop condition so you don't introduce an infinite loop.