Label Statements in Java
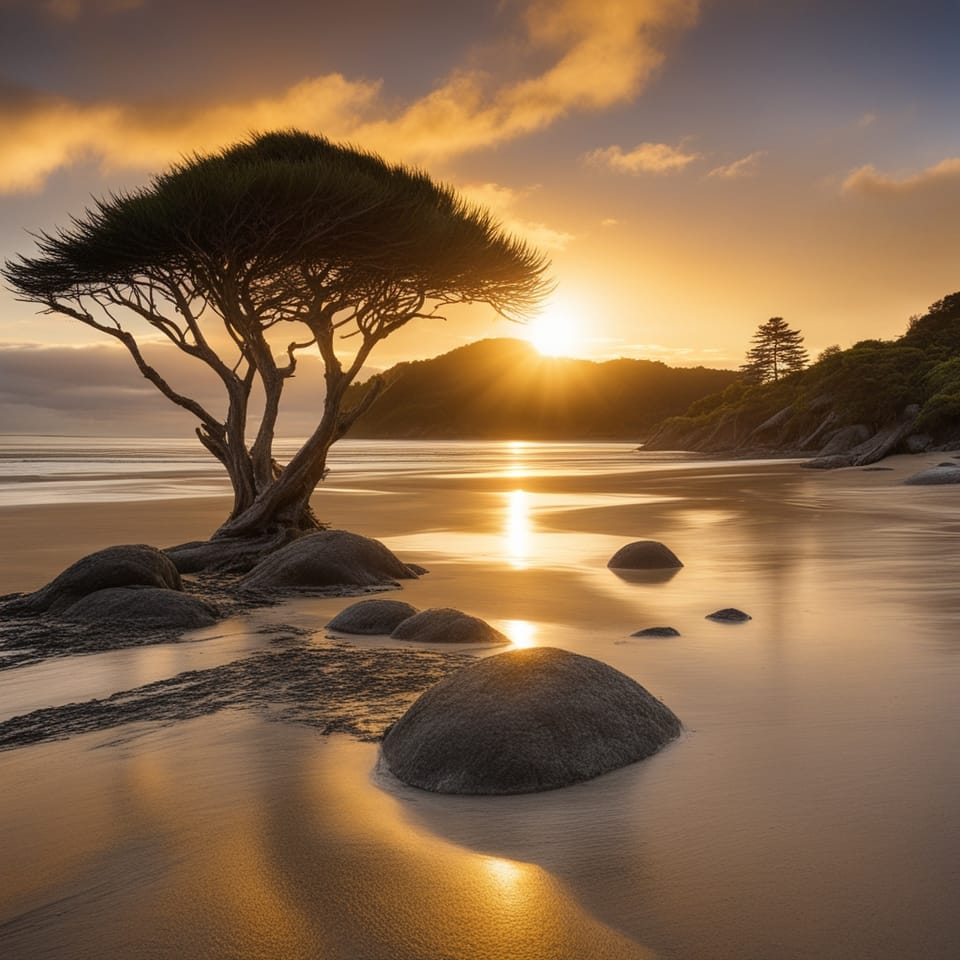
Label Statements
When working with loops, continue and break statements pertain to the current loop you are in. When you have an inner loop and an outer loop, how do you control the outer loop from within the inner loop? This is where label statements come in.
A label statement allows you to give a name to a loop. Label statements allow you to use break or continue statements based on the label that was given to a loop and allow you to control an outer loop from inside of an inner loop. Label statements can be used with any type of loop.
Using Label Statements
The syntax for a label statement using a while loop looks like the following:
myLoop:
while (true) {
// ...
}
This isn't useful by itself and is only showing the syntax of a label statement. To use a label statement and a continue statement on the outer loop inside an inner loop, you can use the following:
outerLoop:
for (int i = 0; i < 5; i++) {
innerLoop:
for (int j = 0; j < 5; j++) {
if (i == j) {
continue outerLoop;
}
}
}
When i equals j instead of the inner loop, it will execute a continue statement on the outer loop. This causes the inner loop to stop executing anymore iterations of the loop and go to the next iteration in the outer loop.
You can also use this with a break statement. The syntax is the same but instead uses the break keyword.
outerLoop:
for (int i = 0; i < 5; i++) {
innerLoop:
for (int j = 0; j < 5; j++) {
if (i == j) {
break outerLoop;
}
}
}
When i equals j, this will break out of the outer loop, and the inner and outer loops will not execute anymore iterations.
If you try to use a label statement that doesn't exist, it will result in a compiler error.
outerLoop:
for (int i = 0; i < 5; i++) {
innerLoop:
for (int j = 0; j < 5; j++) {
if (i == j) {
// Compiler error:
// undefined label: anotherOuterLoop
break anotherOuterLoop;
}
}
}
Conclusion
Label statements, while they have a rare place, should be avoided as much as possible and are considered a bad practice. They can cause code complexity and code duplication. Instead of using a label statement, rethink your logic and how you can simplify it.