Javadoc Linking Using @see, @link, and @value
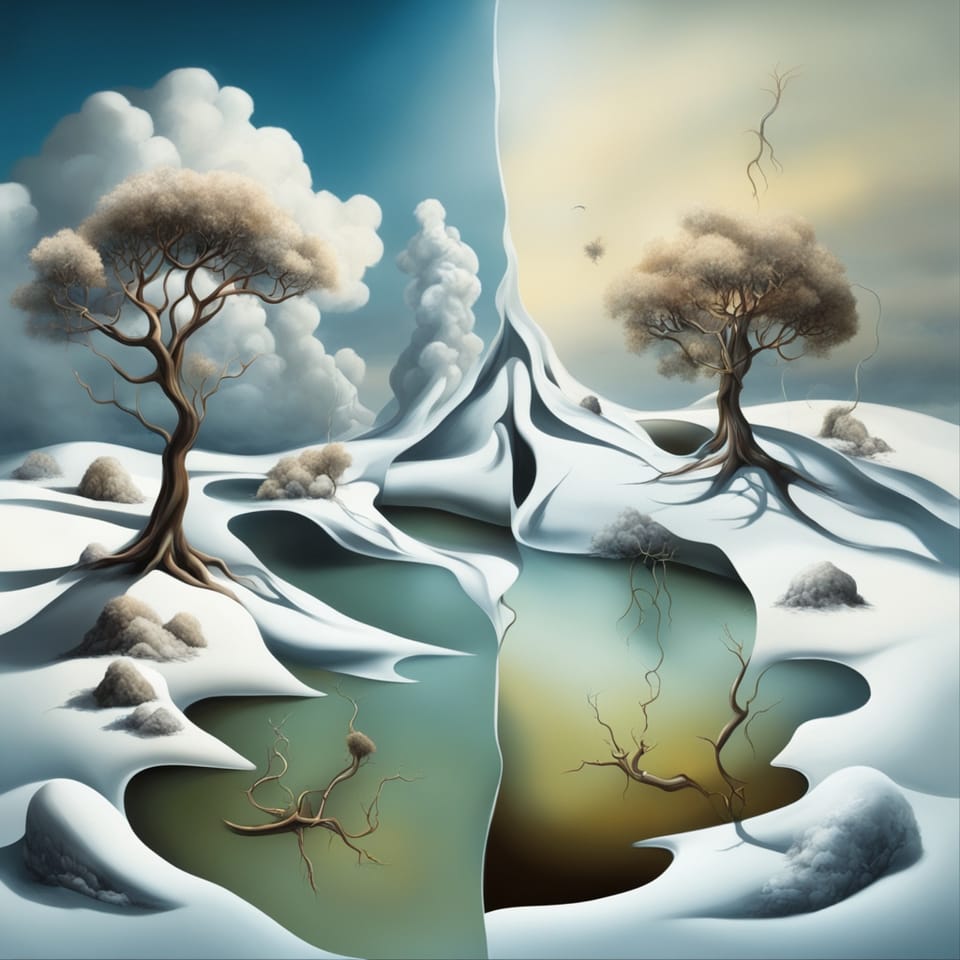
@see, @link, and @value Tags
Both the @see tag and @link tag are used the same in terms of linking. The difference between these two tags is that an @see tag is a block tag, and an @link tag is an inline tag. The @value tag uses the same syntax for linking but is specific for constant values and is an inline tag.
The code used for this article is the following class file:
package press.bytesize.domain;
public class User {
public static final String ADMIN_ID = "admin";
private String name;
public String fullName(final String suffix) {
// ...
}
}
Packages
To link to a package, you simply reference the package name.
/**
* Main descrption... {@link bytesize.press.domain}
*
* @see bytesize.press.domain
*/
Classes and Interfaces
Linking to a class or interface can be done using the FQN (Fully Qualified Name).
/**
* Main descrption... {@link bytesize.press.domain.User}
*
* @see bytesize.press.domain.User
*/
If the class or interface is imported into the class, you can use just the class or interface name.
import bytesize.press.domain.User;
/**
* Main descrption... {@link User}
*
* @see User
*/
public class MyClass {
// ...
}
Methods, Fields, and Constants
To link to a method, field, or constant is similar, but you use hashtag syntax. To link to the name field above, you use the following:
/**
* Main descrption... {@link User#name}
*
* @see User#name
*/
To link to the ADMIN_ID constant above, you use the same syntax.
/**
* Main descrption... {@link User#ADMIN_ID}
*
* @see User#ADMIN_ID
*/
To link to a method, you use similar syntax but with the parameter types included.
/**
* Main descrption...{@link User#fullName(String)}
*
* @see User#fullName(String)
*/
Constant Values
You can place a value of a constant in a Javadoc comment using the @value tag. You reference the constant the same way you would if you were linking using @see or @link tags. The only difference is that, you use the @value tag instead.
/**
* Admin user ID is always {@value User#ADMIN_ID}
*/
public void method() {
// ...
}
This will result in the following description:
Admin user ID is always "admin"
Conclusion
Linking is done using a FQN. If the class or interface is imported, you can use the class or interface name. Methods, fields, and constants are references using hash tag syntax.