Label Statements in Swift
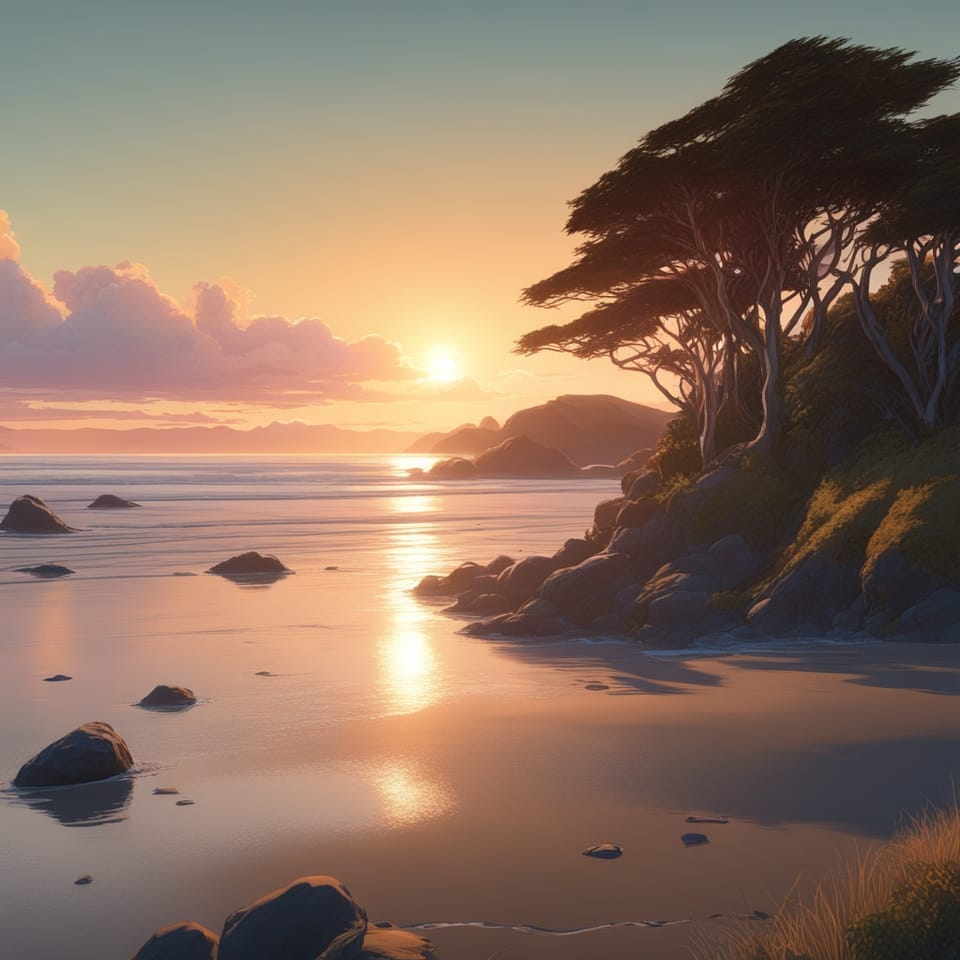
Label Statements
When you are inside of a loop and need to use a break or continue, it applies to the current loop you are in. Label statements are a way to label a loop and allow you to have control over any of the outer loops while inside of an inner loop. This allows you to execute a break or a continue on any of the outer loops inside of an inner loop, and they can be used with any type of loop.
Using Label Statements
To label a loop, you can do so with the following:
myLoop: while true {
// ...
}
Using it with a single loop doesn't buy you anything since they are used to control the outer loop inside of an inner loop. To control the outer loop inside of an inner loop using a continue statement, you could write the following:
firstLoop: for x in 0...5 {
secondLoop: for y in 0...5 {
if x == y {
continue firstLoop
}
// ...
}
}
When x equals y, it will stop the execution of the inner loop labeled secondLoop and skip to the next iteration of the loop labeled firstLoop.
You can also use a break statement instead of a continue statement with label statements. This allows you to break out of an outer loop inside of an inner loop.
firstLoop: for x in 0...5 {
secondLoop: for y in 0...5 {
if x == 5 {
break firstLoop
}
// ...
}
}
When x equals 5, it will stop the execution of the loop labeled secondLoop and break out of the loop labeled firstLoop.
When you are using label statements, if you try to use a label that doesn't exist, it will result in a compiler error. For example, if you mistype the label and add an s at the end of it, it would result in the following compiler error.
firstLoop: for x in 0...5 {
secondLoop: for y in 0...5 {
if x == 5 {
// Compiler error:
// Cannot find label 'secondLoops' in scope,
// did you mean 'secondLoop'
break firstLoops
}
// ...
}
}
Conclusion
Since break and continue statements pertain to the loop you are in, labeled statements allow you to control the outer loop inside the inner loop. Using a label statement, you can execute a break or continue statement on any of the outer loops.