Java's Number Class
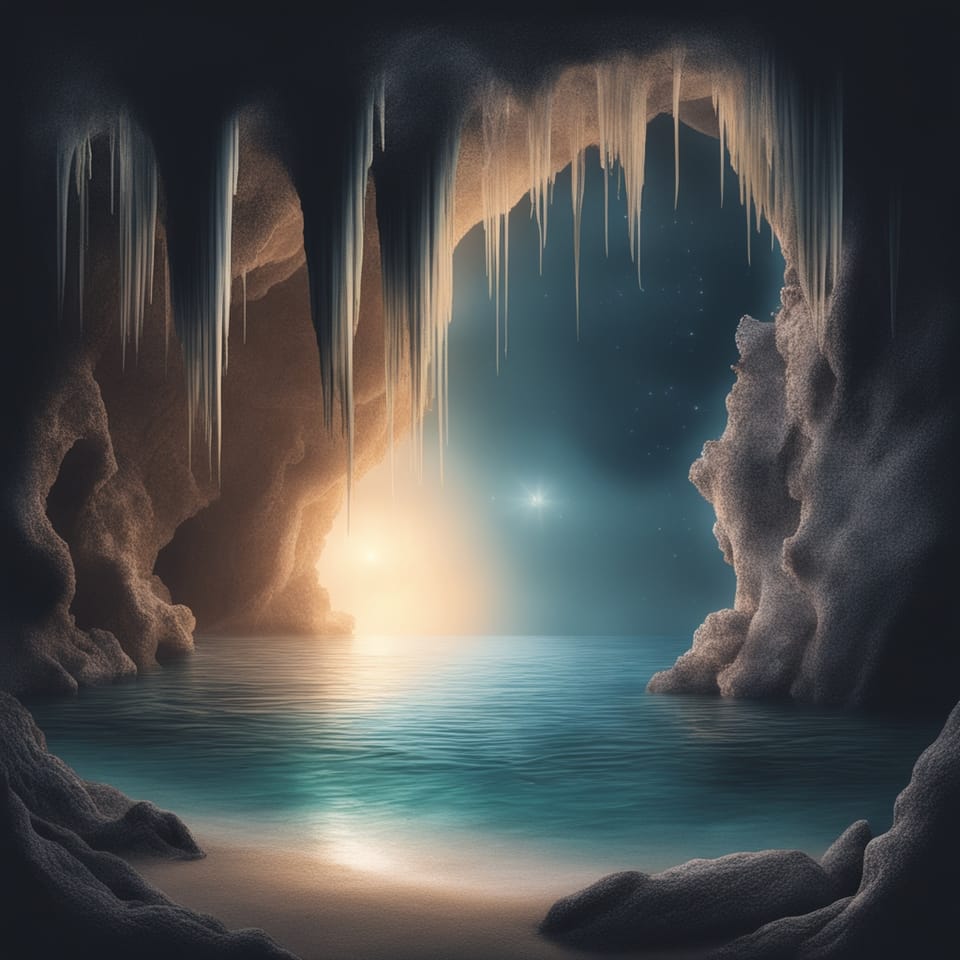
The Number Class
The Number class is the superclass for numeric classes and has methods to convert to primitive number types. BigInteger, Long, Short, Double, AtomicLong, and several other classes implement this class. The Number class contains the following methods for converting to primitive types:
- byteValue(): Converts to a primitive byte.
- shortValue(): Converts to a primitive short.
- intValue(): Converts to a primitive int.
- longValue(): Converts to a primitive long.
- floatValue(): Converts to a primitive float.
- doubleValue(): Converts to a primitive double.
When going from a smaller number to a larger number, widening primitive conversion may be used. Going from a larger number to a smaller number, narrowing primitive conversion may be used. Because of this, you can have loss of value, so use caution.
When to Use Number
One use case the Number class is nice for is if you are working with multiple databases. When querying a numeric column, working with one database might return a BigInteger and another might return a Long, even though the max value would be the max value of a long. You can cast the result to a Number and then use one of the methods to convert it to the primitive type you are wanting.
final long id = ((Number) row[0]).longValue();
You should only do this when you know that the type you are converting to is big enough to hold the number. If it isn't, an exception won't be thrown. Instead, you may have loss of value or precision, and the number will not be what you are expecting.
Conclusion
The Number class is an abstract class. It is the superclass of Number in Java for converting to primitive number types.